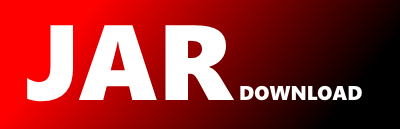
com.symphony.api.model.V1DLPPolicyRequest Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* The policy object to use for creating/updating a policy.
**/
@Schema(description="The policy object to use for creating/updating a policy.")
public class V1DLPPolicyRequest {
@Schema(required = true, description = "The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified. ")
/**
* The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified.
**/
private List contentTypes = new ArrayList();
@Schema(description = "List of dictionaries Ids for the policy.")
/**
* List of dictionaries Ids for the policy.
**/
private List dictionaryIds = null;
@Schema(required = true, description = "Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.")
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
**/
private String name = null;
@Schema(required = true, description = "List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"]. ")
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
**/
private List scopes = new ArrayList();
@Schema(required = true, description = "Type of policy. Possible values \"Block\" or \"Warn\".")
/**
* Type of policy. Possible values \"Block\" or \"Warn\".
**/
private String type = null;
/**
* The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified.
* @return contentTypes
**/
@JsonProperty("contentTypes")
public List getContentTypes() {
return contentTypes;
}
public void setContentTypes(List contentTypes) {
this.contentTypes = contentTypes;
}
public V1DLPPolicyRequest contentTypes(List contentTypes) {
this.contentTypes = contentTypes;
return this;
}
public V1DLPPolicyRequest addContentTypesItem(String contentTypesItem) {
this.contentTypes.add(contentTypesItem);
return this;
}
/**
* List of dictionaries Ids for the policy.
* @return dictionaryIds
**/
@JsonProperty("dictionaryIds")
public List getDictionaryIds() {
return dictionaryIds;
}
public void setDictionaryIds(List dictionaryIds) {
this.dictionaryIds = dictionaryIds;
}
public V1DLPPolicyRequest dictionaryIds(List dictionaryIds) {
this.dictionaryIds = dictionaryIds;
return this;
}
public V1DLPPolicyRequest addDictionaryIdsItem(String dictionaryIdsItem) {
this.dictionaryIds.add(dictionaryIdsItem);
return this;
}
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public V1DLPPolicyRequest name(String name) {
this.name = name;
return this;
}
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
* @return scopes
**/
@JsonProperty("scopes")
public List getScopes() {
return scopes;
}
public void setScopes(List scopes) {
this.scopes = scopes;
}
public V1DLPPolicyRequest scopes(List scopes) {
this.scopes = scopes;
return this;
}
public V1DLPPolicyRequest addScopesItem(String scopesItem) {
this.scopes.add(scopesItem);
return this;
}
/**
* Type of policy. Possible values \"Block\" or \"Warn\".
* @return type
**/
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V1DLPPolicyRequest type(String type) {
this.type = type;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1DLPPolicyRequest {\n");
sb.append(" contentTypes: ").append(toIndentedString(contentTypes)).append("\n");
sb.append(" dictionaryIds: ").append(toIndentedString(dictionaryIds)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" scopes: ").append(toIndentedString(scopes)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy