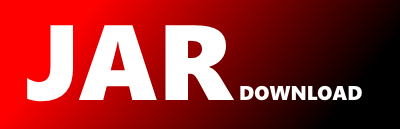
com.symphony.api.model.V1DLPViolationSignalResponse Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V1DLPViolationSignal;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V1DLPViolationSignalResponse {
@Schema(description = "A representation of list of violations due to signal creation/update sent by a user of Symphony")
/**
* A representation of list of violations due to signal creation/update sent by a user of Symphony
**/
private List violations = null;
@Schema(description = "Offset for the next chunk of violations to be submitted in the next request. Value is null if there are no further violations.")
/**
* Offset for the next chunk of violations to be submitted in the next request. Value is null if there are no further violations.
**/
private String nextOffset = null;
/**
* A representation of list of violations due to signal creation/update sent by a user of Symphony
* @return violations
**/
@JsonProperty("violations")
public List getViolations() {
return violations;
}
public void setViolations(List violations) {
this.violations = violations;
}
public V1DLPViolationSignalResponse violations(List violations) {
this.violations = violations;
return this;
}
public V1DLPViolationSignalResponse addViolationsItem(V1DLPViolationSignal violationsItem) {
this.violations.add(violationsItem);
return this;
}
/**
* Offset for the next chunk of violations to be submitted in the next request. Value is null if there are no further violations.
* @return nextOffset
**/
@JsonProperty("nextOffset")
public String getNextOffset() {
return nextOffset;
}
public void setNextOffset(String nextOffset) {
this.nextOffset = nextOffset;
}
public V1DLPViolationSignalResponse nextOffset(String nextOffset) {
this.nextOffset = nextOffset;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1DLPViolationSignalResponse {\n");
sb.append(" violations: ").append(toIndentedString(violations)).append("\n");
sb.append(" nextOffset: ").append(toIndentedString(nextOffset)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy