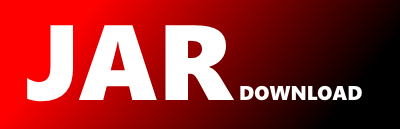
com.symphony.api.model.V1HealthCheckResponse Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V1HealthCheckResponse {
@Schema(description = "Indicates whether the Agent server can connect to the Pod")
/**
* Indicates whether the Agent server can connect to the Pod
**/
private Boolean podConnectivity = null;
@Schema(description = "Error details in case of no Pod connectivity")
/**
* Error details in case of no Pod connectivity
**/
private String podConnectivityError = null;
@Schema(description = "Indicates whether the Agent server can connect to the Key Manager")
/**
* Indicates whether the Agent server can connect to the Key Manager
**/
private Boolean keyManagerConnectivity = null;
@Schema(description = "Error details in case of no Key Manager connectivity")
/**
* Error details in case of no Key Manager connectivity
**/
private String keyManagerConnectivityError = null;
@Schema(description = "The version number of the Agent server")
/**
* The version number of the Agent server
**/
private String version = null;
/**
* Indicates whether the Agent server can connect to the Pod
* @return podConnectivity
**/
@JsonProperty("podConnectivity")
public Boolean isPodConnectivity() {
return podConnectivity;
}
public void setPodConnectivity(Boolean podConnectivity) {
this.podConnectivity = podConnectivity;
}
public V1HealthCheckResponse podConnectivity(Boolean podConnectivity) {
this.podConnectivity = podConnectivity;
return this;
}
/**
* Error details in case of no Pod connectivity
* @return podConnectivityError
**/
@JsonProperty("podConnectivityError")
public String getPodConnectivityError() {
return podConnectivityError;
}
public void setPodConnectivityError(String podConnectivityError) {
this.podConnectivityError = podConnectivityError;
}
public V1HealthCheckResponse podConnectivityError(String podConnectivityError) {
this.podConnectivityError = podConnectivityError;
return this;
}
/**
* Indicates whether the Agent server can connect to the Key Manager
* @return keyManagerConnectivity
**/
@JsonProperty("keyManagerConnectivity")
public Boolean isKeyManagerConnectivity() {
return keyManagerConnectivity;
}
public void setKeyManagerConnectivity(Boolean keyManagerConnectivity) {
this.keyManagerConnectivity = keyManagerConnectivity;
}
public V1HealthCheckResponse keyManagerConnectivity(Boolean keyManagerConnectivity) {
this.keyManagerConnectivity = keyManagerConnectivity;
return this;
}
/**
* Error details in case of no Key Manager connectivity
* @return keyManagerConnectivityError
**/
@JsonProperty("keyManagerConnectivityError")
public String getKeyManagerConnectivityError() {
return keyManagerConnectivityError;
}
public void setKeyManagerConnectivityError(String keyManagerConnectivityError) {
this.keyManagerConnectivityError = keyManagerConnectivityError;
}
public V1HealthCheckResponse keyManagerConnectivityError(String keyManagerConnectivityError) {
this.keyManagerConnectivityError = keyManagerConnectivityError;
return this;
}
/**
* The version number of the Agent server
* @return version
**/
@JsonProperty("version")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public V1HealthCheckResponse version(String version) {
this.version = version;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1HealthCheckResponse {\n");
sb.append(" podConnectivity: ").append(toIndentedString(podConnectivity)).append("\n");
sb.append(" podConnectivityError: ").append(toIndentedString(podConnectivityError)).append("\n");
sb.append(" keyManagerConnectivity: ").append(toIndentedString(keyManagerConnectivity)).append("\n");
sb.append(" keyManagerConnectivityError: ").append(toIndentedString(keyManagerConnectivityError)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy