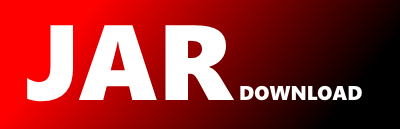
com.symphony.api.model.V2AdminStreamInfo Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V2AdminStreamAttributes;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Stream information
**/
@Schema(description="Stream information")
public class V2AdminStreamInfo {
@Schema(description = "stream id")
/**
* stream id
**/
private String id = null;
@Schema(description = "true indicate this stream has the scope of external and false indictate this stream has the scope of internal. Deprecated, use origin")
/**
* true indicate this stream has the scope of external and false indictate this stream has the scope of internal. Deprecated, use origin
**/
private Boolean isExternal = null;
@Schema(description = "true indicate that this stream has the status of active and false indicate this stream has the scope of inactive")
/**
* true indicate that this stream has the status of active and false indicate this stream has the scope of inactive
**/
private Boolean isActive = null;
@Schema(description = "true indicate that this stream has a privacy setting of public. This only apply a ROOM stream type.")
/**
* true indicate that this stream has a privacy setting of public. This only apply a ROOM stream type.
**/
private Boolean isPublic = null;
@Schema(description = "type of stream (IM, MIM, ROOM)")
/**
* type of stream (IM, MIM, ROOM)
**/
private String type = null;
@Schema(description = "If true, this is a cross-pod stream.")
/**
* If true, this is a cross-pod stream.
**/
private Boolean crossPod = null;
@Schema(description = "INTERNAL if the creator of this stream belongs to the pod, EXTERNAL otherwise")
/**
* INTERNAL if the creator of this stream belongs to the pod, EXTERNAL otherwise
**/
private String origin = null;
@Schema(description = "")
private V2AdminStreamAttributes attributes = null;
/**
* stream id
* @return id
**/
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public V2AdminStreamInfo id(String id) {
this.id = id;
return this;
}
/**
* true indicate this stream has the scope of external and false indictate this stream has the scope of internal. Deprecated, use origin
* @return isExternal
**/
@JsonProperty("isExternal")
public Boolean isIsExternal() {
return isExternal;
}
public void setIsExternal(Boolean isExternal) {
this.isExternal = isExternal;
}
public V2AdminStreamInfo isExternal(Boolean isExternal) {
this.isExternal = isExternal;
return this;
}
/**
* true indicate that this stream has the status of active and false indicate this stream has the scope of inactive
* @return isActive
**/
@JsonProperty("isActive")
public Boolean isIsActive() {
return isActive;
}
public void setIsActive(Boolean isActive) {
this.isActive = isActive;
}
public V2AdminStreamInfo isActive(Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* true indicate that this stream has a privacy setting of public. This only apply a ROOM stream type.
* @return isPublic
**/
@JsonProperty("isPublic")
public Boolean isIsPublic() {
return isPublic;
}
public void setIsPublic(Boolean isPublic) {
this.isPublic = isPublic;
}
public V2AdminStreamInfo isPublic(Boolean isPublic) {
this.isPublic = isPublic;
return this;
}
/**
* type of stream (IM, MIM, ROOM)
* @return type
**/
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V2AdminStreamInfo type(String type) {
this.type = type;
return this;
}
/**
* If true, this is a cross-pod stream.
* @return crossPod
**/
@JsonProperty("crossPod")
public Boolean isCrossPod() {
return crossPod;
}
public void setCrossPod(Boolean crossPod) {
this.crossPod = crossPod;
}
public V2AdminStreamInfo crossPod(Boolean crossPod) {
this.crossPod = crossPod;
return this;
}
/**
* INTERNAL if the creator of this stream belongs to the pod, EXTERNAL otherwise
* @return origin
**/
@JsonProperty("origin")
public String getOrigin() {
return origin;
}
public void setOrigin(String origin) {
this.origin = origin;
}
public V2AdminStreamInfo origin(String origin) {
this.origin = origin;
return this;
}
/**
* Get attributes
* @return attributes
**/
@JsonProperty("attributes")
public V2AdminStreamAttributes getAttributes() {
return attributes;
}
public void setAttributes(V2AdminStreamAttributes attributes) {
this.attributes = attributes;
}
public V2AdminStreamInfo attributes(V2AdminStreamAttributes attributes) {
this.attributes = attributes;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V2AdminStreamInfo {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" isExternal: ").append(toIndentedString(isExternal)).append("\n");
sb.append(" isActive: ").append(toIndentedString(isActive)).append("\n");
sb.append(" isPublic: ").append(toIndentedString(isPublic)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" crossPod: ").append(toIndentedString(crossPod)).append("\n");
sb.append(" origin: ").append(toIndentedString(origin)).append("\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy