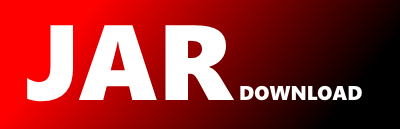
com.symphony.api.model.V2UserAttributes Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V2UserKeyRequest;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* V2 User record.
**/
@Schema(description="V2 User record.")
public class V2UserAttributes {
@Schema(description = "")
private String emailAddress = null;
@Schema(description = "")
private String firstName = null;
@Schema(description = "")
private String lastName = null;
@Schema(description = "")
private String userName = null;
@Schema(description = "")
private String displayName = null;
@Schema(description = "")
private String companyName = null;
@Schema(description = "")
private String department = null;
@Schema(description = "")
private String division = null;
@Schema(description = "")
private String title = null;
@Schema(description = "")
private String workPhoneNumber = null;
@Schema(description = "")
private String mobilePhoneNumber = null;
@Schema(description = "")
private String twoFactorAuthPhone = null;
@Schema(description = "")
private String smsNumber = null;
public enum AccountTypeEnum {
NORMAL("NORMAL"),
SYSTEM("SYSTEM"),
SDL("SDL");
private String value;
AccountTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static AccountTypeEnum fromValue(String text) {
for (AccountTypeEnum b : AccountTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "")
private AccountTypeEnum accountType = null;
@Schema(description = "")
private String location = null;
@Schema(description = "")
private String recommendedLanguage = null;
@Schema(description = "")
private String jobFunction = null;
@Schema(description = "")
private List assetClasses = null;
@Schema(description = "")
private List industries = null;
@Schema(description = "")
private List marketCoverage = null;
@Schema(description = "")
private List responsibility = null;
@Schema(description = "")
private List function = null;
@Schema(description = "")
private List instrument = null;
@Schema(description = "")
private V2UserKeyRequest currentKey = null;
@Schema(description = "")
private V2UserKeyRequest previousKey = null;
/**
* Get emailAddress
* @return emailAddress
**/
@JsonProperty("emailAddress")
public String getEmailAddress() {
return emailAddress;
}
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
public V2UserAttributes emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
/**
* Get firstName
* @return firstName
**/
@JsonProperty("firstName")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public V2UserAttributes firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Get lastName
* @return lastName
**/
@JsonProperty("lastName")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public V2UserAttributes lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Get userName
* @return userName
**/
@JsonProperty("userName")
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public V2UserAttributes userName(String userName) {
this.userName = userName;
return this;
}
/**
* Get displayName
* @return displayName
**/
@JsonProperty("displayName")
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public V2UserAttributes displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get companyName
* @return companyName
**/
@JsonProperty("companyName")
public String getCompanyName() {
return companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public V2UserAttributes companyName(String companyName) {
this.companyName = companyName;
return this;
}
/**
* Get department
* @return department
**/
@JsonProperty("department")
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
public V2UserAttributes department(String department) {
this.department = department;
return this;
}
/**
* Get division
* @return division
**/
@JsonProperty("division")
public String getDivision() {
return division;
}
public void setDivision(String division) {
this.division = division;
}
public V2UserAttributes division(String division) {
this.division = division;
return this;
}
/**
* Get title
* @return title
**/
@JsonProperty("title")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public V2UserAttributes title(String title) {
this.title = title;
return this;
}
/**
* Get workPhoneNumber
* @return workPhoneNumber
**/
@JsonProperty("workPhoneNumber")
public String getWorkPhoneNumber() {
return workPhoneNumber;
}
public void setWorkPhoneNumber(String workPhoneNumber) {
this.workPhoneNumber = workPhoneNumber;
}
public V2UserAttributes workPhoneNumber(String workPhoneNumber) {
this.workPhoneNumber = workPhoneNumber;
return this;
}
/**
* Get mobilePhoneNumber
* @return mobilePhoneNumber
**/
@JsonProperty("mobilePhoneNumber")
public String getMobilePhoneNumber() {
return mobilePhoneNumber;
}
public void setMobilePhoneNumber(String mobilePhoneNumber) {
this.mobilePhoneNumber = mobilePhoneNumber;
}
public V2UserAttributes mobilePhoneNumber(String mobilePhoneNumber) {
this.mobilePhoneNumber = mobilePhoneNumber;
return this;
}
/**
* Get twoFactorAuthPhone
* @return twoFactorAuthPhone
**/
@JsonProperty("twoFactorAuthPhone")
public String getTwoFactorAuthPhone() {
return twoFactorAuthPhone;
}
public void setTwoFactorAuthPhone(String twoFactorAuthPhone) {
this.twoFactorAuthPhone = twoFactorAuthPhone;
}
public V2UserAttributes twoFactorAuthPhone(String twoFactorAuthPhone) {
this.twoFactorAuthPhone = twoFactorAuthPhone;
return this;
}
/**
* Get smsNumber
* @return smsNumber
**/
@JsonProperty("smsNumber")
public String getSmsNumber() {
return smsNumber;
}
public void setSmsNumber(String smsNumber) {
this.smsNumber = smsNumber;
}
public V2UserAttributes smsNumber(String smsNumber) {
this.smsNumber = smsNumber;
return this;
}
/**
* Get accountType
* @return accountType
**/
@JsonProperty("accountType")
public String getAccountType() {
if (accountType == null) {
return null;
}
return accountType.getValue();
}
public void setAccountType(AccountTypeEnum accountType) {
this.accountType = accountType;
}
public V2UserAttributes accountType(AccountTypeEnum accountType) {
this.accountType = accountType;
return this;
}
/**
* Get location
* @return location
**/
@JsonProperty("location")
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public V2UserAttributes location(String location) {
this.location = location;
return this;
}
/**
* Get recommendedLanguage
* @return recommendedLanguage
**/
@JsonProperty("recommendedLanguage")
public String getRecommendedLanguage() {
return recommendedLanguage;
}
public void setRecommendedLanguage(String recommendedLanguage) {
this.recommendedLanguage = recommendedLanguage;
}
public V2UserAttributes recommendedLanguage(String recommendedLanguage) {
this.recommendedLanguage = recommendedLanguage;
return this;
}
/**
* Get jobFunction
* @return jobFunction
**/
@JsonProperty("jobFunction")
public String getJobFunction() {
return jobFunction;
}
public void setJobFunction(String jobFunction) {
this.jobFunction = jobFunction;
}
public V2UserAttributes jobFunction(String jobFunction) {
this.jobFunction = jobFunction;
return this;
}
/**
* Get assetClasses
* @return assetClasses
**/
@JsonProperty("assetClasses")
public List getAssetClasses() {
return assetClasses;
}
public void setAssetClasses(List assetClasses) {
this.assetClasses = assetClasses;
}
public V2UserAttributes assetClasses(List assetClasses) {
this.assetClasses = assetClasses;
return this;
}
public V2UserAttributes addAssetClassesItem(String assetClassesItem) {
this.assetClasses.add(assetClassesItem);
return this;
}
/**
* Get industries
* @return industries
**/
@JsonProperty("industries")
public List getIndustries() {
return industries;
}
public void setIndustries(List industries) {
this.industries = industries;
}
public V2UserAttributes industries(List industries) {
this.industries = industries;
return this;
}
public V2UserAttributes addIndustriesItem(String industriesItem) {
this.industries.add(industriesItem);
return this;
}
/**
* Get marketCoverage
* @return marketCoverage
**/
@JsonProperty("marketCoverage")
public List getMarketCoverage() {
return marketCoverage;
}
public void setMarketCoverage(List marketCoverage) {
this.marketCoverage = marketCoverage;
}
public V2UserAttributes marketCoverage(List marketCoverage) {
this.marketCoverage = marketCoverage;
return this;
}
public V2UserAttributes addMarketCoverageItem(String marketCoverageItem) {
this.marketCoverage.add(marketCoverageItem);
return this;
}
/**
* Get responsibility
* @return responsibility
**/
@JsonProperty("responsibility")
public List getResponsibility() {
return responsibility;
}
public void setResponsibility(List responsibility) {
this.responsibility = responsibility;
}
public V2UserAttributes responsibility(List responsibility) {
this.responsibility = responsibility;
return this;
}
public V2UserAttributes addResponsibilityItem(String responsibilityItem) {
this.responsibility.add(responsibilityItem);
return this;
}
/**
* Get function
* @return function
**/
@JsonProperty("function")
public List getFunction() {
return function;
}
public void setFunction(List function) {
this.function = function;
}
public V2UserAttributes function(List function) {
this.function = function;
return this;
}
public V2UserAttributes addFunctionItem(String functionItem) {
this.function.add(functionItem);
return this;
}
/**
* Get instrument
* @return instrument
**/
@JsonProperty("instrument")
public List getInstrument() {
return instrument;
}
public void setInstrument(List instrument) {
this.instrument = instrument;
}
public V2UserAttributes instrument(List instrument) {
this.instrument = instrument;
return this;
}
public V2UserAttributes addInstrumentItem(String instrumentItem) {
this.instrument.add(instrumentItem);
return this;
}
/**
* Get currentKey
* @return currentKey
**/
@JsonProperty("currentKey")
public V2UserKeyRequest getCurrentKey() {
return currentKey;
}
public void setCurrentKey(V2UserKeyRequest currentKey) {
this.currentKey = currentKey;
}
public V2UserAttributes currentKey(V2UserKeyRequest currentKey) {
this.currentKey = currentKey;
return this;
}
/**
* Get previousKey
* @return previousKey
**/
@JsonProperty("previousKey")
public V2UserKeyRequest getPreviousKey() {
return previousKey;
}
public void setPreviousKey(V2UserKeyRequest previousKey) {
this.previousKey = previousKey;
}
public V2UserAttributes previousKey(V2UserKeyRequest previousKey) {
this.previousKey = previousKey;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V2UserAttributes {\n");
sb.append(" emailAddress: ").append(toIndentedString(emailAddress)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" userName: ").append(toIndentedString(userName)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" companyName: ").append(toIndentedString(companyName)).append("\n");
sb.append(" department: ").append(toIndentedString(department)).append("\n");
sb.append(" division: ").append(toIndentedString(division)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" workPhoneNumber: ").append(toIndentedString(workPhoneNumber)).append("\n");
sb.append(" mobilePhoneNumber: ").append(toIndentedString(mobilePhoneNumber)).append("\n");
sb.append(" twoFactorAuthPhone: ").append(toIndentedString(twoFactorAuthPhone)).append("\n");
sb.append(" smsNumber: ").append(toIndentedString(smsNumber)).append("\n");
sb.append(" accountType: ").append(toIndentedString(accountType)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" recommendedLanguage: ").append(toIndentedString(recommendedLanguage)).append("\n");
sb.append(" jobFunction: ").append(toIndentedString(jobFunction)).append("\n");
sb.append(" assetClasses: ").append(toIndentedString(assetClasses)).append("\n");
sb.append(" industries: ").append(toIndentedString(industries)).append("\n");
sb.append(" marketCoverage: ").append(toIndentedString(marketCoverage)).append("\n");
sb.append(" responsibility: ").append(toIndentedString(responsibility)).append("\n");
sb.append(" function: ").append(toIndentedString(function)).append("\n");
sb.append(" instrument: ").append(toIndentedString(instrument)).append("\n");
sb.append(" currentKey: ").append(toIndentedString(currentKey)).append("\n");
sb.append(" previousKey: ").append(toIndentedString(previousKey)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy