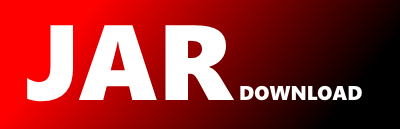
com.symphony.api.model.V2UserDetail Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.Avatar;
import com.symphony.api.model.IntegerList;
import com.symphony.api.model.StringList;
import com.symphony.api.model.UserSystemInfo;
import com.symphony.api.model.V2UserAttributes;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* V2 Detailed User record.
**/
@Schema(description="V2 Detailed User record.")
public class V2UserDetail {
@Schema(description = "")
private V2UserAttributes userAttributes = null;
@Schema(description = "")
private UserSystemInfo userSystemInfo = null;
@Schema(description = "")
private IntegerList features = null;
@Schema(description = "")
private IntegerList apps = null;
@Schema(description = "")
private IntegerList groups = null;
@Schema(description = "")
private StringList roles = null;
@Schema(description = "")
private IntegerList disclaimers = null;
@Schema(description = "")
private Avatar avatar = null;
/**
* Get userAttributes
* @return userAttributes
**/
@JsonProperty("userAttributes")
public V2UserAttributes getUserAttributes() {
return userAttributes;
}
public void setUserAttributes(V2UserAttributes userAttributes) {
this.userAttributes = userAttributes;
}
public V2UserDetail userAttributes(V2UserAttributes userAttributes) {
this.userAttributes = userAttributes;
return this;
}
/**
* Get userSystemInfo
* @return userSystemInfo
**/
@JsonProperty("userSystemInfo")
public UserSystemInfo getUserSystemInfo() {
return userSystemInfo;
}
public void setUserSystemInfo(UserSystemInfo userSystemInfo) {
this.userSystemInfo = userSystemInfo;
}
public V2UserDetail userSystemInfo(UserSystemInfo userSystemInfo) {
this.userSystemInfo = userSystemInfo;
return this;
}
/**
* Get features
* @return features
**/
@JsonProperty("features")
public IntegerList getFeatures() {
return features;
}
public void setFeatures(IntegerList features) {
this.features = features;
}
public V2UserDetail features(IntegerList features) {
this.features = features;
return this;
}
/**
* Get apps
* @return apps
**/
@JsonProperty("apps")
public IntegerList getApps() {
return apps;
}
public void setApps(IntegerList apps) {
this.apps = apps;
}
public V2UserDetail apps(IntegerList apps) {
this.apps = apps;
return this;
}
/**
* Get groups
* @return groups
**/
@JsonProperty("groups")
public IntegerList getGroups() {
return groups;
}
public void setGroups(IntegerList groups) {
this.groups = groups;
}
public V2UserDetail groups(IntegerList groups) {
this.groups = groups;
return this;
}
/**
* Get roles
* @return roles
**/
@JsonProperty("roles")
public StringList getRoles() {
return roles;
}
public void setRoles(StringList roles) {
this.roles = roles;
}
public V2UserDetail roles(StringList roles) {
this.roles = roles;
return this;
}
/**
* Get disclaimers
* @return disclaimers
**/
@JsonProperty("disclaimers")
public IntegerList getDisclaimers() {
return disclaimers;
}
public void setDisclaimers(IntegerList disclaimers) {
this.disclaimers = disclaimers;
}
public V2UserDetail disclaimers(IntegerList disclaimers) {
this.disclaimers = disclaimers;
return this;
}
/**
* Get avatar
* @return avatar
**/
@JsonProperty("avatar")
public Avatar getAvatar() {
return avatar;
}
public void setAvatar(Avatar avatar) {
this.avatar = avatar;
}
public V2UserDetail avatar(Avatar avatar) {
this.avatar = avatar;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V2UserDetail {\n");
sb.append(" userAttributes: ").append(toIndentedString(userAttributes)).append("\n");
sb.append(" userSystemInfo: ").append(toIndentedString(userSystemInfo)).append("\n");
sb.append(" features: ").append(toIndentedString(features)).append("\n");
sb.append(" apps: ").append(toIndentedString(apps)).append("\n");
sb.append(" groups: ").append(toIndentedString(groups)).append("\n");
sb.append(" roles: ").append(toIndentedString(roles)).append("\n");
sb.append(" disclaimers: ").append(toIndentedString(disclaimers)).append("\n");
sb.append(" avatar: ").append(toIndentedString(avatar)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy