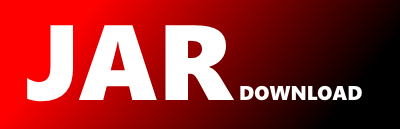
com.symphony.api.model.V3DLPPolicyRequest Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V3DLPPolicyAppliesTo;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Request to be used to get policies.
**/
@Schema(description="Request to be used to get policies.")
public class V3DLPPolicyRequest {
@Schema(required = true, description = "Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed. ")
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
**/
private String name = null;
@Schema(required = true, description = "List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"]. ")
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
**/
private List scopes = new ArrayList();
@Schema(required = true, description = "")
private List appliesTo = new ArrayList();
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public V3DLPPolicyRequest name(String name) {
this.name = name;
return this;
}
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
* @return scopes
**/
@JsonProperty("scopes")
public List getScopes() {
return scopes;
}
public void setScopes(List scopes) {
this.scopes = scopes;
}
public V3DLPPolicyRequest scopes(List scopes) {
this.scopes = scopes;
return this;
}
public V3DLPPolicyRequest addScopesItem(String scopesItem) {
this.scopes.add(scopesItem);
return this;
}
/**
* Get appliesTo
* @return appliesTo
**/
@JsonProperty("appliesTo")
public List getAppliesTo() {
return appliesTo;
}
public void setAppliesTo(List appliesTo) {
this.appliesTo = appliesTo;
}
public V3DLPPolicyRequest appliesTo(List appliesTo) {
this.appliesTo = appliesTo;
return this;
}
public V3DLPPolicyRequest addAppliesToItem(V3DLPPolicyAppliesTo appliesToItem) {
this.appliesTo.add(appliesToItem);
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V3DLPPolicyRequest {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" scopes: ").append(toIndentedString(scopes)).append("\n");
sb.append(" appliesTo: ").append(toIndentedString(appliesTo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy