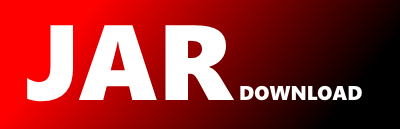
com.symphony.api.model.V3DLPRule Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V3DLPFileClassifierConfig;
import com.symphony.api.model.V3DLPFileExtensionConfig;
import com.symphony.api.model.V3DLPFilePasswordConfig;
import com.symphony.api.model.V3DLPFileSizeConfig;
import com.symphony.api.model.V3DLPTextMatchConfig;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* A Rule defines the actual matching specification for policies. It holds a type and a configuration for the rule, these properties should be used to build the corresponding matching implementation. Only one of the configuration property should be set [textMatchConfig, fileSizeConfig, fileExtensionConfig, filePasswordConfig, fileClassifierConfig].
**/
@Schema(description="A Rule defines the actual matching specification for policies. It holds a type and a configuration for the rule, these properties should be used to build the corresponding matching implementation. Only one of the configuration property should be set [textMatchConfig, fileSizeConfig, fileExtensionConfig, filePasswordConfig, fileClassifierConfig]. ")
public class V3DLPRule {
@Schema(description = "")
private String id = null;
@Schema(required = true, description = "Type of a rule used by policy. Can be [\"UNKNOWN\", \"TEXT_MATCH\", \"FILE_EXTENSION\", \"FILE_SIZE\", \"FILE_PASSWORD\", \"FILE_CLASSIFIER\"].")
/**
* Type of a rule used by policy. Can be [\"UNKNOWN\", \"TEXT_MATCH\", \"FILE_EXTENSION\", \"FILE_SIZE\", \"FILE_PASSWORD\", \"FILE_CLASSIFIER\"].
**/
private String type = null;
@Schema(required = true, description = "Name for rule.")
/**
* Name for rule.
**/
private String name = null;
@Schema(description = "")
private V3DLPTextMatchConfig textMatchConfig = null;
@Schema(description = "")
private V3DLPFileSizeConfig fileSizeConfig = null;
@Schema(description = "")
private V3DLPFileExtensionConfig fileExtensionConfig = null;
@Schema(description = "")
private V3DLPFilePasswordConfig filePasswordConfig = null;
@Schema(description = "")
private V3DLPFileClassifierConfig fileClassifierConfig = null;
/**
* Get id
* @return id
**/
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public V3DLPRule id(String id) {
this.id = id;
return this;
}
/**
* Type of a rule used by policy. Can be [\"UNKNOWN\", \"TEXT_MATCH\", \"FILE_EXTENSION\", \"FILE_SIZE\", \"FILE_PASSWORD\", \"FILE_CLASSIFIER\"].
* @return type
**/
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V3DLPRule type(String type) {
this.type = type;
return this;
}
/**
* Name for rule.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public V3DLPRule name(String name) {
this.name = name;
return this;
}
/**
* Get textMatchConfig
* @return textMatchConfig
**/
@JsonProperty("textMatchConfig")
public V3DLPTextMatchConfig getTextMatchConfig() {
return textMatchConfig;
}
public void setTextMatchConfig(V3DLPTextMatchConfig textMatchConfig) {
this.textMatchConfig = textMatchConfig;
}
public V3DLPRule textMatchConfig(V3DLPTextMatchConfig textMatchConfig) {
this.textMatchConfig = textMatchConfig;
return this;
}
/**
* Get fileSizeConfig
* @return fileSizeConfig
**/
@JsonProperty("fileSizeConfig")
public V3DLPFileSizeConfig getFileSizeConfig() {
return fileSizeConfig;
}
public void setFileSizeConfig(V3DLPFileSizeConfig fileSizeConfig) {
this.fileSizeConfig = fileSizeConfig;
}
public V3DLPRule fileSizeConfig(V3DLPFileSizeConfig fileSizeConfig) {
this.fileSizeConfig = fileSizeConfig;
return this;
}
/**
* Get fileExtensionConfig
* @return fileExtensionConfig
**/
@JsonProperty("fileExtensionConfig")
public V3DLPFileExtensionConfig getFileExtensionConfig() {
return fileExtensionConfig;
}
public void setFileExtensionConfig(V3DLPFileExtensionConfig fileExtensionConfig) {
this.fileExtensionConfig = fileExtensionConfig;
}
public V3DLPRule fileExtensionConfig(V3DLPFileExtensionConfig fileExtensionConfig) {
this.fileExtensionConfig = fileExtensionConfig;
return this;
}
/**
* Get filePasswordConfig
* @return filePasswordConfig
**/
@JsonProperty("filePasswordConfig")
public V3DLPFilePasswordConfig getFilePasswordConfig() {
return filePasswordConfig;
}
public void setFilePasswordConfig(V3DLPFilePasswordConfig filePasswordConfig) {
this.filePasswordConfig = filePasswordConfig;
}
public V3DLPRule filePasswordConfig(V3DLPFilePasswordConfig filePasswordConfig) {
this.filePasswordConfig = filePasswordConfig;
return this;
}
/**
* Get fileClassifierConfig
* @return fileClassifierConfig
**/
@JsonProperty("fileClassifierConfig")
public V3DLPFileClassifierConfig getFileClassifierConfig() {
return fileClassifierConfig;
}
public void setFileClassifierConfig(V3DLPFileClassifierConfig fileClassifierConfig) {
this.fileClassifierConfig = fileClassifierConfig;
}
public V3DLPRule fileClassifierConfig(V3DLPFileClassifierConfig fileClassifierConfig) {
this.fileClassifierConfig = fileClassifierConfig;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V3DLPRule {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" textMatchConfig: ").append(toIndentedString(textMatchConfig)).append("\n");
sb.append(" fileSizeConfig: ").append(toIndentedString(fileSizeConfig)).append("\n");
sb.append(" fileExtensionConfig: ").append(toIndentedString(fileExtensionConfig)).append("\n");
sb.append(" filePasswordConfig: ").append(toIndentedString(filePasswordConfig)).append("\n");
sb.append(" fileClassifierConfig: ").append(toIndentedString(fileClassifierConfig)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy