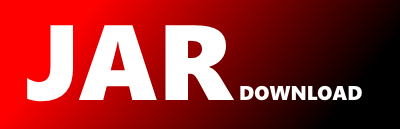
com.symphony.api.model.V3DLPViolation Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V1DLPOutcome;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* A representation of a violation due to an event created by a user of Symphony
**/
@Schema(description="A representation of a violation due to an event created by a user of Symphony")
public class V3DLPViolation {
@Schema(description = "Enforcement event ID. Unique ID that identifies this enforcement.")
/**
* Enforcement event ID. Unique ID that identifies this enforcement.
**/
private String enforcementEventID = null;
@Schema(description = "Entity ID is the content Id of the violation, for example, for messages, its the Id of the message")
/**
* Entity ID is the content Id of the violation, for example, for messages, its the Id of the message
**/
private String entityID = null;
@Schema(description = "Timestamp of the violation in milliseconds since Jan 1 1970")
/**
* Timestamp of the violation in milliseconds since Jan 1 1970
**/
private Long createTime = null;
@Schema(description = "Timestamp of the last modification of violation in milliseconds since Jan 1 1970")
/**
* Timestamp of the last modification of violation in milliseconds since Jan 1 1970
**/
private Long lastModified = null;
@Schema(description = "Id of the requester responsible for the message/stream/signal")
/**
* Id of the requester responsible for the message/stream/signal
**/
private Long requesterId = null;
@Schema(description = "JSON representation of the details of the violation.")
/**
* JSON representation of the details of the violation.
**/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy