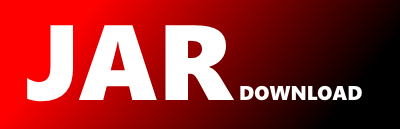
com.symphony.api.model.V3DLPViolationMessage Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V3DLPViolation;
import com.symphony.api.model.V4Message;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V3DLPViolationMessage {
@Schema(description = "")
private V3DLPViolation violation = null;
@Schema(description = "")
private V4Message message = null;
@Schema(description = "")
private V4Message sharedMessage = null;
@Schema(description = "A diagnostic message containing an error message in the event there are parsing errors. May also be present in the case of a successful call if there is useful narrative to return. ")
/**
* A diagnostic message containing an error message in the event there are parsing errors. May also be present in the case of a successful call if there is useful narrative to return.
**/
private String diagnostic = null;
/**
* Get violation
* @return violation
**/
@JsonProperty("violation")
public V3DLPViolation getViolation() {
return violation;
}
public void setViolation(V3DLPViolation violation) {
this.violation = violation;
}
public V3DLPViolationMessage violation(V3DLPViolation violation) {
this.violation = violation;
return this;
}
/**
* Get message
* @return message
**/
@JsonProperty("message")
public V4Message getMessage() {
return message;
}
public void setMessage(V4Message message) {
this.message = message;
}
public V3DLPViolationMessage message(V4Message message) {
this.message = message;
return this;
}
/**
* Get sharedMessage
* @return sharedMessage
**/
@JsonProperty("sharedMessage")
public V4Message getSharedMessage() {
return sharedMessage;
}
public void setSharedMessage(V4Message sharedMessage) {
this.sharedMessage = sharedMessage;
}
public V3DLPViolationMessage sharedMessage(V4Message sharedMessage) {
this.sharedMessage = sharedMessage;
return this;
}
/**
* A diagnostic message containing an error message in the event there are parsing errors. May also be present in the case of a successful call if there is useful narrative to return.
* @return diagnostic
**/
@JsonProperty("diagnostic")
public String getDiagnostic() {
return diagnostic;
}
public void setDiagnostic(String diagnostic) {
this.diagnostic = diagnostic;
}
public V3DLPViolationMessage diagnostic(String diagnostic) {
this.diagnostic = diagnostic;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V3DLPViolationMessage {\n");
sb.append(" violation: ").append(toIndentedString(violation)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" sharedMessage: ").append(toIndentedString(sharedMessage)).append("\n");
sb.append(" diagnostic: ").append(toIndentedString(diagnostic)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy