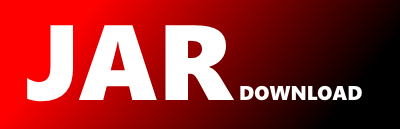
com.symphony.api.model.V3RoomAttributes Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.RoomTag;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V3RoomAttributes {
@Schema(description = "Room name.")
/**
* Room name.
**/
private String name = null;
@Schema(description = "Keywords for search to use to find this room")
/**
* Keywords for search to use to find this room
**/
private List keywords = null;
@Schema(description = "Room description.")
/**
* Room description.
**/
private String description = null;
@Schema(description = "If true, any chatroom participant can add new participants. If false, only owners can add new participants.")
/**
* If true, any chatroom participant can add new participants. If false, only owners can add new participants.
**/
private Boolean membersCanInvite = null;
@Schema(description = "If true, this chatroom (name, description and messages) can be searched and listed by non-participants. If false, only participants can search this room.")
/**
* If true, this chatroom (name, description and messages) can be searched and listed by non-participants. If false, only participants can search this room.
**/
private Boolean discoverable = null;
@Schema(description = "If true, this is a public chatroom. IF false, a private chatroom.")
/**
* If true, this is a public chatroom. IF false, a private chatroom.
**/
private Boolean _public = null;
@Schema(description = "If true, only stream owners can send messages.")
/**
* If true, only stream owners can send messages.
**/
private Boolean readOnly = null;
@Schema(description = "If true, clients disable the clipboard copy for content in this stream.")
/**
* If true, clients disable the clipboard copy for content in this stream.
**/
private Boolean copyProtected = null;
@Schema(description = "If true, this room is a cross pod room")
/**
* If true, this room is a cross pod room
**/
private Boolean crossPod = null;
@Schema(description = "If true, new members can view the room chat history of the room.")
/**
* If true, new members can view the room chat history of the room.
**/
private Boolean viewHistory = null;
@Schema(description = "If true, this is a multi lateral room where we can find users belonging to more than 2 companies.")
/**
* If true, this is a multi lateral room where we can find users belonging to more than 2 companies.
**/
private Boolean multiLateralRoom = null;
@Schema(description = "If true, this room is for a scheduled meeting.")
/**
* If true, this room is for a scheduled meeting.
**/
private Boolean scheduledMeeting = null;
@Schema(description = "Possible value EMAIL (indicate this room will be used for Email Integration)")
/**
* Possible value EMAIL (indicate this room will be used for Email Integration)
**/
private String subType = null;
@Schema(description = "UrlSafe message id of the pinned message inside the room. To perform unpin operation, send an empty string.")
/**
* UrlSafe message id of the pinned message inside the room. To perform unpin operation, send an empty string.
**/
private String pinnedMessageId = null;
/**
* Room name.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public V3RoomAttributes name(String name) {
this.name = name;
return this;
}
/**
* Keywords for search to use to find this room
* @return keywords
**/
@JsonProperty("keywords")
public List getKeywords() {
return keywords;
}
public void setKeywords(List keywords) {
this.keywords = keywords;
}
public V3RoomAttributes keywords(List keywords) {
this.keywords = keywords;
return this;
}
public V3RoomAttributes addKeywordsItem(RoomTag keywordsItem) {
this.keywords.add(keywordsItem);
return this;
}
/**
* Room description.
* @return description
**/
@JsonProperty("description")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public V3RoomAttributes description(String description) {
this.description = description;
return this;
}
/**
* If true, any chatroom participant can add new participants. If false, only owners can add new participants.
* @return membersCanInvite
**/
@JsonProperty("membersCanInvite")
public Boolean isMembersCanInvite() {
return membersCanInvite;
}
public void setMembersCanInvite(Boolean membersCanInvite) {
this.membersCanInvite = membersCanInvite;
}
public V3RoomAttributes membersCanInvite(Boolean membersCanInvite) {
this.membersCanInvite = membersCanInvite;
return this;
}
/**
* If true, this chatroom (name, description and messages) can be searched and listed by non-participants. If false, only participants can search this room.
* @return discoverable
**/
@JsonProperty("discoverable")
public Boolean isDiscoverable() {
return discoverable;
}
public void setDiscoverable(Boolean discoverable) {
this.discoverable = discoverable;
}
public V3RoomAttributes discoverable(Boolean discoverable) {
this.discoverable = discoverable;
return this;
}
/**
* If true, this is a public chatroom. IF false, a private chatroom.
* @return _public
**/
@JsonProperty("public")
public Boolean isPublic() {
return _public;
}
public void setPublic(Boolean _public) {
this._public = _public;
}
public V3RoomAttributes _public(Boolean _public) {
this._public = _public;
return this;
}
/**
* If true, only stream owners can send messages.
* @return readOnly
**/
@JsonProperty("readOnly")
public Boolean isReadOnly() {
return readOnly;
}
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
public V3RoomAttributes readOnly(Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
/**
* If true, clients disable the clipboard copy for content in this stream.
* @return copyProtected
**/
@JsonProperty("copyProtected")
public Boolean isCopyProtected() {
return copyProtected;
}
public void setCopyProtected(Boolean copyProtected) {
this.copyProtected = copyProtected;
}
public V3RoomAttributes copyProtected(Boolean copyProtected) {
this.copyProtected = copyProtected;
return this;
}
/**
* If true, this room is a cross pod room
* @return crossPod
**/
@JsonProperty("crossPod")
public Boolean isCrossPod() {
return crossPod;
}
public void setCrossPod(Boolean crossPod) {
this.crossPod = crossPod;
}
public V3RoomAttributes crossPod(Boolean crossPod) {
this.crossPod = crossPod;
return this;
}
/**
* If true, new members can view the room chat history of the room.
* @return viewHistory
**/
@JsonProperty("viewHistory")
public Boolean isViewHistory() {
return viewHistory;
}
public void setViewHistory(Boolean viewHistory) {
this.viewHistory = viewHistory;
}
public V3RoomAttributes viewHistory(Boolean viewHistory) {
this.viewHistory = viewHistory;
return this;
}
/**
* If true, this is a multi lateral room where we can find users belonging to more than 2 companies.
* @return multiLateralRoom
**/
@JsonProperty("multiLateralRoom")
public Boolean isMultiLateralRoom() {
return multiLateralRoom;
}
public void setMultiLateralRoom(Boolean multiLateralRoom) {
this.multiLateralRoom = multiLateralRoom;
}
public V3RoomAttributes multiLateralRoom(Boolean multiLateralRoom) {
this.multiLateralRoom = multiLateralRoom;
return this;
}
/**
* If true, this room is for a scheduled meeting.
* @return scheduledMeeting
**/
@JsonProperty("scheduledMeeting")
public Boolean isScheduledMeeting() {
return scheduledMeeting;
}
public void setScheduledMeeting(Boolean scheduledMeeting) {
this.scheduledMeeting = scheduledMeeting;
}
public V3RoomAttributes scheduledMeeting(Boolean scheduledMeeting) {
this.scheduledMeeting = scheduledMeeting;
return this;
}
/**
* Possible value EMAIL (indicate this room will be used for Email Integration)
* @return subType
**/
@JsonProperty("subType")
public String getSubType() {
return subType;
}
public void setSubType(String subType) {
this.subType = subType;
}
public V3RoomAttributes subType(String subType) {
this.subType = subType;
return this;
}
/**
* UrlSafe message id of the pinned message inside the room. To perform unpin operation, send an empty string.
* @return pinnedMessageId
**/
@JsonProperty("pinnedMessageId")
public String getPinnedMessageId() {
return pinnedMessageId;
}
public void setPinnedMessageId(String pinnedMessageId) {
this.pinnedMessageId = pinnedMessageId;
}
public V3RoomAttributes pinnedMessageId(String pinnedMessageId) {
this.pinnedMessageId = pinnedMessageId;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V3RoomAttributes {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" keywords: ").append(toIndentedString(keywords)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" membersCanInvite: ").append(toIndentedString(membersCanInvite)).append("\n");
sb.append(" discoverable: ").append(toIndentedString(discoverable)).append("\n");
sb.append(" _public: ").append(toIndentedString(_public)).append("\n");
sb.append(" readOnly: ").append(toIndentedString(readOnly)).append("\n");
sb.append(" copyProtected: ").append(toIndentedString(copyProtected)).append("\n");
sb.append(" crossPod: ").append(toIndentedString(crossPod)).append("\n");
sb.append(" viewHistory: ").append(toIndentedString(viewHistory)).append("\n");
sb.append(" multiLateralRoom: ").append(toIndentedString(multiLateralRoom)).append("\n");
sb.append(" scheduledMeeting: ").append(toIndentedString(scheduledMeeting)).append("\n");
sb.append(" subType: ").append(toIndentedString(subType)).append("\n");
sb.append(" pinnedMessageId: ").append(toIndentedString(pinnedMessageId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy