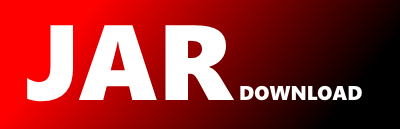
com.symphony.api.model.V4Event Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V4Initiator;
import com.symphony.api.model.V4Payload;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V4Event {
@Schema(description = "Event ID")
/**
* Event ID
**/
private String id = null;
@Schema(description = "Message ID")
/**
* Message ID
**/
private String messageId = null;
@Schema(description = "Timestamp of event")
/**
* Timestamp of event
**/
private Long timestamp = null;
@Schema(description = "Event type, possible events are: - MESSAGESENT - SHAREDPOST - INSTANTMESSAGECREATED - ROOMCREATED - ROOMUPDATED - ROOMDEACTIVATED - ROOMREACTIVATED - USERJOINEDROOM - USERLEFTROOM - ROOMMEMBERPROMOTEDTOOWNER - ROOMMEMBERDEMOTEDFROMOWNER - CONNECTIONREQUESTED - CONNECTIONACCEPTED - MESSAGESUPPRESSED - SYMPHONYELEMENTSACTION - USERREQUESTEDTOJOINROOM ")
/**
* Event type, possible events are: - MESSAGESENT - SHAREDPOST - INSTANTMESSAGECREATED - ROOMCREATED - ROOMUPDATED - ROOMDEACTIVATED - ROOMREACTIVATED - USERJOINEDROOM - USERLEFTROOM - ROOMMEMBERPROMOTEDTOOWNER - ROOMMEMBERDEMOTEDFROMOWNER - CONNECTIONREQUESTED - CONNECTIONACCEPTED - MESSAGESUPPRESSED - SYMPHONYELEMENTSACTION - USERREQUESTEDTOJOINROOM
**/
private String type = null;
@Schema(description = "Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs. ")
/**
* Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs.
**/
private String diagnostic = null;
@Schema(description = "")
private V4Initiator initiator = null;
@Schema(description = "")
private V4Payload payload = null;
/**
* Event ID
* @return id
**/
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public V4Event id(String id) {
this.id = id;
return this;
}
/**
* Message ID
* @return messageId
**/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public V4Event messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Timestamp of event
* @return timestamp
**/
@JsonProperty("timestamp")
public Long getTimestamp() {
return timestamp;
}
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp;
}
public V4Event timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Event type, possible events are: - MESSAGESENT - SHAREDPOST - INSTANTMESSAGECREATED - ROOMCREATED - ROOMUPDATED - ROOMDEACTIVATED - ROOMREACTIVATED - USERJOINEDROOM - USERLEFTROOM - ROOMMEMBERPROMOTEDTOOWNER - ROOMMEMBERDEMOTEDFROMOWNER - CONNECTIONREQUESTED - CONNECTIONACCEPTED - MESSAGESUPPRESSED - SYMPHONYELEMENTSACTION - USERREQUESTEDTOJOINROOM
* @return type
**/
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V4Event type(String type) {
this.type = type;
return this;
}
/**
* Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs.
* @return diagnostic
**/
@JsonProperty("diagnostic")
public String getDiagnostic() {
return diagnostic;
}
public void setDiagnostic(String diagnostic) {
this.diagnostic = diagnostic;
}
public V4Event diagnostic(String diagnostic) {
this.diagnostic = diagnostic;
return this;
}
/**
* Get initiator
* @return initiator
**/
@JsonProperty("initiator")
public V4Initiator getInitiator() {
return initiator;
}
public void setInitiator(V4Initiator initiator) {
this.initiator = initiator;
}
public V4Event initiator(V4Initiator initiator) {
this.initiator = initiator;
return this;
}
/**
* Get payload
* @return payload
**/
@JsonProperty("payload")
public V4Payload getPayload() {
return payload;
}
public void setPayload(V4Payload payload) {
this.payload = payload;
}
public V4Event payload(V4Payload payload) {
this.payload = payload;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V4Event {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" diagnostic: ").append(toIndentedString(diagnostic)).append("\n");
sb.append(" initiator: ").append(toIndentedString(initiator)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy