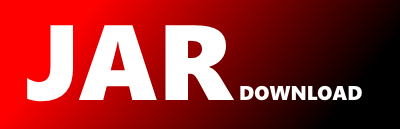
com.symphony.api.model.V4ImportedMessage Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* A historic message to be imported into the system. The importing user must have the Content Management role. Also, the importing user must be a member of the conversation it is importing into. The user that the message is intended to have come from must also be present in the conversation. The intended message timestamp must be a valid time from the past. It cannot be a future timestamp. By design, imported messages do not stream to datafeed or firehose endpoints.
**/
@Schema(description="A historic message to be imported into the system. The importing user must have the Content Management role. Also, the importing user must be a member of the conversation it is importing into. The user that the message is intended to have come from must also be present in the conversation. The intended message timestamp must be a valid time from the past. It cannot be a future timestamp. By design, imported messages do not stream to datafeed or firehose endpoints. ")
public class V4ImportedMessage {
@Schema(required = true, description = "Message text in MessageMLV2")
/**
* Message text in MessageMLV2
**/
private String message = null;
@Schema(description = "Entity data in EntityJSON")
/**
* Entity data in EntityJSON
**/
private String data = null;
@Schema(required = true, description = "The timestamp representing the time when the message was sent in the original system in milliseconds since Jan 1st 1970. ")
/**
* The timestamp representing the time when the message was sent in the original system in milliseconds since Jan 1st 1970.
**/
private Long intendedMessageTimestamp = null;
@Schema(required = true, description = "The long integer userid of the Symphony user who you intend to show sent the message. ")
/**
* The long integer userid of the Symphony user who you intend to show sent the message.
**/
private Long intendedMessageFromUserId = null;
@Schema(required = true, description = "The ID of the system through which the message was originally sent. ")
/**
* The ID of the system through which the message was originally sent.
**/
private String originatingSystemId = null;
@Schema(description = "The ID of the message in the original system. ")
/**
* The ID of the message in the original system.
**/
private String originalMessageId = null;
@Schema(required = true, description = "")
private String streamId = null;
/**
* Message text in MessageMLV2
* @return message
**/
@JsonProperty("message")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public V4ImportedMessage message(String message) {
this.message = message;
return this;
}
/**
* Entity data in EntityJSON
* @return data
**/
@JsonProperty("data")
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
public V4ImportedMessage data(String data) {
this.data = data;
return this;
}
/**
* The timestamp representing the time when the message was sent in the original system in milliseconds since Jan 1st 1970.
* @return intendedMessageTimestamp
**/
@JsonProperty("intendedMessageTimestamp")
public Long getIntendedMessageTimestamp() {
return intendedMessageTimestamp;
}
public void setIntendedMessageTimestamp(Long intendedMessageTimestamp) {
this.intendedMessageTimestamp = intendedMessageTimestamp;
}
public V4ImportedMessage intendedMessageTimestamp(Long intendedMessageTimestamp) {
this.intendedMessageTimestamp = intendedMessageTimestamp;
return this;
}
/**
* The long integer userid of the Symphony user who you intend to show sent the message.
* @return intendedMessageFromUserId
**/
@JsonProperty("intendedMessageFromUserId")
public Long getIntendedMessageFromUserId() {
return intendedMessageFromUserId;
}
public void setIntendedMessageFromUserId(Long intendedMessageFromUserId) {
this.intendedMessageFromUserId = intendedMessageFromUserId;
}
public V4ImportedMessage intendedMessageFromUserId(Long intendedMessageFromUserId) {
this.intendedMessageFromUserId = intendedMessageFromUserId;
return this;
}
/**
* The ID of the system through which the message was originally sent.
* @return originatingSystemId
**/
@JsonProperty("originatingSystemId")
public String getOriginatingSystemId() {
return originatingSystemId;
}
public void setOriginatingSystemId(String originatingSystemId) {
this.originatingSystemId = originatingSystemId;
}
public V4ImportedMessage originatingSystemId(String originatingSystemId) {
this.originatingSystemId = originatingSystemId;
return this;
}
/**
* The ID of the message in the original system.
* @return originalMessageId
**/
@JsonProperty("originalMessageId")
public String getOriginalMessageId() {
return originalMessageId;
}
public void setOriginalMessageId(String originalMessageId) {
this.originalMessageId = originalMessageId;
}
public V4ImportedMessage originalMessageId(String originalMessageId) {
this.originalMessageId = originalMessageId;
return this;
}
/**
* Get streamId
* @return streamId
**/
@JsonProperty("streamId")
public String getStreamId() {
return streamId;
}
public void setStreamId(String streamId) {
this.streamId = streamId;
}
public V4ImportedMessage streamId(String streamId) {
this.streamId = streamId;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V4ImportedMessage {\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" intendedMessageTimestamp: ").append(toIndentedString(intendedMessageTimestamp)).append("\n");
sb.append(" intendedMessageFromUserId: ").append(toIndentedString(intendedMessageFromUserId)).append("\n");
sb.append(" originatingSystemId: ").append(toIndentedString(originatingSystemId)).append("\n");
sb.append(" originalMessageId: ").append(toIndentedString(originalMessageId)).append("\n");
sb.append(" streamId: ").append(toIndentedString(streamId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy