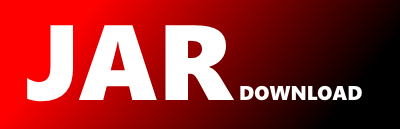
com.symphony.api.model.V4Message Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V4AttachmentInfo;
import com.symphony.api.model.V4Message;
import com.symphony.api.model.V4Stream;
import com.symphony.api.model.V4User;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* A representation of a message sent by a user of Symphony
**/
@Schema(description="A representation of a message sent by a user of Symphony")
public class V4Message {
@Schema(description = "Id of the message")
/**
* Id of the message
**/
private String messageId = null;
@Schema(description = "Timestamp of the message in milliseconds since Jan 1 1970")
/**
* Timestamp of the message in milliseconds since Jan 1 1970
**/
private Long timestamp = null;
@Schema(description = "Message content in MessageMLV2")
/**
* Message content in MessageMLV2
**/
private String message = null;
@Schema(description = "")
private V4Message sharedMessage = null;
@Schema(description = "Message data in EntityJSON")
/**
* Message data in EntityJSON
**/
private String data = null;
@Schema(description = "Message attachments")
/**
* Message attachments
**/
private List attachments = null;
@Schema(description = "")
private V4User user = null;
@Schema(description = "")
private V4Stream stream = null;
@Schema(description = "Indicates if the message have external recipients. Only present on real time messaging.")
/**
* Indicates if the message have external recipients. Only present on real time messaging.
**/
private Boolean externalRecipients = null;
@Schema(description = "Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs. ")
/**
* Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs.
**/
private String diagnostic = null;
@Schema(description = "User agent string for client that sent the message. Allows callers to identify which client sent the origin message (e.g. API Agent, SFE Client, mobile, etc) ")
/**
* User agent string for client that sent the message. Allows callers to identify which client sent the origin message (e.g. API Agent, SFE Client, mobile, etc)
**/
private String userAgent = null;
@Schema(description = "Indicates the format in which the message was originally sent. This could have been either: - com.symphony.markdown - Markdown OR Message ML V1 - com.symphony.messageml.v2 - Message ML V2 ")
/**
* Indicates the format in which the message was originally sent. This could have been either: - com.symphony.markdown - Markdown OR Message ML V1 - com.symphony.messageml.v2 - Message ML V2
**/
private String originalFormat = null;
@Schema(description = "Message that may be sent along with a regular message if configured for the POD, usually the first message sent in a room that day. ")
/**
* Message that may be sent along with a regular message if configured for the POD, usually the first message sent in a room that day.
**/
private String disclaimer = null;
@Schema(example = "fa691cd3-484a-4109-aeb2-57c05b78c95b", description = "Unique session identifier from where the message was created. ")
/**
* Unique session identifier from where the message was created.
**/
private String sid = null;
@Schema(description = "Id of the message that the current message is replacing (present only if set)")
/**
* Id of the message that the current message is replacing (present only if set)
**/
private String replacing = null;
@Schema(description = "Id of the message that the current message is being replaced with (present only if set)")
/**
* Id of the message that the current message is being replaced with (present only if set)
**/
private String replacedBy = null;
@Schema(description = "Timestamp of when the initial message has been created in milliseconds since Jan 1 1970 (present only if set)")
/**
* Timestamp of when the initial message has been created in milliseconds since Jan 1 1970 (present only if set)
**/
private Long initialTimestamp = null;
@Schema(description = "Id the the initial message that has been updated (present only if set)")
/**
* Id the the initial message that has been updated (present only if set)
**/
private String initialMessageId = null;
/**
* Id of the message
* @return messageId
**/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public V4Message messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Timestamp of the message in milliseconds since Jan 1 1970
* @return timestamp
**/
@JsonProperty("timestamp")
public Long getTimestamp() {
return timestamp;
}
public void setTimestamp(Long timestamp) {
this.timestamp = timestamp;
}
public V4Message timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Message content in MessageMLV2
* @return message
**/
@JsonProperty("message")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public V4Message message(String message) {
this.message = message;
return this;
}
/**
* Get sharedMessage
* @return sharedMessage
**/
@JsonProperty("sharedMessage")
public V4Message getSharedMessage() {
return sharedMessage;
}
public void setSharedMessage(V4Message sharedMessage) {
this.sharedMessage = sharedMessage;
}
public V4Message sharedMessage(V4Message sharedMessage) {
this.sharedMessage = sharedMessage;
return this;
}
/**
* Message data in EntityJSON
* @return data
**/
@JsonProperty("data")
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
public V4Message data(String data) {
this.data = data;
return this;
}
/**
* Message attachments
* @return attachments
**/
@JsonProperty("attachments")
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public V4Message attachments(List attachments) {
this.attachments = attachments;
return this;
}
public V4Message addAttachmentsItem(V4AttachmentInfo attachmentsItem) {
this.attachments.add(attachmentsItem);
return this;
}
/**
* Get user
* @return user
**/
@JsonProperty("user")
public V4User getUser() {
return user;
}
public void setUser(V4User user) {
this.user = user;
}
public V4Message user(V4User user) {
this.user = user;
return this;
}
/**
* Get stream
* @return stream
**/
@JsonProperty("stream")
public V4Stream getStream() {
return stream;
}
public void setStream(V4Stream stream) {
this.stream = stream;
}
public V4Message stream(V4Stream stream) {
this.stream = stream;
return this;
}
/**
* Indicates if the message have external recipients. Only present on real time messaging.
* @return externalRecipients
**/
@JsonProperty("externalRecipients")
public Boolean isExternalRecipients() {
return externalRecipients;
}
public void setExternalRecipients(Boolean externalRecipients) {
this.externalRecipients = externalRecipients;
}
public V4Message externalRecipients(Boolean externalRecipients) {
this.externalRecipients = externalRecipients;
return this;
}
/**
* Details if event failed to parse for any reason. The contents of this field may not be useful, depending on the nature of the error. Only present when error occurs.
* @return diagnostic
**/
@JsonProperty("diagnostic")
public String getDiagnostic() {
return diagnostic;
}
public void setDiagnostic(String diagnostic) {
this.diagnostic = diagnostic;
}
public V4Message diagnostic(String diagnostic) {
this.diagnostic = diagnostic;
return this;
}
/**
* User agent string for client that sent the message. Allows callers to identify which client sent the origin message (e.g. API Agent, SFE Client, mobile, etc)
* @return userAgent
**/
@JsonProperty("userAgent")
public String getUserAgent() {
return userAgent;
}
public void setUserAgent(String userAgent) {
this.userAgent = userAgent;
}
public V4Message userAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
/**
* Indicates the format in which the message was originally sent. This could have been either: - com.symphony.markdown - Markdown OR Message ML V1 - com.symphony.messageml.v2 - Message ML V2
* @return originalFormat
**/
@JsonProperty("originalFormat")
public String getOriginalFormat() {
return originalFormat;
}
public void setOriginalFormat(String originalFormat) {
this.originalFormat = originalFormat;
}
public V4Message originalFormat(String originalFormat) {
this.originalFormat = originalFormat;
return this;
}
/**
* Message that may be sent along with a regular message if configured for the POD, usually the first message sent in a room that day.
* @return disclaimer
**/
@JsonProperty("disclaimer")
public String getDisclaimer() {
return disclaimer;
}
public void setDisclaimer(String disclaimer) {
this.disclaimer = disclaimer;
}
public V4Message disclaimer(String disclaimer) {
this.disclaimer = disclaimer;
return this;
}
/**
* Unique session identifier from where the message was created.
* @return sid
**/
@JsonProperty("sid")
public String getSid() {
return sid;
}
public void setSid(String sid) {
this.sid = sid;
}
public V4Message sid(String sid) {
this.sid = sid;
return this;
}
/**
* Id of the message that the current message is replacing (present only if set)
* @return replacing
**/
@JsonProperty("replacing")
public String getReplacing() {
return replacing;
}
public void setReplacing(String replacing) {
this.replacing = replacing;
}
public V4Message replacing(String replacing) {
this.replacing = replacing;
return this;
}
/**
* Id of the message that the current message is being replaced with (present only if set)
* @return replacedBy
**/
@JsonProperty("replacedBy")
public String getReplacedBy() {
return replacedBy;
}
public void setReplacedBy(String replacedBy) {
this.replacedBy = replacedBy;
}
public V4Message replacedBy(String replacedBy) {
this.replacedBy = replacedBy;
return this;
}
/**
* Timestamp of when the initial message has been created in milliseconds since Jan 1 1970 (present only if set)
* @return initialTimestamp
**/
@JsonProperty("initialTimestamp")
public Long getInitialTimestamp() {
return initialTimestamp;
}
public void setInitialTimestamp(Long initialTimestamp) {
this.initialTimestamp = initialTimestamp;
}
public V4Message initialTimestamp(Long initialTimestamp) {
this.initialTimestamp = initialTimestamp;
return this;
}
/**
* Id the the initial message that has been updated (present only if set)
* @return initialMessageId
**/
@JsonProperty("initialMessageId")
public String getInitialMessageId() {
return initialMessageId;
}
public void setInitialMessageId(String initialMessageId) {
this.initialMessageId = initialMessageId;
}
public V4Message initialMessageId(String initialMessageId) {
this.initialMessageId = initialMessageId;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V4Message {\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" sharedMessage: ").append(toIndentedString(sharedMessage)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" stream: ").append(toIndentedString(stream)).append("\n");
sb.append(" externalRecipients: ").append(toIndentedString(externalRecipients)).append("\n");
sb.append(" diagnostic: ").append(toIndentedString(diagnostic)).append("\n");
sb.append(" userAgent: ").append(toIndentedString(userAgent)).append("\n");
sb.append(" originalFormat: ").append(toIndentedString(originalFormat)).append("\n");
sb.append(" disclaimer: ").append(toIndentedString(disclaimer)).append("\n");
sb.append(" sid: ").append(toIndentedString(sid)).append("\n");
sb.append(" replacing: ").append(toIndentedString(replacing)).append("\n");
sb.append(" replacedBy: ").append(toIndentedString(replacedBy)).append("\n");
sb.append(" initialTimestamp: ").append(toIndentedString(initialTimestamp)).append("\n");
sb.append(" initialMessageId: ").append(toIndentedString(initialMessageId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy