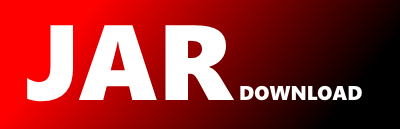
com.symphony.api.model.V4Payload Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V4ConnectionAccepted;
import com.symphony.api.model.V4ConnectionRequested;
import com.symphony.api.model.V4InstantMessageCreated;
import com.symphony.api.model.V4MessageSent;
import com.symphony.api.model.V4MessageSuppressed;
import com.symphony.api.model.V4RoomCreated;
import com.symphony.api.model.V4RoomDeactivated;
import com.symphony.api.model.V4RoomMemberDemotedFromOwner;
import com.symphony.api.model.V4RoomMemberPromotedToOwner;
import com.symphony.api.model.V4RoomReactivated;
import com.symphony.api.model.V4RoomUpdated;
import com.symphony.api.model.V4SharedPost;
import com.symphony.api.model.V4SymphonyElementsAction;
import com.symphony.api.model.V4UserJoinedRoom;
import com.symphony.api.model.V4UserLeftRoom;
import com.symphony.api.model.V4UserRequestedToJoinRoom;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class V4Payload {
@Schema(description = "")
private V4MessageSent messageSent = null;
@Schema(description = "")
private V4SharedPost sharedPost = null;
@Schema(description = "")
private V4InstantMessageCreated instantMessageCreated = null;
@Schema(description = "")
private V4RoomCreated roomCreated = null;
@Schema(description = "")
private V4RoomUpdated roomUpdated = null;
@Schema(description = "")
private V4RoomDeactivated roomDeactivated = null;
@Schema(description = "")
private V4RoomReactivated roomReactivated = null;
@Schema(description = "")
private V4UserJoinedRoom userJoinedRoom = null;
@Schema(description = "")
private V4UserLeftRoom userLeftRoom = null;
@Schema(description = "")
private V4RoomMemberPromotedToOwner roomMemberPromotedToOwner = null;
@Schema(description = "")
private V4RoomMemberDemotedFromOwner roomMemberDemotedFromOwner = null;
@Schema(description = "")
private V4ConnectionRequested connectionRequested = null;
@Schema(description = "")
private V4ConnectionAccepted connectionAccepted = null;
@Schema(description = "")
private V4MessageSuppressed messageSuppressed = null;
@Schema(description = "")
private V4SymphonyElementsAction symphonyElementsAction = null;
@Schema(description = "")
private V4UserRequestedToJoinRoom userRequestedToJoinRoom = null;
/**
* Get messageSent
* @return messageSent
**/
@JsonProperty("messageSent")
public V4MessageSent getMessageSent() {
return messageSent;
}
public void setMessageSent(V4MessageSent messageSent) {
this.messageSent = messageSent;
}
public V4Payload messageSent(V4MessageSent messageSent) {
this.messageSent = messageSent;
return this;
}
/**
* Get sharedPost
* @return sharedPost
**/
@JsonProperty("sharedPost")
public V4SharedPost getSharedPost() {
return sharedPost;
}
public void setSharedPost(V4SharedPost sharedPost) {
this.sharedPost = sharedPost;
}
public V4Payload sharedPost(V4SharedPost sharedPost) {
this.sharedPost = sharedPost;
return this;
}
/**
* Get instantMessageCreated
* @return instantMessageCreated
**/
@JsonProperty("instantMessageCreated")
public V4InstantMessageCreated getInstantMessageCreated() {
return instantMessageCreated;
}
public void setInstantMessageCreated(V4InstantMessageCreated instantMessageCreated) {
this.instantMessageCreated = instantMessageCreated;
}
public V4Payload instantMessageCreated(V4InstantMessageCreated instantMessageCreated) {
this.instantMessageCreated = instantMessageCreated;
return this;
}
/**
* Get roomCreated
* @return roomCreated
**/
@JsonProperty("roomCreated")
public V4RoomCreated getRoomCreated() {
return roomCreated;
}
public void setRoomCreated(V4RoomCreated roomCreated) {
this.roomCreated = roomCreated;
}
public V4Payload roomCreated(V4RoomCreated roomCreated) {
this.roomCreated = roomCreated;
return this;
}
/**
* Get roomUpdated
* @return roomUpdated
**/
@JsonProperty("roomUpdated")
public V4RoomUpdated getRoomUpdated() {
return roomUpdated;
}
public void setRoomUpdated(V4RoomUpdated roomUpdated) {
this.roomUpdated = roomUpdated;
}
public V4Payload roomUpdated(V4RoomUpdated roomUpdated) {
this.roomUpdated = roomUpdated;
return this;
}
/**
* Get roomDeactivated
* @return roomDeactivated
**/
@JsonProperty("roomDeactivated")
public V4RoomDeactivated getRoomDeactivated() {
return roomDeactivated;
}
public void setRoomDeactivated(V4RoomDeactivated roomDeactivated) {
this.roomDeactivated = roomDeactivated;
}
public V4Payload roomDeactivated(V4RoomDeactivated roomDeactivated) {
this.roomDeactivated = roomDeactivated;
return this;
}
/**
* Get roomReactivated
* @return roomReactivated
**/
@JsonProperty("roomReactivated")
public V4RoomReactivated getRoomReactivated() {
return roomReactivated;
}
public void setRoomReactivated(V4RoomReactivated roomReactivated) {
this.roomReactivated = roomReactivated;
}
public V4Payload roomReactivated(V4RoomReactivated roomReactivated) {
this.roomReactivated = roomReactivated;
return this;
}
/**
* Get userJoinedRoom
* @return userJoinedRoom
**/
@JsonProperty("userJoinedRoom")
public V4UserJoinedRoom getUserJoinedRoom() {
return userJoinedRoom;
}
public void setUserJoinedRoom(V4UserJoinedRoom userJoinedRoom) {
this.userJoinedRoom = userJoinedRoom;
}
public V4Payload userJoinedRoom(V4UserJoinedRoom userJoinedRoom) {
this.userJoinedRoom = userJoinedRoom;
return this;
}
/**
* Get userLeftRoom
* @return userLeftRoom
**/
@JsonProperty("userLeftRoom")
public V4UserLeftRoom getUserLeftRoom() {
return userLeftRoom;
}
public void setUserLeftRoom(V4UserLeftRoom userLeftRoom) {
this.userLeftRoom = userLeftRoom;
}
public V4Payload userLeftRoom(V4UserLeftRoom userLeftRoom) {
this.userLeftRoom = userLeftRoom;
return this;
}
/**
* Get roomMemberPromotedToOwner
* @return roomMemberPromotedToOwner
**/
@JsonProperty("roomMemberPromotedToOwner")
public V4RoomMemberPromotedToOwner getRoomMemberPromotedToOwner() {
return roomMemberPromotedToOwner;
}
public void setRoomMemberPromotedToOwner(V4RoomMemberPromotedToOwner roomMemberPromotedToOwner) {
this.roomMemberPromotedToOwner = roomMemberPromotedToOwner;
}
public V4Payload roomMemberPromotedToOwner(V4RoomMemberPromotedToOwner roomMemberPromotedToOwner) {
this.roomMemberPromotedToOwner = roomMemberPromotedToOwner;
return this;
}
/**
* Get roomMemberDemotedFromOwner
* @return roomMemberDemotedFromOwner
**/
@JsonProperty("roomMemberDemotedFromOwner")
public V4RoomMemberDemotedFromOwner getRoomMemberDemotedFromOwner() {
return roomMemberDemotedFromOwner;
}
public void setRoomMemberDemotedFromOwner(V4RoomMemberDemotedFromOwner roomMemberDemotedFromOwner) {
this.roomMemberDemotedFromOwner = roomMemberDemotedFromOwner;
}
public V4Payload roomMemberDemotedFromOwner(V4RoomMemberDemotedFromOwner roomMemberDemotedFromOwner) {
this.roomMemberDemotedFromOwner = roomMemberDemotedFromOwner;
return this;
}
/**
* Get connectionRequested
* @return connectionRequested
**/
@JsonProperty("connectionRequested")
public V4ConnectionRequested getConnectionRequested() {
return connectionRequested;
}
public void setConnectionRequested(V4ConnectionRequested connectionRequested) {
this.connectionRequested = connectionRequested;
}
public V4Payload connectionRequested(V4ConnectionRequested connectionRequested) {
this.connectionRequested = connectionRequested;
return this;
}
/**
* Get connectionAccepted
* @return connectionAccepted
**/
@JsonProperty("connectionAccepted")
public V4ConnectionAccepted getConnectionAccepted() {
return connectionAccepted;
}
public void setConnectionAccepted(V4ConnectionAccepted connectionAccepted) {
this.connectionAccepted = connectionAccepted;
}
public V4Payload connectionAccepted(V4ConnectionAccepted connectionAccepted) {
this.connectionAccepted = connectionAccepted;
return this;
}
/**
* Get messageSuppressed
* @return messageSuppressed
**/
@JsonProperty("messageSuppressed")
public V4MessageSuppressed getMessageSuppressed() {
return messageSuppressed;
}
public void setMessageSuppressed(V4MessageSuppressed messageSuppressed) {
this.messageSuppressed = messageSuppressed;
}
public V4Payload messageSuppressed(V4MessageSuppressed messageSuppressed) {
this.messageSuppressed = messageSuppressed;
return this;
}
/**
* Get symphonyElementsAction
* @return symphonyElementsAction
**/
@JsonProperty("symphonyElementsAction")
public V4SymphonyElementsAction getSymphonyElementsAction() {
return symphonyElementsAction;
}
public void setSymphonyElementsAction(V4SymphonyElementsAction symphonyElementsAction) {
this.symphonyElementsAction = symphonyElementsAction;
}
public V4Payload symphonyElementsAction(V4SymphonyElementsAction symphonyElementsAction) {
this.symphonyElementsAction = symphonyElementsAction;
return this;
}
/**
* Get userRequestedToJoinRoom
* @return userRequestedToJoinRoom
**/
@JsonProperty("userRequestedToJoinRoom")
public V4UserRequestedToJoinRoom getUserRequestedToJoinRoom() {
return userRequestedToJoinRoom;
}
public void setUserRequestedToJoinRoom(V4UserRequestedToJoinRoom userRequestedToJoinRoom) {
this.userRequestedToJoinRoom = userRequestedToJoinRoom;
}
public V4Payload userRequestedToJoinRoom(V4UserRequestedToJoinRoom userRequestedToJoinRoom) {
this.userRequestedToJoinRoom = userRequestedToJoinRoom;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V4Payload {\n");
sb.append(" messageSent: ").append(toIndentedString(messageSent)).append("\n");
sb.append(" sharedPost: ").append(toIndentedString(sharedPost)).append("\n");
sb.append(" instantMessageCreated: ").append(toIndentedString(instantMessageCreated)).append("\n");
sb.append(" roomCreated: ").append(toIndentedString(roomCreated)).append("\n");
sb.append(" roomUpdated: ").append(toIndentedString(roomUpdated)).append("\n");
sb.append(" roomDeactivated: ").append(toIndentedString(roomDeactivated)).append("\n");
sb.append(" roomReactivated: ").append(toIndentedString(roomReactivated)).append("\n");
sb.append(" userJoinedRoom: ").append(toIndentedString(userJoinedRoom)).append("\n");
sb.append(" userLeftRoom: ").append(toIndentedString(userLeftRoom)).append("\n");
sb.append(" roomMemberPromotedToOwner: ").append(toIndentedString(roomMemberPromotedToOwner)).append("\n");
sb.append(" roomMemberDemotedFromOwner: ").append(toIndentedString(roomMemberDemotedFromOwner)).append("\n");
sb.append(" connectionRequested: ").append(toIndentedString(connectionRequested)).append("\n");
sb.append(" connectionAccepted: ").append(toIndentedString(connectionAccepted)).append("\n");
sb.append(" messageSuppressed: ").append(toIndentedString(messageSuppressed)).append("\n");
sb.append(" symphonyElementsAction: ").append(toIndentedString(symphonyElementsAction)).append("\n");
sb.append(" userRequestedToJoinRoom: ").append(toIndentedString(userRequestedToJoinRoom)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy