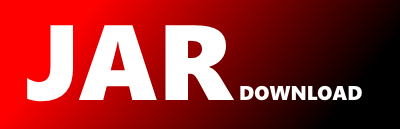
com.symphony.api.pod.DefaultApi Maven / Gradle / Ivy
package com.symphony.api.pod;
import com.symphony.api.model.Error;
import com.symphony.api.model.FileExtension;
import com.symphony.api.model.FileExtensionsResponse;
import com.symphony.api.model.MessageDetails;
import com.symphony.api.model.MessageIds;
import com.symphony.api.model.MessageIdsFromStream;
import com.symphony.api.model.MessageMetadataResponse;
import com.symphony.api.model.MessageReceiptDetailResponse;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.MediaType;
import org.apache.cxf.jaxrs.ext.multipart.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* Pod API
*
* This document refers to Symphony API calls that do not need encryption or decryption of content. - sessionToken can be obtained by calling the authenticationAPI on the symphony back end and the key manager respectively. Refer to the methods described in authenticatorAPI.yaml. - Actions are defined to be atomic, ie will succeed in their entirety or fail and have changed nothing. - If it returns a 40X status then it will have made no change to the system even if ome subset of the request would have succeeded. - If this contract cannot be met for any reason then this is an error and the response code will be 50X.
*
*/
@Path("/")
public interface DefaultApi {
/**
* Allows deletion of a specific file extension supported for upload
*
* Provides a method to delete a specific file extension configured for upload support via an admin. The file extension identifying the resource is treated case-insensitively by the API.
*
*/
@DELETE
@Path("/file_ext/v1/allowed_extensions/{extension}")
@Produces({ "application/json" })
@Operation(summary = "Allows deletion of a specific file extension supported for upload", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "204", description = "204 response"),
@ApiResponse(responseCode = "400", description = "Invalid arguments were passed by the client ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Authorization is not provided to this request ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Unexpected service error - a retry may work ", content = @Content(schema = @Schema(implementation = Error.class))) })
public void deleteAllowedFileExtension(@HeaderParam("sessionToken") String sessionToken, @PathParam("extension") String extension);
/**
* Allows iteration of all file extensions supported for upload
*
* Provides a RESTful API to iterate all file extensions configured by the tenant admin that are allowed for the upload. Pagination of this list is managed through a combination of the optional request parameters and service-side managed maximums. Pagination of the results is provided through the before or after input paramters and presented through the opaque cursor values provided as output from a previous response. Only one of before or after or neither may be provided. DO NOT store cursors. Cursors can quickly become invalid if items are added or deleted. Use them only during a short-period of time that you are traversing the list.
*
*/
@GET
@Path("/file_ext/v1/allowed_extensions")
@Produces({ "application/json" })
@Operation(summary = "Allows iteration of all file extensions supported for upload", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Requested sequence of file extensions object records with the page size limited by the optional limit paramter or the service-specific maximum limit offered. ", content = @Content(schema = @Schema(implementation = FileExtensionsResponse.class))),
@ApiResponse(responseCode = "400", description = "Invalid arguments were passed by the client ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Authentication was not provided ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Authorization is not provided to this request ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Unexpected service error - a retry may work ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "503", description = "Temporarily unable to handle request - could be due to service overload or maintenance "),
@ApiResponse(responseCode = "504", description = "Timeout waiting on response at gateway ") })
public FileExtensionsResponse listAllowedFileExtensions(@QueryParam("limit")Integer limit, @QueryParam("before")String before, @QueryParam("after")String after);
/**
* Allows replacement or creation of a specific file extension supported for upload
*
* Provides a method to create or replace a specific file extension configured for upload support via an admin. The API treats the file extension in the path case-insensitively by converting it to lowecase.
*
*/
@PUT
@Path("/file_ext/v1/allowed_extensions/{extension}")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Allows replacement or creation of a specific file extension supported for upload", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "200 response", content = @Content(schema = @Schema(implementation = FileExtension.class))),
@ApiResponse(responseCode = "400", description = "Invalid arguments were passed by the client: the file extension object specified the source as 'system' yet the file extension is not known to the system (API cannot create system file extensions, only customer-defined file extensions), the extension in the path doesn't match the extension in the body, the length of the file extension exceeded the maximum length (64 characters) ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Authorization is not provided to this request ", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Unexpected service error - a retry may work ", content = @Content(schema = @Schema(implementation = Error.class))) })
public FileExtension putAllowedFileExtension(FileExtension body, @HeaderParam("sessionToken") String sessionToken, @PathParam("extension") String extension);
/**
* Get the message metadata relationship
*
*/
@GET
@Path("/v1/admin/messages/{messageId}/metadata/relationships")
@Produces({ "application/json" })
@Operation(summary = "Get the message metadata relationship", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Successful Operation", content = @Content(schema = @Schema(implementation = MessageMetadataResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public MessageMetadataResponse v1AdminMessagesMessageIdMetadataRelationshipsGet(@HeaderParam("sessionToken") String sessionToken, @HeaderParam("User-Agent") String userAgent, @PathParam("messageId") String messageId);
/**
* Fetch receipts details from a specific message.
*
*/
@GET
@Path("/v1/admin/messages/{messageId}/receipts")
@Produces({ "application/json" })
@Operation(summary = "Fetch receipts details from a specific message.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = MessageReceiptDetailResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "404", description = "Not Found: Message receipt details cannot be found.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public MessageReceiptDetailResponse v1AdminMessagesMessageIdReceiptsGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("messageId") String messageId, @QueryParam("before")String before, @QueryParam("after")String after);
/**
* Fetch message details
*
*/
@POST
@Path("/v1/admin/messages")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Fetch message details", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = MessageDetails.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "404", description = "Not found: Message ID could not be found.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "422", description = "Unprocessable entity: Invalid message type.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public MessageDetails v1AdminMessagesPost(MessageIds body, @HeaderParam("sessionToken") String sessionToken);
/**
* Fetch message ids using timestamp.
*
* Gets all message Ids that matches a given stream defined by a streamId in a specified time frame
*
*/
@GET
@Path("/v2/admin/streams/{streamId}/messageIds")
@Produces({ "application/json" })
@Operation(summary = "Fetch message ids using timestamp.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = MessageIdsFromStream.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "404", description = "Not Found: Stream cannot be found.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "422", description = "Unprocessable Entity: Timestamp range is bigger than twenty-four hours.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public MessageIdsFromStream v2AdminStreamsStreamIdMessageIdsGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("streamId") String streamId, @QueryParam("since")Long since, @QueryParam("to")Long to, @QueryParam("limit")Integer limit, @QueryParam("offset")Integer offset);
}