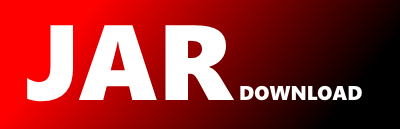
com.symphony.api.pod.MessageSuppressionApi Maven / Gradle / Ivy
package com.symphony.api.pod;
import com.symphony.api.model.Error;
import com.symphony.api.model.MessageSuppressionResponse;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.MediaType;
import org.apache.cxf.jaxrs.ext.multipart.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* Pod API
*
* This document refers to Symphony API calls that do not need encryption or decryption of content. - sessionToken can be obtained by calling the authenticationAPI on the symphony back end and the key manager respectively. Refer to the methods described in authenticatorAPI.yaml. - Actions are defined to be atomic, ie will succeed in their entirety or fail and have changed nothing. - If it returns a 40X status then it will have made no change to the system even if ome subset of the request would have succeeded. - If this contract cannot be met for any reason then this is an error and the response code will be 50X.
*
*/
@Path("/")
public interface MessageSuppressionApi {
/**
* Suppress a message
*
*/
@POST
@Path("/v1/admin/messagesuppression/{id}/suppress")
@Produces({ "application/json" })
@Operation(summary = "Suppress a message", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = MessageSuppressionResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public MessageSuppressionResponse v1AdminMessagesuppressionIdSuppressPost(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken);
}