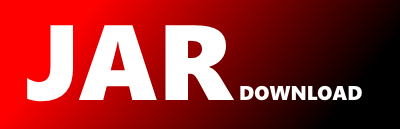
com.symphony.api.pod.StreamsApi Maven / Gradle / Ivy
package com.symphony.api.pod;
import com.symphony.api.model.AdminStreamFilter;
import com.symphony.api.model.AdminStreamList;
import com.symphony.api.model.Error;
import com.symphony.api.model.RoomAttributes;
import com.symphony.api.model.RoomCreate;
import com.symphony.api.model.RoomDetail;
import com.symphony.api.model.RoomSearchCriteria;
import com.symphony.api.model.RoomSearchResults;
import com.symphony.api.model.Stream;
import com.symphony.api.model.StreamAttachmentResponse;
import com.symphony.api.model.StreamAttributes;
import com.symphony.api.model.StreamFilter;
import com.symphony.api.model.StreamList;
import com.symphony.api.model.V1IMAttributes;
import com.symphony.api.model.V1IMDetail;
import com.symphony.api.model.V2AdminStreamFilter;
import com.symphony.api.model.V2AdminStreamList;
import com.symphony.api.model.V2MembershipList;
import com.symphony.api.model.V2RoomAttributes;
import com.symphony.api.model.V2RoomDetail;
import com.symphony.api.model.V2RoomSearchCriteria;
import com.symphony.api.model.V2StreamAttributes;
import com.symphony.api.model.V3RoomAttributes;
import com.symphony.api.model.V3RoomDetail;
import com.symphony.api.model.V3RoomSearchResults;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.MediaType;
import org.apache.cxf.jaxrs.ext.multipart.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* Pod API
*
* This document refers to Symphony API calls that do not need encryption or decryption of content. - sessionToken can be obtained by calling the authenticationAPI on the symphony back end and the key manager respectively. Refer to the methods described in authenticatorAPI.yaml. - Actions are defined to be atomic, ie will succeed in their entirety or fail and have changed nothing. - If it returns a 40X status then it will have made no change to the system even if ome subset of the request would have succeeded. - If this contract cannot be met for any reason then this is an error and the response code will be 50X.
*
*/
@Path("/")
public interface StreamsApi {
/**
* Create a new single or multi party instant message conversation
*
* At least two user IDs must be provided or an error response will be sent. The caller is not included in the members of the created chat. Duplicate users will be included in the membership of the chat but the duplication will be silently ignored. If there is an existing IM conversation with the same set of participants then the id of that existing stream will be returned.
*
*/
@POST
@Path("/v1/admin/im/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new single or multi party instant message conversation", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = Stream.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public Stream v1AdminImCreatePost(List body, @HeaderParam("sessionToken") String sessionToken);
/**
* Deactivate or reactivate a chatroom via AC Portal.
*
*/
@POST
@Path("/v1/admin/room/{id}/setActive")
@Produces({ "application/json" })
@Operation(summary = "Deactivate or reactivate a chatroom via AC Portal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomDetail v1AdminRoomIdSetActivePost(@PathParam("id") String id, @QueryParam("active")Boolean active, @HeaderParam("sessionToken") String sessionToken);
/**
* List the current members of an existing stream. The stream can be of type IM, MIM, or ROOM
*
*/
@GET
@Path("/v1/admin/stream/{id}/membership/list")
@Produces({ "application/json" })
@Operation(summary = "List the current members of an existing stream. The stream can be of type IM, MIM, or ROOM", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2MembershipList.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2MembershipList v1AdminStreamIdMembershipListGet(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Retrieve all the streams across the enterprise where the membership of the stream has been modified between a given time range
*
*/
@POST
@Path("/v1/admin/streams/list")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Retrieve all the streams across the enterprise where the membership of the stream has been modified between a given time range ", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = AdminStreamList.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public AdminStreamList v1AdminStreamsListPost(@HeaderParam("sessionToken") String sessionToken, AdminStreamFilter body, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Create a new single or multi party instant message conversation between the caller and specified users.
*
* At least one user ID must be provided or an error response will be sent. The caller is implicitly included in the members of the created chat. Duplicate users will be included in the membership of the chat but the duplication will be silently ignored. If there is an existing IM conversation with the same set of participants then the id of that existing stream will be returned. This method was incorrectly specified to take a query parameter in version 1.0 of this specification but now expects a JSON array of user IDs in the body of the request.
*
*/
@POST
@Path("/v1/im/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new single or multi party instant message conversation between the caller and specified users.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = Stream.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public Stream v1ImCreatePost(List body, @HeaderParam("sessionToken") String sessionToken);
/**
* Get information about a partcular IM.
*
*/
@GET
@Path("/v1/im/{id}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular IM.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V1IMDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V1IMDetail v1ImIdInfoGet(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken);
/**
* Update the attributes of an existing IM.
*
*/
@POST
@Path("/v1/im/{id}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the attributes of an existing IM.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V1IMDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "451", description = "Unavailable for Legal Reasons: Compliance Issues found in IM update request.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V1IMDetail v1ImIdUpdatePost(V1IMAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id);
/**
* Create a new chatroom.
*
* Create a new chatroom. If no attributes are specified, the room is created as a private chatroom.
*
*/
@POST
@Path("/v1/room/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomDetail v1RoomCreatePost(RoomCreate body, @HeaderParam("sessionToken") String sessionToken);
/**
* Get information about a partcular chatroom.
*
*/
@GET
@Path("/v1/room/{id}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomDetail v1RoomIdInfoGet(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken);
/**
* Deactivate or reactivate a chatroom. At creation, a new chatroom is active.
*
*/
@POST
@Path("/v1/room/{id}/setActive")
@Produces({ "application/json" })
@Operation(summary = "Deactivate or reactivate a chatroom. At creation, a new chatroom is active.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomDetail v1RoomIdSetActivePost(@PathParam("id") String id, @QueryParam("active")Boolean active, @HeaderParam("sessionToken") String sessionToken);
/**
* Update the attributes of an existing chatroom.
*
*/
@POST
@Path("/v1/room/{id}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the attributes of an existing chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomDetail v1RoomIdUpdatePost(RoomAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id);
/**
* Retrieve a list of all streams of which the requesting user is a member, sorted by creation date (ascending).
*
*/
@POST
@Path("/v1/streams/list")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Retrieve a list of all streams of which the requesting user is a member, sorted by creation date (ascending). ", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = StreamList.class))),
@ApiResponse(responseCode = "204", description = "Stream not found."),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public StreamList v1StreamsListPost(@HeaderParam("sessionToken") String sessionToken, StreamFilter body, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Get attachments in a particular stream.
*
*/
@GET
@Path("/v1/streams/{sid}/attachments")
@Produces({ "application/json" })
@Operation(summary = "Get attachments in a particular stream.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = StreamAttachmentResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public StreamAttachmentResponse v1StreamsSidAttachmentsGet(@PathParam("sid") String sid, @HeaderParam("sessionToken") String sessionToken, @QueryParam("since")Long since, @QueryParam("to")Long to, @QueryParam("limit")Integer limit, @QueryParam("sortDir")String sortDir);
/**
* Get information about a partcular stream.
*
*/
@GET
@Path("/v1/streams/{sid}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular stream.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = StreamAttributes.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public StreamAttributes v1StreamsSidInfoGet(@PathParam("sid") String sid, @HeaderParam("sessionToken") String sessionToken);
/**
* Retrieve all the streams across the enterprise where the membership of the stream has been modified between a given time range
*
*/
@POST
@Path("/v2/admin/streams/list")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Retrieve all the streams across the enterprise where the membership of the stream has been modified between a given time range ", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2AdminStreamList.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2AdminStreamList v2AdminStreamsListPost(@HeaderParam("sessionToken") String sessionToken, V2AdminStreamFilter body, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Create a new chatroom.
*
* Create a new chatroom. If no attributes are specified, the room is created as a private chatroom.
*
*/
@POST
@Path("/v2/room/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2RoomDetail v2RoomCreatePost(V2RoomAttributes body, @HeaderParam("sessionToken") String sessionToken);
/**
* Get information about a partcular chatroom.
*
*/
@GET
@Path("/v2/room/{id}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2RoomDetail v2RoomIdInfoGet(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken);
/**
* Update the attributes of an existing chatroom.
*
*/
@POST
@Path("/v2/room/{id}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the attributes of an existing chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2RoomDetail v2RoomIdUpdatePost(V2RoomAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id);
/**
* Search rooms according to the specified criteria.
*
*/
@POST
@Path("/v2/room/search")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Search rooms according to the specified criteria.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = RoomSearchResults.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoomSearchResults v2RoomSearchPost(RoomSearchCriteria body, @HeaderParam("sessionToken") String sessionToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Get information about a partcular stream.
*
*/
@GET
@Path("/v2/streams/{sid}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular stream.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V2StreamAttributes.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2StreamAttributes v2StreamsSidInfoGet(@PathParam("sid") String sid, @HeaderParam("sessionToken") String sessionToken);
/**
* Create a new chatroom.
*
* Create a new chatroom. If no attributes are specified, the room is created as a private chatroom.
*
*/
@POST
@Path("/v3/room/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V3RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "451", description = "Unavailable for Legal Reasons: Compliance Issues found in room creation request.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V3RoomDetail v3RoomCreatePost(V3RoomAttributes body, @HeaderParam("sessionToken") String sessionToken);
/**
* Get information about a partcular chatroom.
*
*/
@GET
@Path("/v3/room/{id}/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about a partcular chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V3RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V3RoomDetail v3RoomIdInfoGet(@PathParam("id") String id, @HeaderParam("sessionToken") String sessionToken);
/**
* Update the attributes of an existing chatroom.
*
*/
@POST
@Path("/v3/room/{id}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the attributes of an existing chatroom.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V3RoomDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "451", description = "Unavailable for Legal Reasons: Compliance Issues found in room update request.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V3RoomDetail v3RoomIdUpdatePost(V3RoomAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id);
/**
* Search rooms according to the specified criteria.
*
*/
@POST
@Path("/v3/room/search")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Search rooms according to the specified criteria.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "OK", content = @Content(schema = @Schema(implementation = V3RoomSearchResults.class))),
@ApiResponse(responseCode = "400", description = "Client error.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V3RoomSearchResults v3RoomSearchPost(V2RoomSearchCriteria body, @HeaderParam("sessionToken") String sessionToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
}