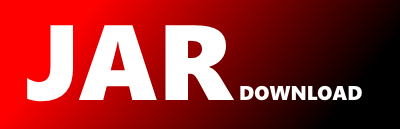
com.symphony.api.pod.UserApi Maven / Gradle / Ivy
package com.symphony.api.pod;
import com.symphony.api.model.AvatarList;
import com.symphony.api.model.AvatarUpdate;
import com.symphony.api.model.DelegateAction;
import com.symphony.api.model.Disclaimer;
import com.symphony.api.model.Error;
import com.symphony.api.model.Feature;
import com.symphony.api.model.FeatureList;
import com.symphony.api.model.FollowersList;
import com.symphony.api.model.FollowersListResponse;
import com.symphony.api.model.FollowingListResponse;
import com.symphony.api.model.IntegerList;
import com.symphony.api.model.RoleDetailList;
import com.symphony.api.model.StringId;
import com.symphony.api.model.SuccessResponse;
import com.symphony.api.model.UserAttributes;
import com.symphony.api.model.UserCreate;
import com.symphony.api.model.UserDetail;
import com.symphony.api.model.UserDetailList;
import com.symphony.api.model.UserFilter;
import com.symphony.api.model.UserIdList;
import com.symphony.api.model.UserStatus;
import com.symphony.api.model.UserSuspension;
import com.symphony.api.model.V2UserAttributes;
import com.symphony.api.model.V2UserCreate;
import com.symphony.api.model.V2UserDetail;
import com.symphony.api.model.V2UserDetailList;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.MediaType;
import org.apache.cxf.jaxrs.ext.multipart.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* Pod API
*
* This document refers to Symphony API calls that do not need encryption or decryption of content. - sessionToken can be obtained by calling the authenticationAPI on the symphony back end and the key manager respectively. Refer to the methods described in authenticatorAPI.yaml. - Actions are defined to be atomic, ie will succeed in their entirety or fail and have changed nothing. - If it returns a 40X status then it will have made no change to the system even if ome subset of the request would have succeeded. - If this contract cannot be met for any reason then this is an error and the response code will be 50X.
*
*/
@Path("/")
public interface UserApi {
/**
* Get a list of all roles available in the company (pod)
*
*/
@GET
@Path("/v1/admin/system/roles/list")
@Produces({ "application/json" })
@Operation(summary = "Get a list of all roles available in the company (pod)", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = RoleDetailList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public RoleDetailList v1AdminSystemRolesListGet(@HeaderParam("sessionToken") String sessionToken);
/**
* Create a new user
*
*/
@POST
@Path("/v1/admin/user/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserDetail v1AdminUserCreatePost(UserCreate body, @HeaderParam("sessionToken") String sessionToken);
/**
* Find a user based on attributes
*
*/
@POST
@Path("/v1/admin/user/find")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Find a user based on attributes", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserDetailList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserDetailList v1AdminUserFindPost(UserFilter body, @HeaderParam("sessionToken") String sessionToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Retreive a list of all users in the company (pod)
*
*/
@GET
@Path("/v1/admin/user/list")
@Produces({ "application/json" })
@Operation(summary = "Retreive a list of all users in the company (pod)", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserIdList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserIdList v1AdminUserListGet(@HeaderParam("sessionToken") String sessionToken);
/**
* Get the URL of the avatar of a particular user
*
*/
@GET
@Path("/v1/admin/user/{uid}/avatar")
@Produces({ "application/json" })
@Operation(summary = "Get the URL of the avatar of a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = AvatarList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public AvatarList v1AdminUserUidAvatarGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update the avatar of a particular user
*
*/
@POST
@Path("/v1/admin/user/{uid}/avatar/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the avatar of a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidAvatarUpdatePost(AvatarUpdate body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Get the delegates assigned to a user
*
*/
@GET
@Path("/v1/admin/user/{uid}/delegates")
@Produces({ "application/json" })
@Operation(summary = "Get the delegates assigned to a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "The userid's of the delegates of this user.", content = @Content(schema = @Schema(implementation = IntegerList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public IntegerList v1AdminUserUidDelegatesGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update the delegates assigned to a user
*
*/
@POST
@Path("/v1/admin/user/{uid}/delegates/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the delegates assigned to a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Sucesss.", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidDelegatesUpdatePost(DelegateAction body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Unassign a disclaimer from a user
*
*/
@DELETE
@Path("/v1/admin/user/{uid}/disclaimer")
@Produces({ "application/json" })
@Operation(summary = "Unassign a disclaimer from a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidDisclaimerDelete(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Get the disclaimer assigned to a user
*
*/
@GET
@Path("/v1/admin/user/{uid}/disclaimer")
@Produces({ "application/json" })
@Operation(summary = "Get the disclaimer assigned to a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = Disclaimer.class))),
@ApiResponse(responseCode = "204", description = "No content. User doesn't have an assigned disclaimer"),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public Disclaimer v1AdminUserUidDisclaimerGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Assign a disclaimer to a user
*
*/
@POST
@Path("/v1/admin/user/{uid}/disclaimer/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Assign a disclaimer to a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidDisclaimerUpdatePost(StringId body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Get the list of Symphony feature entitlements enabled for a particular user
*
*/
@GET
@Path("/v1/admin/user/{uid}/features")
@Produces({ "application/json" })
@Operation(summary = "Get the list of Symphony feature entitlements enabled for a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = FeatureList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public FeatureList v1AdminUserUidFeaturesGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update the list of Symphony feature entitlements for a particular user
*
*/
@POST
@Path("/v1/admin/user/{uid}/features/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the list of Symphony feature entitlements for a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidFeaturesUpdatePost(List body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Retreive user details for a particular user
*
*/
@GET
@Path("/v1/admin/user/{uid}")
@Produces({ "application/json" })
@Operation(summary = "Retreive user details for a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserDetail v1AdminUserUidGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Add a role to a user
*
*/
@POST
@Path("/v1/admin/user/{uid}/roles/add")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Add a role to a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidRolesAddPost(StringId body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Remove a role from a user
*
*/
@POST
@Path("/v1/admin/user/{uid}/roles/remove")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Remove a role from a user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "404", description = "Not Found: User cannot be found", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidRolesRemovePost(StringId body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Get the status, active or inactive, for a particular user
*
*/
@GET
@Path("/v1/admin/user/{uid}/status")
@Produces({ "application/json" })
@Operation(summary = "Get the status, active or inactive, for a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserStatus.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserStatus v1AdminUserUidStatusGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update the status of a particular user
*
*/
@POST
@Path("/v1/admin/user/{uid}/status/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the status of a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUidStatusUpdatePost(UserStatus body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update an existing user
*
*/
@POST
@Path("/v1/admin/user/{uid}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update an existing user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public UserDetail v1AdminUserUidUpdatePost(UserAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update the status of suspension of a particular user
*
*/
@PUT
@Path("/v1/admin/user/{userId}/suspension/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update the status of suspension of a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1AdminUserUserIdSuspensionUpdatePut(UserSuspension body, @HeaderParam("sessionToken") String sessionToken, @PathParam("userId") Long userId);
/**
* Make a list of users start following a specific user
*
*/
@POST
@Path("/v1/user/{uid}/follow")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Make a list of users start following a specific user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1UserUidFollowPost(FollowersList body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Returns the list of followers for a specific user
*
*/
@GET
@Path("/v1/user/{uid}/followers")
@Produces({ "application/json" })
@Operation(summary = "Returns the list of followers for a specific user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = FollowersListResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public FollowersListResponse v1UserUidFollowersGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid, @QueryParam("limit")Integer limit, @QueryParam("before")String before, @QueryParam("after")String after);
/**
* Returns the list of users that a specific user is following
*
*/
@GET
@Path("/v1/user/{uid}/following")
@Produces({ "application/json" })
@Operation(summary = "Returns the list of users that a specific user is following", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = FollowingListResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public FollowingListResponse v1UserUidFollowingGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid, @QueryParam("limit")Integer limit, @QueryParam("before")String before, @QueryParam("after")String after);
/**
* Make a list of users unfollowing a specific user
*
*/
@POST
@Path("/v1/user/{uid}/unfollow")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Make a list of users unfollowing a specific user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public SuccessResponse v1UserUidUnfollowPost(FollowersList body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Create a new V2 User
*
*/
@POST
@Path("/v2/admin/user/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a new V2 User", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = V2UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2UserDetail v2AdminUserCreatePost(V2UserCreate body, @HeaderParam("sessionToken") String sessionToken);
/**
* Retrieve a list of all users in the company (pod)
*
*/
@GET
@Path("/v2/admin/user/list")
@Produces({ "application/json" })
@Operation(summary = "Retrieve a list of all users in the company (pod)", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = V2UserDetailList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2UserDetailList v2AdminUserListGet(@HeaderParam("sessionToken") String sessionToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
/**
* Retreive V2 User details for a particular user
*
*/
@GET
@Path("/v2/admin/user/{uid}")
@Produces({ "application/json" })
@Operation(summary = "Retreive V2 User details for a particular user", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = V2UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2UserDetail v2AdminUserUidGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
/**
* Update an existing V2 User
*
*/
@POST
@Path("/v2/admin/user/{uid}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update an existing V2 User", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Success", content = @Content(schema = @Schema(implementation = V2UserDetail.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = Error.class))) })
public V2UserDetail v2AdminUserUidUpdatePost(V2UserAttributes body, @HeaderParam("sessionToken") String sessionToken, @PathParam("uid") Long uid);
}