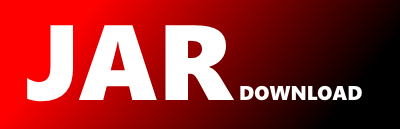
org.firebirdsql.gds.impl.wire.ParameterBufferBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaybird-jdk15 Show documentation
Show all versions of jaybird-jdk15 Show documentation
JDBC Driver for the Firebird RDBMS
/*
* Firebird Open Source J2ee connector - jdbc driver
*
* Distributable under LGPL license.
* You may obtain a copy of the License at http://www.gnu.org/copyleft/lgpl.html
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* LGPL License for more details.
*
* This file was created by members of the firebird development team.
* All individual contributions remain the Copyright (C) of those
* individuals. Contributors to this file are either listed here or
* can be obtained from a CVS history command.
*
* All rights reserved.
*/
package org.firebirdsql.gds.impl.wire;
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
import java.io.IOException;
/**
* Base class for BlobParameterBufferImp and DatabaseParameterBufferImp and perhaps eventualy TransactionParameterBuffer.
*/
public class ParameterBufferBase implements java.io.Serializable, Xdrable
{
// Parameter Buffer Implementation
public void addArgument(int argumentType, String value)
{
getArgumentsList().add(new StringArgument(argumentType, value ));
}
public void addArgument(int argumentType, int value)
{
getArgumentsList().add(new NumericArgument(argumentType, value));
}
public void addArgument(int argumentType)
{
getArgumentsList().add(new SingleItem(argumentType));
}
public void addArgument(int type, byte[] content)
{
getArgumentsList().add(new ByteArrayArgument(type, content));
}
public String getArgumentAsString(int type)
{
final List argumentsList = getArgumentsList();
for( int i = 0, n = argumentsList.size(); i>8);
outputStream.write(value>>16);
outputStream.write(value>>24);
}
int getType()
{
return type;
}
public int hashCode()
{
return type;
}
public boolean equals(Object other)
{
if( other == null || other instanceof NumericArgument == false )
return false;
final NumericArgument otherNumericArgument = (NumericArgument)other;
return type == otherNumericArgument.type &&
value == otherNumericArgument.value;
}
private final int type;
private final int value;
}
//---------------------------------------------------------------------------
// ByteArrayArgument
//---------------------------------------------------------------------------
private static final class ByteArrayArgument extends Argument
{
ByteArrayArgument( int type, byte[] value )
{
this.type = type;
this.value = value;
}
void writeTo(XdrOutputStream outputStream) throws IOException
{
outputStream.write(type);
final int valueLength = value.length;
writeLength(valueLength, outputStream);
for(int i = 0; i 1");
}
protected void writeLength(int length, XdrOutputStream outputStream) throws IOException
{
outputStream.write(length);
}
int getType()
{
return type;
}
public int hashCode()
{
return type;
}
public boolean equals(Object other)
{
if( other == null || other instanceof ByteArrayArgument == false )
return false;
final ByteArrayArgument otherByteArrayArgument = (ByteArrayArgument)other;
return type == otherByteArrayArgument.type &&
Arrays.equals(value , otherByteArrayArgument.value);
}
private final int type;
private final byte[] value;
}
//---------------------------------------------------------------------------
// SingleItem
//---------------------------------------------------------------------------
private static final class SingleItem extends Argument
{
SingleItem( int item )
{
this.item = item;
}
void writeTo(XdrOutputStream outputStream) throws IOException
{
outputStream.write(item);
}
int getLength()
{
return 1;
}
int getType()
{
return item;
}
public int hashCode()
{
return item;
}
public boolean equals(Object other)
{
if( other == null || other instanceof SingleItem == false )
return false;
final SingleItem otherSingleItem = (SingleItem)other;
return item == otherSingleItem.item;
}
private final int item;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy