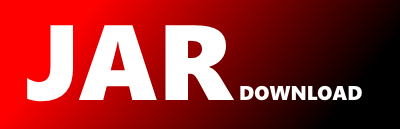
org.firebirdsql.jdbc.FBCachedFetcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaybird-jdk15 Show documentation
Show all versions of jaybird-jdk15 Show documentation
JDBC Driver for the Firebird RDBMS
/*
* $Id: FBCachedFetcher.java 60425 2014-12-24 11:02:41Z mrotteveel $
*
* Firebird Open Source JavaEE Connector - JDBC Driver
*
* Distributable under LGPL license.
* You may obtain a copy of the License at http://www.gnu.org/copyleft/lgpl.html
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* LGPL License for more details.
*
* This file was created by members of the firebird development team.
* All individual contributions remain the Copyright (C) of those
* individuals. Contributors to this file are either listed here or
* can be obtained from a source control history command.
*
* All rights reserved.
*/
package org.firebirdsql.jdbc;
import java.sql.*;
import java.util.ArrayList;
import org.firebirdsql.gds.GDSException;
import org.firebirdsql.gds.XSQLVAR;
import org.firebirdsql.gds.impl.AbstractIscStmtHandle;
import org.firebirdsql.gds.impl.GDSHelper;
import org.firebirdsql.jdbc.field.*;
class FBCachedFetcher implements FBFetcher {
private final boolean forwardOnly;
private Object[] rowsArray;
private int rowNum = 0;
private int fetchSize;
private final FBObjectListener.FetcherListener fetcherListener;
FBCachedFetcher(GDSHelper gdsHelper, int fetchSize, int maxRows, AbstractIscStmtHandle stmt_handle,
FBObjectListener.FetcherListener fetcherListener, boolean forwardOnly) throws SQLException {
this.fetcherListener = fetcherListener;
this.forwardOnly = forwardOnly;
final ArrayList rowsSets = new ArrayList(100);
final XSQLVAR[] xsqlvars = stmt_handle.getOutSqlda().sqlvar;
// Check if there is blobs to catch
final boolean[] isBlob = new boolean[xsqlvars.length];
boolean hasBlobs = false;
for (int i = 0; i < xsqlvars.length; i++){
isBlob[i] = FBField.isType(xsqlvars[i], Types.BLOB) ||
FBField.isType(xsqlvars[i], Types.BINARY) ||
FBField.isType(xsqlvars[i], Types.LONGVARCHAR);
if (isBlob[i])
hasBlobs = true;
}
// load all rows from statement
int rowsCount = 0;
try {
if (fetchSize == 0)
fetchSize = MAX_FETCH_ROWS;
this.fetchSize = fetchSize;
// the following if, is only for callable statement
if (!stmt_handle.isAllRowsFetched() && stmt_handle.size() == 0) {
do {
if (maxRows != 0 && fetchSize > maxRows - rowsCount)
fetchSize = maxRows - rowsCount;
gdsHelper.fetch(stmt_handle, fetchSize);
final int fetchedRowCount = stmt_handle.size();
if (fetchedRowCount > 0){
byte[][][] rows = stmt_handle.getRows();
// Copy of right length when less rows fetched than requested
if (rows.length > fetchedRowCount) {
final byte[][][] tempRows = new byte[fetchedRowCount][][];
System.arraycopy(rows, 0, tempRows, 0, fetchedRowCount);
rows = tempRows;
}
rowsSets.add(rows);
rowsCount += fetchedRowCount;
stmt_handle.removeRows();
}
} while (!stmt_handle.isAllRowsFetched() && (maxRows == 0 || rowsCount rowsArray.length;
}
public void deleteRow() throws SQLException {
final Object[] newRows = new Object[rowsArray.length - 1];
System.arraycopy(rowsArray, 0, newRows, 0, rowNum - 1);
if (rowNum < rowsArray.length)
System.arraycopy(rowsArray, rowNum, newRows, rowNum - 1, rowsArray.length - rowNum);
rowsArray = newRows;
if (isAfterLast())
fetcherListener.rowChanged(this, null);
else
if (isBeforeFirst())
fetcherListener.rowChanged(this, null);
else
fetcherListener.rowChanged(this, (byte[][])rowsArray[rowNum-1]);
}
public void insertRow(byte[][] data) throws SQLException {
final Object[] newRows = new Object[rowsArray.length + 1];
if (rowNum == 0)
rowNum++;
System.arraycopy(rowsArray, 0, newRows, 0, rowNum - 1);
System.arraycopy(rowsArray, rowNum - 1, newRows, rowNum, rowsArray.length - rowNum + 1);
newRows[rowNum - 1] = data;
rowsArray = newRows;
if (isAfterLast() || isBeforeFirst())
fetcherListener.rowChanged(this, null);
else
fetcherListener.rowChanged(this, (byte[][])rowsArray[rowNum-1]);
}
public void updateRow(byte[][] data) throws SQLException {
if (!isAfterLast() && !isBeforeFirst()) {
rowsArray[rowNum - 1] = data;
fetcherListener.rowChanged(this, data);
}
}
public int getFetchSize(){
return fetchSize;
}
public void setFetchSize(int fetchSize){
this.fetchSize = fetchSize;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy