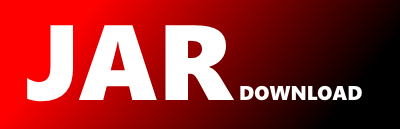
org.firebirdsql.jdbc.FBDatabaseMetaData Maven / Gradle / Ivy
Show all versions of jaybird Show documentation
package org.firebirdsql.jdbc;
import java.sql.ResultSet;
import java.sql.RowIdLifetime;
import java.sql.SQLException;
import java.util.ArrayList;
import org.firebirdsql.gds.GDSException;
import org.firebirdsql.gds.ISCConstants;
import org.firebirdsql.gds.XSQLVAR;
import org.firebirdsql.gds.impl.GDSHelper;
public class FBDatabaseMetaData extends AbstractDatabaseMetaData {
public FBDatabaseMetaData(AbstractConnection c) throws GDSException {
super(c);
// TODO Auto-generated constructor stub
}
public FBDatabaseMetaData(GDSHelper gdsHelper) {
super(gdsHelper);
// TODO Auto-generated constructor stub
}
//-------------------------- JDBC 4.0 -------------------------------------
public boolean supportsStoredFunctionsUsingCallSyntax() throws SQLException {
return false;
}
public boolean autoCommitFailureClosesAllResultSets() throws SQLException {
// the holdable result sets remain open, others are closed, but this
// happens before the statement is executed
return false;
}
/**
* Retrieves a list of the client info properties
* that the driver supports. The result set contains the following columns
*
*
* - NAME String=> The name of the client info property
* - MAX_LEN int=> The maximum length of the value for the property
* - DEFAULT_VALUE String=> The default value of the property
* - DESCRIPTION String=> A description of the property. This will typically
* contain information as to where this property is
* stored in the database.
*
*
* The ResultSet
is sorted by the NAME column
*
* @return A ResultSet
object; each row is a supported client info
* property
*
* @exception SQLException if a database access error occurs
*
* @since 1.6
*/
public ResultSet getClientInfoProperties() throws SQLException {
XSQLVAR[] xsqlvars = new XSQLVAR[4];
xsqlvars[0] = new XSQLVAR();
xsqlvars[0].sqltype = ISCConstants.SQL_VARYING;
xsqlvars[0].sqllen = 31;
xsqlvars[0].sqlname = "NAME";
xsqlvars[0].relname = "UDT";
xsqlvars[1] = new XSQLVAR();
xsqlvars[1].sqltype = ISCConstants.SQL_LONG;
xsqlvars[1].sqllen = 4;
xsqlvars[1].sqlname = "MAX_LEN";
xsqlvars[1].relname = "UDT";
xsqlvars[2] = new XSQLVAR();
xsqlvars[2].sqltype = ISCConstants.SQL_VARYING;
xsqlvars[2].sqllen = 31;
xsqlvars[2].sqlname = "DEFAULT";
xsqlvars[2].relname = "UDT";
xsqlvars[3] = new XSQLVAR();
xsqlvars[3].sqltype = ISCConstants.SQL_VARYING;
xsqlvars[3].sqllen = 31;
xsqlvars[3].sqlname = "DESCRIPTION";
xsqlvars[3].relname = "UDT";
ArrayList rows = new ArrayList(0);
return new FBResultSet(xsqlvars, rows);
}
/**
* Retrieves a description of the given catalog's system or user
* function parameters and return type.
*
*
Only descriptions matching the schema, function and
* parameter name criteria are returned. They are ordered by
* FUNCTION_CAT
, FUNCTION_SCHEM
,
* FUNCTION_NAME
and
* SPECIFIC_ NAME
. Within this, the return value,
* if any, is first. Next are the parameter descriptions in call
* order. The column descriptions follow in column number order.
*
*
Each row in the ResultSet
* is a parameter description, column description or
* return type description with the following fields:
*
* - FUNCTION_CAT String => function catalog (may be
null
)
* - FUNCTION_SCHEM String => function schema (may be
null
)
* - FUNCTION_NAME String => function name. This is the name
* used to invoke the function
*
- COLUMN_NAME String => column/parameter name
*
- COLUMN_TYPE Short => kind of column/parameter:
*
* - functionColumnUnknown - nobody knows
*
- functionColumnIn - IN parameter
*
- functionColumnInOut - INOUT parameter
*
- functionColumnOut - OUT parameter
*
- functionColumnReturn - function return value
*
- functionColumnResult - Indicates that the parameter or column
* is a column in the
ResultSet
*
* - DATA_TYPE int => SQL type from java.sql.Types
*
- TYPE_NAME String => SQL type name, for a UDT type the
* type name is fully qualified
*
- PRECISION int => precision
*
- LENGTH int => length in bytes of data
*
- SCALE short => scale - null is returned for data types where
* SCALE is not applicable.
*
- RADIX short => radix
*
- NULLABLE short => can it contain NULL.
*
* - functionNoNulls - does not allow NULL values
*
- functionNullable - allows NULL values
*
- functionNullableUnknown - nullability unknown
*
* - REMARKS String => comment describing column/parameter
*
- CHAR_OCTET_LENGTH int => the maximum length of binary
* and character based parameters or columns. For any other datatype the returned value
* is a NULL
*
- ORDINAL_POSITION int => the ordinal position, starting
* from 1, for the input and output parameters. A value of 0
* is returned if this row describes the function's return value.
* For result set columns, it is the
* ordinal position of the column in the result set starting from 1.
*
- IS_NULLABLE String => ISO rules are used to determine
* the nullability for a parameter or column.
*
* - YES --- if the parameter or column can include NULLs
*
- NO --- if the parameter or column cannot include NULLs
*
- empty string --- if the nullability for the
* parameter or column is unknown
*
* - SPECIFIC_NAME String => the name which uniquely identifies
* this function within its schema. This is a user specified, or DBMS
* generated, name that may be different then the
FUNCTION_NAME
* for example with overload functions
*
*
* The PRECISION column represents the specified column size for the given
* parameter or column.
* For numeric data, this is the maximum precision. For character data, this is the length in characters.
* For datetime datatypes, this is the length in characters of the String representation (assuming the
* maximum allowed precision of the fractional seconds component). For binary data, this is the length in bytes. For the ROWID datatype,
* this is the length in bytes. Null is returned for data types where the
* column size is not applicable.
* @param catalog a catalog name; must match the catalog name as it
* is stored in the database; "" retrieves those without a catalog;
* null
means that the catalog name should not be used to narrow
* the search
* @param schemaPattern a schema name pattern; must match the schema name
* as it is stored in the database; "" retrieves those without a schema;
* null
means that the schema name should not be used to narrow
* the search
* @param functionNamePattern a procedure name pattern; must match the
* function name as it is stored in the database
* @param columnNamePattern a parameter name pattern; must match the
* parameter or column name as it is stored in the database
* @return ResultSet
- each row describes a
* user function parameter, column or return type
*
* @exception SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getFunctionColumns(String catalog, String schemaPattern, String functionNamePattern, String columnNamePattern) throws SQLException {
// FIXME implement this method
throw new FBDriverNotCapableException();
}
/**
* Retrieves a description of the system and user functions available
* in the given catalog.
*
* Only system and user function descriptions matching the schema and
* function name criteria are returned. They are ordered by
* FUNCTION_CAT
, FUNCTION_SCHEM
,
* FUNCTION_NAME
and
* SPECIFIC_ NAME
.
*
*
Each function description has the the following columns:
*
* - FUNCTION_CAT String => function catalog (may be
null
)
* - FUNCTION_SCHEM String => function schema (may be
null
)
* - FUNCTION_NAME String => function name. This is the name
* used to invoke the function
*
- REMARKS String => explanatory comment on the function
*
- FUNCTION_TYPE short => kind of function:
*
* - functionResultUnknown - Cannot determine if a return value
* or table will be returned
*
- functionNoTable- Does not return a table
*
- functionReturnsTable - Returns a table
*
* - SPECIFIC_NAME String => the name which uniquely identifies
* this function within its schema. This is a user specified, or DBMS
* generated, name that may be different then the
FUNCTION_NAME
* for example with overload functions
*
*
* A user may not have permission to execute any of the functions that are
* returned by getFunctions
*
* @param catalog a catalog name; must match the catalog name as it
* is stored in the database; "" retrieves those without a catalog;
* null
means that the catalog name should not be used to narrow
* the search
* @param schemaPattern a schema name pattern; must match the schema name
* as it is stored in the database; "" retrieves those without a schema;
* null
means that the schema name should not be used to narrow
* the search
* @param functionNamePattern a function name pattern; must match the
* function name as it is stored in the database
* @return ResultSet
- each row is a function description
* @exception SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getFunctions(String catalog, String schemaPattern, String functionNamePattern) throws SQLException {
throw new FBDriverNotCapableException();
}
/**
* Indicates whether or not this data source supports the SQL ROWID
type,
* and if so the lifetime for which a RowId
object remains valid.
*
* The returned int values have the following relationship:
*
* ROWID_UNSUPPORTED < ROWID_VALID_OTHER < ROWID_VALID_TRANSACTION
* < ROWID_VALID_SESSION < ROWID_VALID_FOREVER
*
* so conditional logic such as
*
* if (metadata.getRowIdLifetime() > DatabaseMetaData.ROWID_VALID_TRANSACTION)
*
* can be used. Valid Forever means valid across all Sessions, and valid for
* a Session means valid across all its contained Transactions.
*
* @return the status indicating the lifetime of a RowId
* @throws SQLException if a database access error occurs
* @since 1.6
*/
public RowIdLifetime getRowIdLifetime() throws SQLException {
throw new FBDriverNotCapableException();
}
/**
* Retrieves the schema names available in this database. The results
* are ordered by TABLE_CATALOG
and
* TABLE_SCHEM
.
*
* The schema columns are:
*
* - TABLE_SCHEM String => schema name
*
- TABLE_CATALOG String => catalog name (may be
null
)
*
*
*
* @param catalog a catalog name; must match the catalog name as it is stored
* in the database;"" retrieves those without a catalog; null means catalog
* name should not be used to narrow down the search.
* @param schemaPattern a schema name; must match the schema name as it is
* stored in the database; null means
* schema name should not be used to narrow down the search.
* @return a ResultSet
object in which each row is a
* schema description
* @exception SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getSchemas(String catalog, String schemaPattern) throws SQLException {
XSQLVAR[] xsqlvars = new XSQLVAR[2];
xsqlvars[0] = new XSQLVAR();
xsqlvars[0].sqltype = ISCConstants.SQL_VARYING;
xsqlvars[0].sqllen = 31;
xsqlvars[0].sqlname = "TABLE_SCHEM";
xsqlvars[0].relname = "TABLESCHEMAS";
xsqlvars[1] = new XSQLVAR();
xsqlvars[1].sqltype = ISCConstants.SQL_VARYING;
xsqlvars[1].sqllen = 31;
xsqlvars[1].sqlname = "TABLE_CATALOG";
xsqlvars[1].relname = "TABLESCHEMAS";
ArrayList rows = new ArrayList(0);
return new FBResultSet(xsqlvars, rows);
}
public boolean isWrapperFor(Class arg0) throws SQLException {
return arg0 != null && arg0.isAssignableFrom(FBDatabaseMetaData.class);
}
public Object unwrap(Class arg0) throws SQLException {
if (!isWrapperFor(arg0))
throw new FBSQLException("No compatible class found.");
return this;
}
}