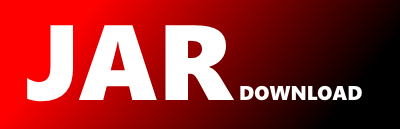
org.firebirdsql.jdbc.FBStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaybird Show documentation
Show all versions of jaybird Show documentation
JDBC Driver for the Firebird RDBMS
package org.firebirdsql.jdbc;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.firebirdsql.gds.impl.GDSHelper;
/**
*
* @author Roman Rokytskyy
*/
public class FBStatement extends AbstractStatement {
/**
*
*/
public FBStatement(GDSHelper c, int rsType, int rsConcurrency, int rsHoldability, FBObjectListener.StatementListener statementListener) throws SQLException {
super(c, rsType, rsConcurrency, rsHoldability, statementListener);
}
/**
* jdbc 3
* @return
* @exception java.sql.SQLException
*/
public ResultSet getGeneratedKeys() throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public int executeUpdate(String param1, int param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("Not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public int executeUpdate(String param1, int[] param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("Not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public int executeUpdate(String param1, String[] param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("Not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public boolean execute(String param1, int param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public boolean execute(String param1, int[] param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
/**
* jdbc 3
* @param param1
* @param param2
* @return
* @exception java.sql.SQLException
*/
public boolean execute(String param1, String[] param2) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
public boolean isPoolable() throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
public void setPoolable(boolean poolable) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
public boolean isWrapperFor(Class arg0) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
public Object unwrap(Class arg0) throws SQLException {
// TODO: implement this java.sql.Statement method
throw new SQLException("not yet implemented");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy