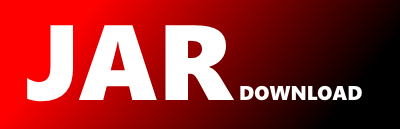
org.bcos.contract.source.Authority Maven / Gradle / Ivy
package org.bcos.contract.source;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Collections;
import java.util.concurrent.Future;
import org.bcos.channel.client.TransactionSucCallback;
import org.bcos.web3j.abi.TypeReference;
import org.bcos.web3j.abi.datatypes.Address;
import org.bcos.web3j.abi.datatypes.Bool;
import org.bcos.web3j.abi.datatypes.Function;
import org.bcos.web3j.abi.datatypes.Type;
import org.bcos.web3j.abi.datatypes.Utf8String;
import org.bcos.web3j.crypto.Credentials;
import org.bcos.web3j.crypto.EncryptType;
import org.bcos.web3j.protocol.Web3j;
import org.bcos.web3j.protocol.core.methods.response.TransactionReceipt;
import org.bcos.web3j.tx.Contract;
import org.bcos.web3j.tx.TransactionManager;
/**
* Auto generated code.
* Do not modify!
* Please use the web3j command line tools, or {@link org.bcos.web3j.codegen.SolidityFunctionWrapperGenerator} to update.
*
* Generated with web3j version none.
*/
public final class Authority extends Contract {
private static String BINARY = "";
public static final String ABI = "[{\"constant\":true,\"inputs\":[],\"name\":\"_version\",\"outputs\":[{\"name\":\"\",\"type\":\"string\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":true,\"inputs\":[{\"name\":\"origin\",\"type\":\"address\"}],\"name\":\"deploy\",\"outputs\":[{\"name\":\"\",\"type\":\"bool\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":true,\"inputs\":[],\"name\":\"_desc\",\"outputs\":[{\"name\":\"\",\"type\":\"string\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[{\"name\":\"version\",\"type\":\"string\"}],\"name\":\"setVersion\",\"outputs\":[],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[{\"name\":\"desc\",\"type\":\"string\"}],\"name\":\"setDesc\",\"outputs\":[],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[],\"name\":\"enable\",\"outputs\":[],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[],\"name\":\"newGroup\",\"outputs\":[{\"name\":\"\",\"type\":\"address\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[{\"name\":\"name\",\"type\":\"string\"}],\"name\":\"setName\",\"outputs\":[],\"payable\":false,\"type\":\"function\"},{\"constant\":false,\"inputs\":[{\"name\":\"user\",\"type\":\"address\"},{\"name\":\"group\",\"type\":\"address\"}],\"name\":\"setUserGroup\",\"outputs\":[],\"payable\":false,\"type\":\"function\"},{\"constant\":true,\"inputs\":[],\"name\":\"_name\",\"outputs\":[{\"name\":\"\",\"type\":\"string\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":true,\"inputs\":[{\"name\":\"user\",\"type\":\"address\"}],\"name\":\"getUserGroup\",\"outputs\":[{\"name\":\"\",\"type\":\"address\"}],\"payable\":false,\"type\":\"function\"},{\"constant\":true,\"inputs\":[{\"name\":\"origin\",\"type\":\"address\"},{\"name\":\"from\",\"type\":\"address\"},{\"name\":\"to\",\"type\":\"address\"},{\"name\":\"func\",\"type\":\"string\"},{\"name\":\"input\",\"type\":\"string\"}],\"name\":\"process\",\"outputs\":[{\"name\":\"\",\"type\":\"bool\"}],\"payable\":false,\"type\":\"function\"}]";
private static String GUOMI_BINARY = "";
private Authority(String contractAddress, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit, Boolean isInitByName) {
super(BINARY, contractAddress, web3j, credentials, gasPrice, gasLimit, isInitByName);
if(EncryptType.encryptType == 1) super.setContractBinary(GUOMI_BINARY);
}
private Authority(String contractAddress, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit, Boolean isInitByName) {
super(BINARY, contractAddress, web3j, transactionManager, gasPrice, gasLimit, isInitByName);
if(EncryptType.encryptType == 1) super.setContractBinary(GUOMI_BINARY);
}
private Authority(String contractAddress, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, credentials, gasPrice, gasLimit, false);
if(EncryptType.encryptType == 1) super.setContractBinary(GUOMI_BINARY);
}
private Authority(String contractAddress, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, transactionManager, gasPrice, gasLimit, false);
if(EncryptType.encryptType == 1) super.setContractBinary(GUOMI_BINARY);
}
public Future _version() {
Function function = new Function("_version",
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public Future deploy(Address origin) {
Function function = new Function("deploy",
Arrays.asList(origin),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public Future _desc() {
Function function = new Function("_desc",
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public Future setVersion(Utf8String version) {
Function function = new Function("setVersion", Arrays.asList(version), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void setVersion(Utf8String version, TransactionSucCallback callback) {
Function function = new Function("setVersion", Arrays.asList(version), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future setDesc(Utf8String desc) {
Function function = new Function("setDesc", Arrays.asList(desc), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void setDesc(Utf8String desc, TransactionSucCallback callback) {
Function function = new Function("setDesc", Arrays.asList(desc), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future enable() {
Function function = new Function("enable", Arrays.asList(), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void enable(TransactionSucCallback callback) {
Function function = new Function("enable", Arrays.asList(), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future newGroup() {
Function function = new Function("newGroup", Arrays.asList(), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void newGroup(TransactionSucCallback callback) {
Function function = new Function("newGroup", Arrays.asList(), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future setName(Utf8String name) {
Function function = new Function("setName", Arrays.asList(name), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void setName(Utf8String name, TransactionSucCallback callback) {
Function function = new Function("setName", Arrays.asList(name), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future setUserGroup(Address user, Address group) {
Function function = new Function("setUserGroup", Arrays.asList(user, group), Collections.>emptyList());
return executeTransactionAsync(function);
}
public void setUserGroup(Address user, Address group, TransactionSucCallback callback) {
Function function = new Function("setUserGroup", Arrays.asList(user, group), Collections.>emptyList());
executeTransactionAsync(function, callback);
}
public Future _name() {
Function function = new Function("_name",
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public Future getUserGroup(Address user) {
Function function = new Function("getUserGroup",
Arrays.asList(user),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public Future process(Address origin, Address from, Address to, Utf8String func, Utf8String input) {
Function function = new Function("process",
Arrays.asList(origin, from, to, func, input),
Arrays.>asList(new TypeReference() {}));
return executeCallSingleValueReturnAsync(function);
}
public static Future deploy(Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit, BigInteger initialWeiValue) {
if(EncryptType.encryptType == 1) setBinary(getGuomiBinary());
return deployAsync(Authority.class, web3j, credentials, gasPrice, gasLimit, BINARY, "", initialWeiValue);
}
public static Future deploy(Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit, BigInteger initialWeiValue) {
if(EncryptType.encryptType == 1) setBinary(getGuomiBinary());
return deployAsync(Authority.class, web3j, transactionManager, gasPrice, gasLimit, BINARY, "", initialWeiValue);
}
public static Authority load(String contractAddress, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
return new Authority(contractAddress, web3j, credentials, gasPrice, gasLimit, false);
}
public static Authority load(String contractAddress, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit) {
return new Authority(contractAddress, web3j, transactionManager, gasPrice, gasLimit, false);
}
public static Authority loadByName(String contractName, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
return new Authority(contractName, web3j, credentials, gasPrice, gasLimit, true);
}
public static Authority loadByName(String contractName, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit) {
return new Authority(contractName, web3j, transactionManager, gasPrice, gasLimit, true);
}
public static void setBinary(String binary) {
BINARY = binary;
}
public static String getGuomiBinary() {
return GUOMI_BINARY;
}
}