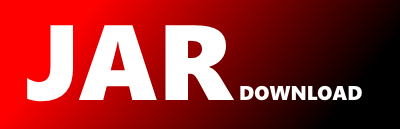
org.bcos.contract.tools.AuthorityManagerTools Maven / Gradle / Ivy
package org.bcos.contract.tools;
import java.math.BigInteger;
import java.util.*;
import org.bcos.channel.client.Service;
import org.bcos.contract.source.AuthorityFilter;
import org.bcos.contract.source.Group;
import org.bcos.contract.source.SystemProxy;
import org.bcos.contract.source.TransactionFilterChain;
import org.bcos.web3j.abi.datatypes.generated.Bytes32;
import org.bcos.web3j.abi.datatypes.generated.Uint256;
import org.bcos.web3j.crypto.Credentials;
import org.bcos.web3j.crypto.GenCredential;
import org.bcos.web3j.crypto.Hash;
import org.bcos.web3j.protocol.Web3j;
import org.bcos.web3j.protocol.channel.ChannelEthereumService;
import org.bcos.web3j.protocol.core.methods.response.TransactionReceipt;
import org.bcos.web3j.utils.Numeric;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.bcos.web3j.abi.datatypes.*;
public class AuthorityManagerTools {
private TransactionFilterChain transactionFilterChain;
private AuthorityFilter authorityFilter;
private Web3j web3j;
private Credentials credentials;
private SystemProxy systemProxy;
ApplicationContext context;
public static BigInteger gasPrice = new BigInteger("99999999999");
public static BigInteger gasLimited = new BigInteger("9999999999999");
public static BigInteger initialValue = new BigInteger("0");
public AuthorityManagerTools() {
authorityFilter=null;
transactionFilterChain =null;
credentials=null;
systemProxy=null;
web3j = null;
context=null;
}
//loadConfig
public boolean loadConfig() throws Exception{
context = new ClassPathXmlApplicationContext("classpath:applicationContext.xml");
Service service = context.getBean(Service.class);
ToolConf toolConf=context.getBean(ToolConf.class);
service.run();
ChannelEthereumService channelEthereumService = new ChannelEthereumService();
channelEthereumService.setChannelService(service);
web3j = Web3j.build(channelEthereumService);
boolean flag=false;
if(web3j!=null){
flag=true;
}
String privKey=toolConf.getPrivKey();
credentials = GenCredential.create(privKey);
systemProxy = SystemProxy.load(toolConf.getSystemProxyAddress(), web3j,credentials, gasPrice,
gasLimited);
transactionFilterChain=TransactionFilterChain.load(SystemContractTools.getAction(systemProxy, "TransactionFilterChain").toString(), web3j, credentials, gasPrice, gasLimited);
return flag;
}
//info
public int getInfo() throws Exception{
Uint256 filtersLength=transactionFilterChain.getFiltersLength().get();
return filtersLength.getValue().intValue();
}
//addFilter
public Boolean addFilter(String name1,String version1,String desc1)throws Exception{
try {
transactionFilterChain.addFilterAndInfo(new Utf8String(name1), new Utf8String(version1), new Utf8String(desc1)).get();
System.out.println(transactionFilterChain.getContractAddress());
Uint256 filtersLength=transactionFilterChain.getFiltersLength().get();
System.out.println("The number of filters on the chain:" + filtersLength.getValue().intValue());
Address filterAddress=transactionFilterChain.getFilter(new Uint256(filtersLength.getValue().intValue()-1)).get();
authorityFilter=AuthorityFilter.load(filterAddress.toString(), web3j, credentials, gasPrice, gasLimited);
Bool authorityFlag=authorityFilter.getEnable().get();
return authorityFlag.getValue();
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
//delFilter
public int delFilter(String num)throws Exception{
int filterNum=Integer.parseInt(num);
int filtersLength=transactionFilterChain.getFiltersLength().get().getValue().intValue();
int delNum=0;
if(filtersLength<= filterNum||filterNum<0){
System.out.println("The number parameter is required to be non-negative and less than the length of the FilterChain "+filtersLength);
return 0;
}else{
System.out.println("Start the delFilter operation:");
try {
transactionFilterChain.delFilter(new Uint256(filterNum)).get();
int filtersLengthNow=transactionFilterChain.getFiltersLength().get().getValue().intValue();
delNum=filtersLength-filtersLengthNow;
} catch (Exception e) {
e.printStackTrace();
}
}
return delNum;
}
//showFilter
public void showFilter()throws Exception{
int filtersLength=transactionFilterChain.getFiltersLength().get().getValue().intValue();
System.out.println("The number of filters on the chain:" + filtersLength);
for(int i=0; i result2=authorityFilter.getInfo().get();
Bool authorityFlag=authorityFilter.getEnable().get();
if(authorityFlag.getValue()){
System.out.println("Filter[" + i+ "]information: "+((Utf8String) result2.get(0)).getValue()+" "+((Utf8String) result2.get(1)).getValue()+" "+((Utf8String) result2.get(2)).getValue()+" The Filter permission control has started");
}else{
System.out.println("Filter[" + i+ "]information: "+((Utf8String) result2.get(0)).getValue()+" "+((Utf8String) result2.get(1)).getValue()+" "+((Utf8String) result2.get(2)).getValue()+" The Filter permission controls the off state");
}
}
}
//resetFilter
public void resetFilter()throws Exception{
System.out.println("Start restoring the chain operation:");
int filtersLength=transactionFilterChain.getFiltersLength().get().getValue().intValue();
for(int i=0; i rlist = group.getKeys().get().getValue();
if(rlist.size()!=0){
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy