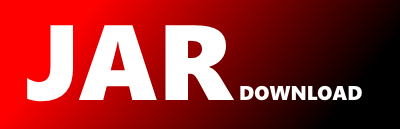
org.bcos.web3j.protocol.rx.Web3jRx Maven / Gradle / Ivy
package org.bcos.web3j.protocol.rx;
import java.math.BigInteger;
import rx.Observable;
import org.bcos.web3j.protocol.core.DefaultBlockParameter;
import org.bcos.web3j.protocol.core.methods.request.EthFilter;
import org.bcos.web3j.protocol.core.methods.response.EthBlock;
import org.bcos.web3j.protocol.core.methods.response.Log;
import org.bcos.web3j.protocol.core.methods.response.Transaction;
/**
* The Observables JSON-RPC client event API.
*/
public interface Web3jRx {
/**
* Create an observable to filter for specific log events on the blockchain.
*
* @param ethFilter filter criteria
* @return Observable that emits all Log events matching the filter
*/
Observable ethLogObservable(EthFilter ethFilter);
/**
* Create an Observable to emit block hashes.
*
* @return Observable that emits all new block hashes as new blocks are created on the
* blockchain
*/
Observable ethBlockHashObservable();
/**
* Create an Observable to emit pending transactions, i.e. those transactions that have been
* submitted by a node, but don't yet form part of a block (haven't been mined yet).
*
* @return Observable to emit pending transaction hashes.
*/
Observable ethPendingTransactionHashObservable();
/**
* Create an Observable to emit all new transactions as they are confirmed on the blockchain.
* i.e. they have been mined and are incorporated into a block.
*
* @return Observable to emit new transactions on the blockchain
*/
Observable transactionObservable();
/**
* Create an Observable to emit all pending transactions that have yet to be placed into a
* block on the blockchain.
*
* @return Observable to emit pending transactions
*/
Observable pendingTransactionObservable();
/**
* Create an Observable that emits newly created blocks on the blockchain.
*
* @param fullTransactionObjects if true, provides transactions embedded in blocks, otherwise
* transaction hashes
* @return Observable that emits all new blocks as they are added to the blockchain
*/
Observable blockObservable(boolean fullTransactionObjects);
/**
* Create an Observable that emits all blocks from the blockchain contained within the
* requested range.
*
* @param startBlock block number to commence with
* @param endBlock block number to finish with
* @param fullTransactionObjects if true, provides transactions embedded in blocks, otherwise
* transaction hashes
* @return Observable to emit these blocks
*/
Observable replayBlocksObservable(
DefaultBlockParameter startBlock, DefaultBlockParameter endBlock,
boolean fullTransactionObjects);
/**
* Create an Observable that emits all transactions from the blockchain contained within the
* requested range.
*
* @param startBlock block number to commence with
* @param endBlock block number to finish with
* @return Observable to emit these transactions in the order they appear in the blocks
*/
Observable replayTransactionsObservable(
DefaultBlockParameter startBlock, DefaultBlockParameter endBlock);
/**
* Create an Observable that emits all transactions from the blockchain starting with a
* provided block number. Once it has replayed up to the most current block, the provided
* Observable is invoked.
*
* To automatically subscribe to new blocks, use
* {@link #catchUpToLatestAndSubscribeToNewBlocksObservable(DefaultBlockParameter, boolean)}.
*
* @param startBlock the block number we wish to request from
* @param fullTransactionObjects if we require full {@link Transaction} objects to be provided
* in the {@link EthBlock} responses
* @param onCompleteObservable a subsequent Observable that we wish to run once we are caught
* up with the latest block
* @return Observable to emit all requested blocks
*/
Observable catchUpToLatestBlockObservable(
DefaultBlockParameter startBlock, boolean fullTransactionObjects,
Observable onCompleteObservable);
/**
* Creates an Observable that emits all blocks from the requested block number to the most
* current. Once it has emitted the most current block, onComplete is called.
*
* @param startBlock the block number we wish to request from
* @param fullTransactionObjects if we require full {@link Transaction} objects to be provided
* in the {@link EthBlock} responses
* @return Observable to emit all requested blocks
*/
Observable catchUpToLatestBlockObservable(
DefaultBlockParameter startBlock, boolean fullTransactionObjects);
/**
* Creates an Observable that emits all transactions from the requested block number to the most
* current. Once it has emitted the most current block's transactions, onComplete is called.
*
* @param startBlock the block number we wish to request from
* @return Observable to emit all requested transactions
*/
Observable catchUpToLatestTransactionObservable(
DefaultBlockParameter startBlock);
/**
* Creates an Observable that emits all blocks from the requested block number to the most
* current. Once it has emitted the most current block, it starts emitting new blocks as they
* are created.
*
* @param startBlock the block number we wish to request from
* @param fullTransactionObjects if we require full {@link Transaction} objects to be provided
* in the {@link EthBlock} responses
* @return Observable to emit all requested blocks and future
*/
Observable catchUpToLatestAndSubscribeToNewBlocksObservable(
DefaultBlockParameter startBlock, boolean fullTransactionObjects);
/**
* As per
* {@link #catchUpToLatestAndSubscribeToNewBlocksObservable(DefaultBlockParameter, boolean)},
* except that all transactions contained within the blocks are emitted.
*
* @param startBlock the block number we wish to request from
* @return Observable to emit all requested transactions and future
*/
Observable catchUpToLatestAndSubscribeToNewTransactionsObservable(
DefaultBlockParameter startBlock);
}