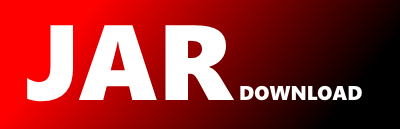
org.fisco.bcos.web3j.precompile.config.SystemConfig Maven / Gradle / Ivy
package org.fisco.bcos.web3j.precompile.config;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.Collections;
import org.fisco.bcos.web3j.abi.TypeReference;
import org.fisco.bcos.web3j.abi.datatypes.Function;
import org.fisco.bcos.web3j.abi.datatypes.Type;
import org.fisco.bcos.web3j.crypto.Credentials;
import org.fisco.bcos.web3j.protocol.Web3j;
import org.fisco.bcos.web3j.protocol.core.RemoteCall;
import org.fisco.bcos.web3j.protocol.core.methods.response.TransactionReceipt;
import org.fisco.bcos.web3j.tx.Contract;
import org.fisco.bcos.web3j.tx.TransactionManager;
import org.fisco.bcos.web3j.tx.gas.ContractGasProvider;
/**
* Auto generated code.
*
* Do not modify!
*
*
Please use the web3j command line tools,
* or the org.fisco.bcos.web3j.codegen.SolidityFunctionWrapperGenerator in the codegen module to update.
*
*
Generated with web3j version none.
*/
public class SystemConfig extends Contract {
private static final String BINARY = "";
public static final String FUNC_SETVALUEBYKEY = "setValueByKey";
@Deprecated
protected SystemConfig(
String contractAddress,
Web3j web3j,
Credentials credentials,
BigInteger gasPrice,
BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, credentials, gasPrice, gasLimit);
}
protected SystemConfig(
String contractAddress,
Web3j web3j,
Credentials credentials,
ContractGasProvider contractGasProvider) {
super(BINARY, contractAddress, web3j, credentials, contractGasProvider);
}
@Deprecated
protected SystemConfig(
String contractAddress,
Web3j web3j,
TransactionManager transactionManager,
BigInteger gasPrice,
BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, transactionManager, gasPrice, gasLimit);
}
protected SystemConfig(
String contractAddress,
Web3j web3j,
TransactionManager transactionManager,
ContractGasProvider contractGasProvider) {
super(BINARY, contractAddress, web3j, transactionManager, contractGasProvider);
}
public RemoteCall setValueByKey(String key, String value) {
final Function function =
new Function(
FUNC_SETVALUEBYKEY,
Arrays.asList(
new org.fisco.bcos.web3j.abi.datatypes.Utf8String(key),
new org.fisco.bcos.web3j.abi.datatypes.Utf8String(value)),
Collections.>emptyList());
return executeRemoteCallTransaction(function);
}
@Deprecated
public static SystemConfig load(
String contractAddress,
Web3j web3j,
Credentials credentials,
BigInteger gasPrice,
BigInteger gasLimit) {
return new SystemConfig(contractAddress, web3j, credentials, gasPrice, gasLimit);
}
@Deprecated
public static SystemConfig load(
String contractAddress,
Web3j web3j,
TransactionManager transactionManager,
BigInteger gasPrice,
BigInteger gasLimit) {
return new SystemConfig(contractAddress, web3j, transactionManager, gasPrice, gasLimit);
}
public static SystemConfig load(
String contractAddress,
Web3j web3j,
Credentials credentials,
ContractGasProvider contractGasProvider) {
return new SystemConfig(contractAddress, web3j, credentials, contractGasProvider);
}
public static SystemConfig load(
String contractAddress,
Web3j web3j,
TransactionManager transactionManager,
ContractGasProvider contractGasProvider) {
return new SystemConfig(contractAddress, web3j, transactionManager, contractGasProvider);
}
public static RemoteCall deploy(
Web3j web3j, Credentials credentials, ContractGasProvider contractGasProvider) {
return deployRemoteCall(
SystemConfig.class, web3j, credentials, contractGasProvider, BINARY, "");
}
@Deprecated
public static RemoteCall deploy(
Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
return deployRemoteCall(SystemConfig.class, web3j, credentials, gasPrice, gasLimit, BINARY, "");
}
public static RemoteCall deploy(
Web3j web3j, TransactionManager transactionManager, ContractGasProvider contractGasProvider) {
return deployRemoteCall(
SystemConfig.class, web3j, transactionManager, contractGasProvider, BINARY, "");
}
@Deprecated
public static RemoteCall deploy(
Web3j web3j,
TransactionManager transactionManager,
BigInteger gasPrice,
BigInteger gasLimit) {
return deployRemoteCall(
SystemConfig.class, web3j, transactionManager, gasPrice, gasLimit, BINARY, "");
}
}