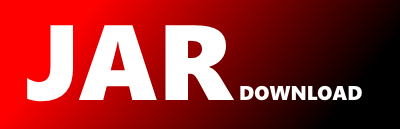
org.eclipse.ui.actions.PartEventAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workbench Show documentation
Show all versions of workbench Show documentation
This plug-in contains the bulk of the Workbench implementation, and depends on JFace, SWT, and Core Runtime. It cannot be used independently from org.eclipse.ui. Workbench client plug-ins should not depend directly on this plug-in.
The newest version!
/*******************************************************************************
* Copyright (c) 2000, 2006 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.ui.actions;
import org.eclipse.jface.action.Action;
import org.eclipse.ui.IPartListener;
import org.eclipse.ui.IWorkbenchPart;
/**
* The abstract superclass for actions that listen to part activation and
* open/close events. This implementation tracks the active part (see
* getActivePart
) and provides a convenient place to monitor
* part lifecycle events that could affect the availability of the action.
*
* Subclasses must implement the following IAction
method:
*
* run
- to do the action's work
*
*
*
* Subclasses may extend any of the IPartListener
methods if the
* action availablity needs to be recalculated:
*
* partActivated
* partDeactivated
* partOpened
* partClosed
* partBroughtToTop
*
*
*
* Although this method implements the IPartListener
interface,
* it does NOT register itself.
*
*/
public abstract class PartEventAction extends Action implements IPartListener {
/**
* The active part, or null
if none.
*/
private IWorkbenchPart activePart;
/**
* Creates a new action with the given text.
*
* @param text the action's text, or null
if there is no text
*/
protected PartEventAction(String text) {
super(text);
}
/**
* Creates a new action with the given text and style.
*
* @param text the action's text, or null
if there is no text
* @param style one of AS_PUSH_BUTTON
, AS_CHECK_BOX
,
* AS_DROP_DOWN_MENU
, AS_RADIO_BUTTON
, and
* AS_UNSPECIFIED
* @since 3.0
*/
protected PartEventAction(String text, int style) {
super(text, style);
}
/**
* Returns the currently active part in the workbench.
*
* @return currently active part in the workbench, or null
if none
*/
public IWorkbenchPart getActivePart() {
return activePart;
}
/**
* The PartEventAction
implementation of this
* IPartListener
method records that the given part is active.
* Subclasses may extend this method if action availability has to be
* recalculated.
*/
public void partActivated(IWorkbenchPart part) {
activePart = part;
}
/**
* The PartEventAction
implementation of this
* IPartListener
method does nothing. Subclasses should extend
* this method if action availability has to be recalculated.
*/
public void partBroughtToTop(IWorkbenchPart part) {
// do nothing
}
/**
* The PartEventAction
implementation of this
* IPartListener
method clears the active part if it just closed.
* Subclasses may extend this method if action availability has to be
* recalculated.
*/
public void partClosed(IWorkbenchPart part) {
if (part == activePart) {
activePart = null;
}
}
/**
* The PartEventAction
implementation of this
* IPartListener
method records that there is no active part.
* Subclasses may extend this method if action availability has to be
* recalculated.
*/
public void partDeactivated(IWorkbenchPart part) {
activePart = null;
}
/**
* The PartEventAction
implementation of this
* IPartListener
method does nothing. Subclasses should extend
* this method if action availability has to be recalculated.
*/
public void partOpened(IWorkbenchPart part) {
// do nothing
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy