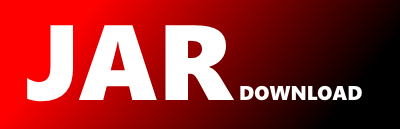
org.fluentcodes.tools.testobjects.ForTestClass Maven / Gradle / Ivy
package org.fluentcodes.tools.testobjects;
import java.util.*;
public class ForTestClass {
private int integer;
private String string;
private boolean bool;
private Date date;
private byte[] bin;
private List stringList;
private Map stringMap;
private ForTestClass forTestClass;
private List forTestList;
private Map forTestMap;
public static final ForTestClass of1() {
return new ForTestClass()
.setInteger(1)
.setBool(true)
.setString(ForTestString.of1())
.setDate(new Date(1))
.setBin("Bin1".getBytes());
}
public static final ForTestClass of2() {
return new ForTestClass()
.setInteger(2)
.setBool(false)
.setString(ForTestString.of2())
.setDate(new Date(2))
.setBin("Bin2".getBytes());
}
public static final ForTestClass of3() {
return new ForTestClass()
.setInteger(3)
.setBool(false)
.setString(ForTestString.of3())
.setDate(new Date(3))
.setBin("Bin3".getBytes());
}
public static final List ofList1() {
List list = new ArrayList();
list.add(of1());
return list;
}
public static final Map ofMap1() {
Map map = new LinkedHashMap<>();
map.put(ForTestString.ofKey1(), of1());
return map;
}
public static final ForTestClass ofForTest() {
return of1().setForTestClass(of2());
}
public static final ForTestClass ofListForTest() {
return of1().setForTestList(ofList1());
}
public static final ForTestClass ofMapForTest() {
return of1().setForTestMap(ofMap1());
}
public int getInteger() {
return integer;
}
public ForTestClass setInteger(int integer) {
this.integer = integer;
return this;
}
public String getString() {
return string;
}
public ForTestClass setString(String string) {
this.string = string;
return this;
}
public boolean isBool() {
return bool;
}
public ForTestClass setBool(boolean bool) {
this.bool = bool;
return this;
}
public Date getDate() {
return date;
}
public ForTestClass setDate(Date date) {
this.date = date;
return this;
}
public byte[] getBin() {
return bin;
}
public ForTestClass setBin(byte[] bin) {
this.bin = bin;
return this;
}
public List getStringList() {
return stringList;
}
public ForTestClass setStringList(List stringList) {
this.stringList = stringList;
return this;
}
public Map getStringMap() {
return stringMap;
}
public ForTestClass setStringMap(Map stringMap) {
this.stringMap = stringMap;
return this;
}
public ForTestClass getForTestClass() {
return forTestClass;
}
public ForTestClass setForTestClass(ForTestClass forTestClass) {
this.forTestClass = forTestClass;
return this;
}
public List getForTestList() {
return forTestList;
}
public ForTestClass setForTestList(List forTestList) {
this.forTestList = forTestList;
return this;
}
public Map getForTestMap() {
return forTestMap;
}
public ForTestClass setForTestMap(Map forTestMap) {
this.forTestMap = forTestMap;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy