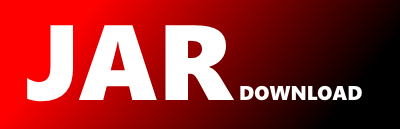
org.flyte.api.v1.AutoOneOf_Literal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import java.util.List;
import java.util.Map;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoOneOfProcessor")
final class AutoOneOf_Literal {
private AutoOneOf_Literal() {} // There are no instances of this type.
static Literal scalar(Scalar scalar) {
if (scalar == null) {
throw new NullPointerException();
}
return new Impl_scalar(scalar);
}
static Literal collection(List collection) {
if (collection == null) {
throw new NullPointerException();
}
return new Impl_collection(collection);
}
static Literal map(Map map) {
if (map == null) {
throw new NullPointerException();
}
return new Impl_map(map);
}
// Parent class that each implementation will inherit from.
private abstract static class Parent_ extends Literal {
@Override
public Scalar scalar() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public List collection() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public Map map() {
throw new UnsupportedOperationException(kind().toString());
}
}
// Implementation when the contained property is "scalar".
private static final class Impl_scalar extends Parent_ {
private final Scalar scalar;
Impl_scalar(Scalar scalar) {
this.scalar = scalar;
}
@Override
public Scalar scalar() {
return scalar;
}
@Override
public String toString() {
return "Literal{scalar=" + this.scalar + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Literal) {
Literal that = (Literal) x;
return this.kind() == that.kind()
&& this.scalar.equals(that.scalar());
} else {
return false;
}
}
@Override
public int hashCode() {
return scalar.hashCode();
}
@Override
public Literal.Kind kind() {
return Literal.Kind.SCALAR;
}
}
// Implementation when the contained property is "collection".
private static final class Impl_collection extends Parent_ {
private final List collection;
Impl_collection(List collection) {
this.collection = collection;
}
@Override
public List collection() {
return collection;
}
@Override
public String toString() {
return "Literal{collection=" + this.collection + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Literal) {
Literal that = (Literal) x;
return this.kind() == that.kind()
&& this.collection.equals(that.collection());
} else {
return false;
}
}
@Override
public int hashCode() {
return collection.hashCode();
}
@Override
public Literal.Kind kind() {
return Literal.Kind.COLLECTION;
}
}
// Implementation when the contained property is "map".
private static final class Impl_map extends Parent_ {
private final Map map;
Impl_map(Map map) {
this.map = map;
}
@Override
public Map map() {
return map;
}
@Override
public String toString() {
return "Literal{map=" + this.map + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Literal) {
Literal that = (Literal) x;
return this.kind() == that.kind()
&& this.map.equals(that.map());
} else {
return false;
}
}
@Override
public int hashCode() {
return map.hashCode();
}
@Override
public Literal.Kind kind() {
return Literal.Kind.MAP;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy