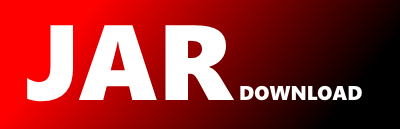
org.flyte.api.v1.AutoOneOf_Primitive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import java.time.Duration;
import java.time.Instant;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoOneOfProcessor")
final class AutoOneOf_Primitive {
private AutoOneOf_Primitive() {} // There are no instances of this type.
static Primitive booleanValue(boolean booleanValue) {
return new Impl_booleanValue(booleanValue);
}
static Primitive integerValue(long integerValue) {
return new Impl_integerValue(integerValue);
}
static Primitive floatValue(double floatValue) {
return new Impl_floatValue(floatValue);
}
static Primitive stringValue(String stringValue) {
if (stringValue == null) {
throw new NullPointerException();
}
return new Impl_stringValue(stringValue);
}
static Primitive datetime(Instant datetime) {
if (datetime == null) {
throw new NullPointerException();
}
return new Impl_datetime(datetime);
}
static Primitive duration(Duration duration) {
if (duration == null) {
throw new NullPointerException();
}
return new Impl_duration(duration);
}
// Parent class that each implementation will inherit from.
private abstract static class Parent_ extends Primitive {
@Override
public boolean booleanValue() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public long integerValue() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public double floatValue() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public String stringValue() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public Instant datetime() {
throw new UnsupportedOperationException(kind().toString());
}
@Override
public Duration duration() {
throw new UnsupportedOperationException(kind().toString());
}
}
// Implementation when the contained property is "booleanValue".
private static final class Impl_booleanValue extends Parent_ {
private final boolean booleanValue;
Impl_booleanValue(boolean booleanValue) {
this.booleanValue = booleanValue;
}
@Override
public boolean booleanValue() {
return booleanValue;
}
@Override
public String toString() {
return "Primitive{booleanValue=" + this.booleanValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& this.booleanValue == that.booleanValue();
} else {
return false;
}
}
@Override
public int hashCode() {
return booleanValue ? 1231 : 1237;
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.BOOLEAN_VALUE;
}
}
// Implementation when the contained property is "integerValue".
private static final class Impl_integerValue extends Parent_ {
private final long integerValue;
Impl_integerValue(long integerValue) {
this.integerValue = integerValue;
}
@Override
public long integerValue() {
return integerValue;
}
@Override
public String toString() {
return "Primitive{integerValue=" + this.integerValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& this.integerValue == that.integerValue();
} else {
return false;
}
}
@Override
public int hashCode() {
return (int) ((integerValue >>> 32) ^ integerValue);
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.INTEGER_VALUE;
}
}
// Implementation when the contained property is "floatValue".
private static final class Impl_floatValue extends Parent_ {
private final double floatValue;
Impl_floatValue(double floatValue) {
this.floatValue = floatValue;
}
@Override
public double floatValue() {
return floatValue;
}
@Override
public String toString() {
return "Primitive{floatValue=" + this.floatValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& Double.doubleToLongBits(this.floatValue) == Double.doubleToLongBits(that.floatValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return (int) ((Double.doubleToLongBits(floatValue) >>> 32) ^ Double.doubleToLongBits(floatValue));
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.FLOAT_VALUE;
}
}
// Implementation when the contained property is "stringValue".
private static final class Impl_stringValue extends Parent_ {
private final String stringValue;
Impl_stringValue(String stringValue) {
this.stringValue = stringValue;
}
@Override
public String stringValue() {
return stringValue;
}
@Override
public String toString() {
return "Primitive{stringValue=" + this.stringValue + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& this.stringValue.equals(that.stringValue());
} else {
return false;
}
}
@Override
public int hashCode() {
return stringValue.hashCode();
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.STRING_VALUE;
}
}
// Implementation when the contained property is "datetime".
private static final class Impl_datetime extends Parent_ {
private final Instant datetime;
Impl_datetime(Instant datetime) {
this.datetime = datetime;
}
@Override
public Instant datetime() {
return datetime;
}
@Override
public String toString() {
return "Primitive{datetime=" + this.datetime + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& this.datetime.equals(that.datetime());
} else {
return false;
}
}
@Override
public int hashCode() {
return datetime.hashCode();
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.DATETIME;
}
}
// Implementation when the contained property is "duration".
private static final class Impl_duration extends Parent_ {
private final Duration duration;
Impl_duration(Duration duration) {
this.duration = duration;
}
@Override
public Duration duration() {
return duration;
}
@Override
public String toString() {
return "Primitive{duration=" + this.duration + "}";
}
@Override
public boolean equals(Object x) {
if (x instanceof Primitive) {
Primitive that = (Primitive) x;
return this.kind() == that.kind()
&& this.duration.equals(that.duration());
} else {
return false;
}
}
@Override
public int hashCode() {
return duration.hashCode();
}
@Override
public Primitive.Kind kind() {
return Primitive.Kind.DURATION;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy