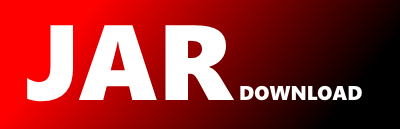
org.flyte.api.v1.AutoValue_Blob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Blob extends Blob {
private final BlobMetadata metadata;
private final String uri;
private AutoValue_Blob(
BlobMetadata metadata,
String uri) {
this.metadata = metadata;
this.uri = uri;
}
@Override
public BlobMetadata metadata() {
return metadata;
}
@Override
public String uri() {
return uri;
}
@Override
public String toString() {
return "Blob{"
+ "metadata=" + metadata + ", "
+ "uri=" + uri
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Blob) {
Blob that = (Blob) o;
return this.metadata.equals(that.metadata())
&& this.uri.equals(that.uri());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= metadata.hashCode();
h$ *= 1000003;
h$ ^= uri.hashCode();
return h$;
}
static final class Builder extends Blob.Builder {
private BlobMetadata metadata;
private String uri;
Builder() {
}
@Override
public Blob.Builder metadata(BlobMetadata metadata) {
if (metadata == null) {
throw new NullPointerException("Null metadata");
}
this.metadata = metadata;
return this;
}
@Override
public Blob.Builder uri(String uri) {
if (uri == null) {
throw new NullPointerException("Null uri");
}
this.uri = uri;
return this;
}
@Override
public Blob build() {
if (this.metadata == null
|| this.uri == null) {
StringBuilder missing = new StringBuilder();
if (this.metadata == null) {
missing.append(" metadata");
}
if (this.uri == null) {
missing.append(" uri");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Blob(
this.metadata,
this.uri);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy