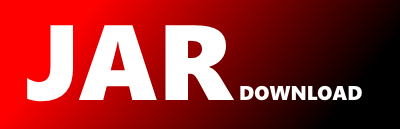
org.flyte.api.v1.AutoValue_Container Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import java.util.List;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Container extends Container {
private final List command;
private final List args;
private final String image;
private final List env;
@Nullable
private final Resources resources;
private AutoValue_Container(
List command,
List args,
String image,
List env,
@Nullable Resources resources) {
this.command = command;
this.args = args;
this.image = image;
this.env = env;
this.resources = resources;
}
@Override
public List command() {
return command;
}
@Override
public List args() {
return args;
}
@Override
public String image() {
return image;
}
@Override
public List env() {
return env;
}
@Nullable
@Override
public Resources resources() {
return resources;
}
@Override
public String toString() {
return "Container{"
+ "command=" + command + ", "
+ "args=" + args + ", "
+ "image=" + image + ", "
+ "env=" + env + ", "
+ "resources=" + resources
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Container) {
Container that = (Container) o;
return this.command.equals(that.command())
&& this.args.equals(that.args())
&& this.image.equals(that.image())
&& this.env.equals(that.env())
&& (this.resources == null ? that.resources() == null : this.resources.equals(that.resources()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= command.hashCode();
h$ *= 1000003;
h$ ^= args.hashCode();
h$ *= 1000003;
h$ ^= image.hashCode();
h$ *= 1000003;
h$ ^= env.hashCode();
h$ *= 1000003;
h$ ^= (resources == null) ? 0 : resources.hashCode();
return h$;
}
static final class Builder extends Container.Builder {
private List command;
private List args;
private String image;
private List env;
private Resources resources;
Builder() {
}
@Override
public Container.Builder command(List command) {
if (command == null) {
throw new NullPointerException("Null command");
}
this.command = command;
return this;
}
@Override
public Container.Builder args(List args) {
if (args == null) {
throw new NullPointerException("Null args");
}
this.args = args;
return this;
}
@Override
public Container.Builder image(String image) {
if (image == null) {
throw new NullPointerException("Null image");
}
this.image = image;
return this;
}
@Override
public Container.Builder env(List env) {
if (env == null) {
throw new NullPointerException("Null env");
}
this.env = env;
return this;
}
@Override
public Container.Builder resources(Resources resources) {
this.resources = resources;
return this;
}
@Override
public Container build() {
if (this.command == null
|| this.args == null
|| this.image == null
|| this.env == null) {
StringBuilder missing = new StringBuilder();
if (this.command == null) {
missing.append(" command");
}
if (this.args == null) {
missing.append(" args");
}
if (this.image == null) {
missing.append(" image");
}
if (this.env == null) {
missing.append(" env");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Container(
this.command,
this.args,
this.image,
this.env,
this.resources);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy