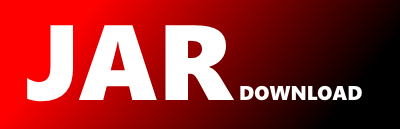
org.flyte.api.v1.AutoValue_LaunchPlan Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import java.util.Map;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_LaunchPlan extends LaunchPlan {
private final String name;
private final PartialWorkflowIdentifier workflowId;
private final Map fixedInputs;
private final Map defaultInputs;
@Nullable
private final CronSchedule cronSchedule;
private AutoValue_LaunchPlan(
String name,
PartialWorkflowIdentifier workflowId,
Map fixedInputs,
Map defaultInputs,
@Nullable CronSchedule cronSchedule) {
this.name = name;
this.workflowId = workflowId;
this.fixedInputs = fixedInputs;
this.defaultInputs = defaultInputs;
this.cronSchedule = cronSchedule;
}
@Override
public String name() {
return name;
}
@Override
public PartialWorkflowIdentifier workflowId() {
return workflowId;
}
@Override
public Map fixedInputs() {
return fixedInputs;
}
@Override
public Map defaultInputs() {
return defaultInputs;
}
@Nullable
@Override
public CronSchedule cronSchedule() {
return cronSchedule;
}
@Override
public String toString() {
return "LaunchPlan{"
+ "name=" + name + ", "
+ "workflowId=" + workflowId + ", "
+ "fixedInputs=" + fixedInputs + ", "
+ "defaultInputs=" + defaultInputs + ", "
+ "cronSchedule=" + cronSchedule
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof LaunchPlan) {
LaunchPlan that = (LaunchPlan) o;
return this.name.equals(that.name())
&& this.workflowId.equals(that.workflowId())
&& this.fixedInputs.equals(that.fixedInputs())
&& this.defaultInputs.equals(that.defaultInputs())
&& (this.cronSchedule == null ? that.cronSchedule() == null : this.cronSchedule.equals(that.cronSchedule()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= workflowId.hashCode();
h$ *= 1000003;
h$ ^= fixedInputs.hashCode();
h$ *= 1000003;
h$ ^= defaultInputs.hashCode();
h$ *= 1000003;
h$ ^= (cronSchedule == null) ? 0 : cronSchedule.hashCode();
return h$;
}
static final class Builder extends LaunchPlan.Builder {
private String name;
private PartialWorkflowIdentifier workflowId;
private Map fixedInputs;
private Map defaultInputs;
private CronSchedule cronSchedule;
Builder() {
}
@Override
public LaunchPlan.Builder name(String name) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
return this;
}
@Override
public LaunchPlan.Builder workflowId(PartialWorkflowIdentifier workflowId) {
if (workflowId == null) {
throw new NullPointerException("Null workflowId");
}
this.workflowId = workflowId;
return this;
}
@Override
public LaunchPlan.Builder fixedInputs(Map fixedInputs) {
if (fixedInputs == null) {
throw new NullPointerException("Null fixedInputs");
}
this.fixedInputs = fixedInputs;
return this;
}
@Override
public LaunchPlan.Builder defaultInputs(Map defaultInputs) {
if (defaultInputs == null) {
throw new NullPointerException("Null defaultInputs");
}
this.defaultInputs = defaultInputs;
return this;
}
@Override
public LaunchPlan.Builder cronSchedule(CronSchedule cronSchedule) {
this.cronSchedule = cronSchedule;
return this;
}
@Override
public LaunchPlan build() {
if (this.name == null
|| this.workflowId == null
|| this.fixedInputs == null
|| this.defaultInputs == null) {
StringBuilder missing = new StringBuilder();
if (this.name == null) {
missing.append(" name");
}
if (this.workflowId == null) {
missing.append(" workflowId");
}
if (this.fixedInputs == null) {
missing.append(" fixedInputs");
}
if (this.defaultInputs == null) {
missing.append(" defaultInputs");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_LaunchPlan(
this.name,
this.workflowId,
this.fixedInputs,
this.defaultInputs,
this.cronSchedule);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy