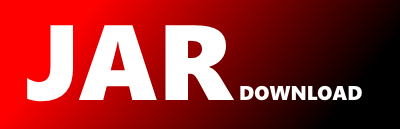
org.flyte.api.v1.AutoValue_Node Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import java.util.List;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Node extends Node {
private final String id;
@Nullable
private final NodeMetadata metadata;
@Nullable
private final TaskNode taskNode;
@Nullable
private final BranchNode branchNode;
@Nullable
private final WorkflowNode workflowNode;
private final List inputs;
private final List upstreamNodeIds;
private AutoValue_Node(
String id,
@Nullable NodeMetadata metadata,
@Nullable TaskNode taskNode,
@Nullable BranchNode branchNode,
@Nullable WorkflowNode workflowNode,
List inputs,
List upstreamNodeIds) {
this.id = id;
this.metadata = metadata;
this.taskNode = taskNode;
this.branchNode = branchNode;
this.workflowNode = workflowNode;
this.inputs = inputs;
this.upstreamNodeIds = upstreamNodeIds;
}
@Override
public String id() {
return id;
}
@Nullable
@Override
public NodeMetadata metadata() {
return metadata;
}
@Nullable
@Override
public TaskNode taskNode() {
return taskNode;
}
@Nullable
@Override
public BranchNode branchNode() {
return branchNode;
}
@Nullable
@Override
public WorkflowNode workflowNode() {
return workflowNode;
}
@Override
public List inputs() {
return inputs;
}
@Override
public List upstreamNodeIds() {
return upstreamNodeIds;
}
@Override
public String toString() {
return "Node{"
+ "id=" + id + ", "
+ "metadata=" + metadata + ", "
+ "taskNode=" + taskNode + ", "
+ "branchNode=" + branchNode + ", "
+ "workflowNode=" + workflowNode + ", "
+ "inputs=" + inputs + ", "
+ "upstreamNodeIds=" + upstreamNodeIds
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Node) {
Node that = (Node) o;
return this.id.equals(that.id())
&& (this.metadata == null ? that.metadata() == null : this.metadata.equals(that.metadata()))
&& (this.taskNode == null ? that.taskNode() == null : this.taskNode.equals(that.taskNode()))
&& (this.branchNode == null ? that.branchNode() == null : this.branchNode.equals(that.branchNode()))
&& (this.workflowNode == null ? that.workflowNode() == null : this.workflowNode.equals(that.workflowNode()))
&& this.inputs.equals(that.inputs())
&& this.upstreamNodeIds.equals(that.upstreamNodeIds());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= (metadata == null) ? 0 : metadata.hashCode();
h$ *= 1000003;
h$ ^= (taskNode == null) ? 0 : taskNode.hashCode();
h$ *= 1000003;
h$ ^= (branchNode == null) ? 0 : branchNode.hashCode();
h$ *= 1000003;
h$ ^= (workflowNode == null) ? 0 : workflowNode.hashCode();
h$ *= 1000003;
h$ ^= inputs.hashCode();
h$ *= 1000003;
h$ ^= upstreamNodeIds.hashCode();
return h$;
}
@Override
public Node.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends Node.Builder {
private String id;
private NodeMetadata metadata;
private TaskNode taskNode;
private BranchNode branchNode;
private WorkflowNode workflowNode;
private List inputs;
private List upstreamNodeIds;
Builder() {
}
private Builder(Node source) {
this.id = source.id();
this.metadata = source.metadata();
this.taskNode = source.taskNode();
this.branchNode = source.branchNode();
this.workflowNode = source.workflowNode();
this.inputs = source.inputs();
this.upstreamNodeIds = source.upstreamNodeIds();
}
@Override
public Node.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public Node.Builder metadata(NodeMetadata metadata) {
this.metadata = metadata;
return this;
}
@Override
public Node.Builder taskNode(TaskNode taskNode) {
this.taskNode = taskNode;
return this;
}
@Override
public Node.Builder branchNode(BranchNode branchNode) {
this.branchNode = branchNode;
return this;
}
@Override
public Node.Builder workflowNode(WorkflowNode workflowNode) {
this.workflowNode = workflowNode;
return this;
}
@Override
public Node.Builder inputs(List inputs) {
if (inputs == null) {
throw new NullPointerException("Null inputs");
}
this.inputs = inputs;
return this;
}
@Override
public Node.Builder upstreamNodeIds(List upstreamNodeIds) {
if (upstreamNodeIds == null) {
throw new NullPointerException("Null upstreamNodeIds");
}
this.upstreamNodeIds = upstreamNodeIds;
return this;
}
@Override
public Node build() {
if (this.id == null
|| this.inputs == null
|| this.upstreamNodeIds == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.inputs == null) {
missing.append(" inputs");
}
if (this.upstreamNodeIds == null) {
missing.append(" upstreamNodeIds");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Node(
this.id,
this.metadata,
this.taskNode,
this.branchNode,
this.workflowNode,
this.inputs,
this.upstreamNodeIds);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy