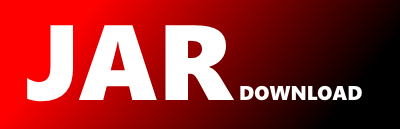
org.flyte.api.v1.AutoValue_TaskTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flytekit-api Show documentation
Show all versions of flytekit-api Show documentation
Java friendly representation of flyteidl protos.
package org.flyte.api.v1;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TaskTemplate extends TaskTemplate {
@Nullable
private final Container container;
private final TypedInterface interface_;
private final String type;
private final RetryStrategy retries;
private final Struct custom;
private final boolean discoverable;
@Nullable
private final String discoveryVersion;
private final boolean cacheSerializable;
private AutoValue_TaskTemplate(
@Nullable Container container,
TypedInterface interface_,
String type,
RetryStrategy retries,
Struct custom,
boolean discoverable,
@Nullable String discoveryVersion,
boolean cacheSerializable) {
this.container = container;
this.interface_ = interface_;
this.type = type;
this.retries = retries;
this.custom = custom;
this.discoverable = discoverable;
this.discoveryVersion = discoveryVersion;
this.cacheSerializable = cacheSerializable;
}
@Nullable
@Override
public Container container() {
return container;
}
@Override
public TypedInterface interface_() {
return interface_;
}
@Override
public String type() {
return type;
}
@Override
public RetryStrategy retries() {
return retries;
}
@Override
public Struct custom() {
return custom;
}
@Override
public boolean discoverable() {
return discoverable;
}
@Nullable
@Override
public String discoveryVersion() {
return discoveryVersion;
}
@Override
public boolean cacheSerializable() {
return cacheSerializable;
}
@Override
public String toString() {
return "TaskTemplate{"
+ "container=" + container + ", "
+ "interface_=" + interface_ + ", "
+ "type=" + type + ", "
+ "retries=" + retries + ", "
+ "custom=" + custom + ", "
+ "discoverable=" + discoverable + ", "
+ "discoveryVersion=" + discoveryVersion + ", "
+ "cacheSerializable=" + cacheSerializable
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TaskTemplate) {
TaskTemplate that = (TaskTemplate) o;
return (this.container == null ? that.container() == null : this.container.equals(that.container()))
&& this.interface_.equals(that.interface_())
&& this.type.equals(that.type())
&& this.retries.equals(that.retries())
&& this.custom.equals(that.custom())
&& this.discoverable == that.discoverable()
&& (this.discoveryVersion == null ? that.discoveryVersion() == null : this.discoveryVersion.equals(that.discoveryVersion()))
&& this.cacheSerializable == that.cacheSerializable();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (container == null) ? 0 : container.hashCode();
h$ *= 1000003;
h$ ^= interface_.hashCode();
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= retries.hashCode();
h$ *= 1000003;
h$ ^= custom.hashCode();
h$ *= 1000003;
h$ ^= discoverable ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (discoveryVersion == null) ? 0 : discoveryVersion.hashCode();
h$ *= 1000003;
h$ ^= cacheSerializable ? 1231 : 1237;
return h$;
}
@Override
public TaskTemplate.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends TaskTemplate.Builder {
private Container container;
private TypedInterface interface_;
private String type;
private RetryStrategy retries;
private Struct custom;
private boolean discoverable;
private String discoveryVersion;
private boolean cacheSerializable;
private byte set$0;
Builder() {
}
private Builder(TaskTemplate source) {
this.container = source.container();
this.interface_ = source.interface_();
this.type = source.type();
this.retries = source.retries();
this.custom = source.custom();
this.discoverable = source.discoverable();
this.discoveryVersion = source.discoveryVersion();
this.cacheSerializable = source.cacheSerializable();
set$0 = (byte) 3;
}
@Override
public TaskTemplate.Builder container(Container container) {
this.container = container;
return this;
}
@Override
public TaskTemplate.Builder interface_(TypedInterface interface_) {
if (interface_ == null) {
throw new NullPointerException("Null interface_");
}
this.interface_ = interface_;
return this;
}
@Override
public TaskTemplate.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public TaskTemplate.Builder retries(RetryStrategy retries) {
if (retries == null) {
throw new NullPointerException("Null retries");
}
this.retries = retries;
return this;
}
@Override
public TaskTemplate.Builder custom(Struct custom) {
if (custom == null) {
throw new NullPointerException("Null custom");
}
this.custom = custom;
return this;
}
@Override
public TaskTemplate.Builder discoverable(boolean discoverable) {
this.discoverable = discoverable;
set$0 |= (byte) 1;
return this;
}
@Override
public TaskTemplate.Builder discoveryVersion(String discoveryVersion) {
this.discoveryVersion = discoveryVersion;
return this;
}
@Override
public TaskTemplate.Builder cacheSerializable(boolean cacheSerializable) {
this.cacheSerializable = cacheSerializable;
set$0 |= (byte) 2;
return this;
}
@Override
public TaskTemplate build() {
if (set$0 != 3
|| this.interface_ == null
|| this.type == null
|| this.retries == null
|| this.custom == null) {
StringBuilder missing = new StringBuilder();
if (this.interface_ == null) {
missing.append(" interface_");
}
if (this.type == null) {
missing.append(" type");
}
if (this.retries == null) {
missing.append(" retries");
}
if (this.custom == null) {
missing.append(" custom");
}
if ((set$0 & 1) == 0) {
missing.append(" discoverable");
}
if ((set$0 & 2) == 0) {
missing.append(" cacheSerializable");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TaskTemplate(
this.container,
this.interface_,
this.type,
this.retries,
this.custom,
this.discoverable,
this.discoveryVersion,
this.cacheSerializable);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy