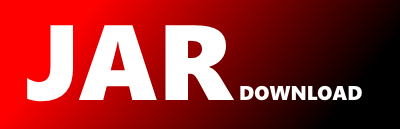
org.flyte.examples.AutoValue_AllInputsTask_AutoAllInputsInput Maven / Gradle / Ivy
package org.flyte.examples;
import java.time.Duration;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import javax.annotation.processing.Generated;
import org.flyte.flytekit.SdkBindingData;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_AllInputsTask_AutoAllInputsInput extends AllInputsTask.AutoAllInputsInput {
private final SdkBindingData i;
private final SdkBindingData f;
private final SdkBindingData s;
private final SdkBindingData b;
private final SdkBindingData t;
private final SdkBindingData d;
private final SdkBindingData> l;
private final SdkBindingData
© 2015 - 2025 Weber Informatics LLC | Privacy Policy