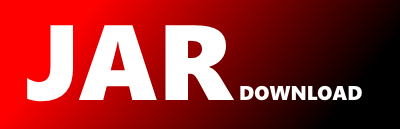
org.flyte.jflyte.utils.AutoValue_Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jflyte-utils Show documentation
Show all versions of jflyte-utils Show documentation
Primarily used by jflyte, but can also be used to extend or build a jflyte alternative
package org.flyte.jflyte.utils;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Config extends Config {
private final String platformUrl;
private final String image;
@Nullable
private final String stagingLocation;
private final String moduleDir;
private final boolean platformInsecure;
private AutoValue_Config(
String platformUrl,
String image,
@Nullable String stagingLocation,
String moduleDir,
boolean platformInsecure) {
this.platformUrl = platformUrl;
this.image = image;
this.stagingLocation = stagingLocation;
this.moduleDir = moduleDir;
this.platformInsecure = platformInsecure;
}
@Override
public String platformUrl() {
return platformUrl;
}
@Override
public String image() {
return image;
}
@Nullable
@Override
public String stagingLocation() {
return stagingLocation;
}
@Override
public String moduleDir() {
return moduleDir;
}
@Override
public boolean platformInsecure() {
return platformInsecure;
}
@Override
public String toString() {
return "Config{"
+ "platformUrl=" + platformUrl + ", "
+ "image=" + image + ", "
+ "stagingLocation=" + stagingLocation + ", "
+ "moduleDir=" + moduleDir + ", "
+ "platformInsecure=" + platformInsecure
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Config) {
Config that = (Config) o;
return this.platformUrl.equals(that.platformUrl())
&& this.image.equals(that.image())
&& (this.stagingLocation == null ? that.stagingLocation() == null : this.stagingLocation.equals(that.stagingLocation()))
&& this.moduleDir.equals(that.moduleDir())
&& this.platformInsecure == that.platformInsecure();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= platformUrl.hashCode();
h$ *= 1000003;
h$ ^= image.hashCode();
h$ *= 1000003;
h$ ^= (stagingLocation == null) ? 0 : stagingLocation.hashCode();
h$ *= 1000003;
h$ ^= moduleDir.hashCode();
h$ *= 1000003;
h$ ^= platformInsecure ? 1231 : 1237;
return h$;
}
static final class Builder extends Config.Builder {
private String platformUrl;
private String image;
private String stagingLocation;
private String moduleDir;
private boolean platformInsecure;
private byte set$0;
Builder() {
}
@Override
Config.Builder platformUrl(String platformUrl) {
if (platformUrl == null) {
throw new NullPointerException("Null platformUrl");
}
this.platformUrl = platformUrl;
return this;
}
@Override
Config.Builder image(String image) {
if (image == null) {
throw new NullPointerException("Null image");
}
this.image = image;
return this;
}
@Override
Config.Builder stagingLocation(String stagingLocation) {
this.stagingLocation = stagingLocation;
return this;
}
@Override
Config.Builder moduleDir(String moduleDir) {
if (moduleDir == null) {
throw new NullPointerException("Null moduleDir");
}
this.moduleDir = moduleDir;
return this;
}
@Override
Config.Builder platformInsecure(boolean platformInsecure) {
this.platformInsecure = platformInsecure;
set$0 |= (byte) 1;
return this;
}
@Override
Config build() {
if (set$0 != 1
|| this.platformUrl == null
|| this.image == null
|| this.moduleDir == null) {
StringBuilder missing = new StringBuilder();
if (this.platformUrl == null) {
missing.append(" platformUrl");
}
if (this.image == null) {
missing.append(" image");
}
if (this.moduleDir == null) {
missing.append(" moduleDir");
}
if ((set$0 & 1) == 0) {
missing.append(" platformInsecure");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Config(
this.platformUrl,
this.image,
this.stagingLocation,
this.moduleDir,
this.platformInsecure);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy