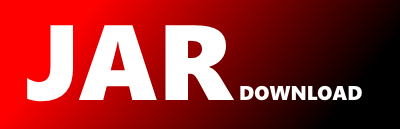
org.flyte.jflyte.utils.AutoValue_IdentifierRewrite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jflyte-utils Show documentation
Show all versions of jflyte-utils Show documentation
Primarily used by jflyte, but can also be used to extend or build a jflyte alternative
package org.flyte.jflyte.utils;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_IdentifierRewrite extends IdentifierRewrite {
private final String domain;
private final String project;
private final String version;
private final FlyteAdminClient adminClient;
private AutoValue_IdentifierRewrite(
String domain,
String project,
String version,
FlyteAdminClient adminClient) {
this.domain = domain;
this.project = project;
this.version = version;
this.adminClient = adminClient;
}
@Override
String domain() {
return domain;
}
@Override
String project() {
return project;
}
@Override
String version() {
return version;
}
@Override
FlyteAdminClient adminClient() {
return adminClient;
}
@Override
public String toString() {
return "IdentifierRewrite{"
+ "domain=" + domain + ", "
+ "project=" + project + ", "
+ "version=" + version + ", "
+ "adminClient=" + adminClient
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IdentifierRewrite) {
IdentifierRewrite that = (IdentifierRewrite) o;
return this.domain.equals(that.domain())
&& this.project.equals(that.project())
&& this.version.equals(that.version())
&& this.adminClient.equals(that.adminClient());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= domain.hashCode();
h$ *= 1000003;
h$ ^= project.hashCode();
h$ *= 1000003;
h$ ^= version.hashCode();
h$ *= 1000003;
h$ ^= adminClient.hashCode();
return h$;
}
static final class Builder extends IdentifierRewrite.Builder {
private String domain;
private String project;
private String version;
private FlyteAdminClient adminClient;
Builder() {
}
@Override
public IdentifierRewrite.Builder domain(String domain) {
if (domain == null) {
throw new NullPointerException("Null domain");
}
this.domain = domain;
return this;
}
@Override
public IdentifierRewrite.Builder project(String project) {
if (project == null) {
throw new NullPointerException("Null project");
}
this.project = project;
return this;
}
@Override
public IdentifierRewrite.Builder version(String version) {
if (version == null) {
throw new NullPointerException("Null version");
}
this.version = version;
return this;
}
@Override
public IdentifierRewrite.Builder adminClient(FlyteAdminClient adminClient) {
if (adminClient == null) {
throw new NullPointerException("Null adminClient");
}
this.adminClient = adminClient;
return this;
}
@Override
public IdentifierRewrite build() {
if (this.domain == null
|| this.project == null
|| this.version == null
|| this.adminClient == null) {
StringBuilder missing = new StringBuilder();
if (this.domain == null) {
missing.append(" domain");
}
if (this.project == null) {
missing.append(" project");
}
if (this.version == null) {
missing.append(" version");
}
if (this.adminClient == null) {
missing.append(" adminClient");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_IdentifierRewrite(
this.domain,
this.project,
this.version,
this.adminClient);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy