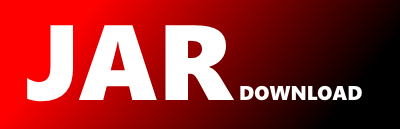
org.flyte.jflyte.utils.AutoValue_ProjectClosure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jflyte-utils Show documentation
Show all versions of jflyte-utils Show documentation
Primarily used by jflyte, but can also be used to extend or build a jflyte alternative
package org.flyte.jflyte.utils;
import java.util.Map;
import javax.annotation.processing.Generated;
import org.flyte.api.v1.LaunchPlan;
import org.flyte.api.v1.LaunchPlanIdentifier;
import org.flyte.api.v1.TaskIdentifier;
import org.flyte.api.v1.WorkflowIdentifier;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ProjectClosure extends ProjectClosure {
private final Map taskSpecs;
private final Map workflowSpecs;
private final Map launchPlans;
private AutoValue_ProjectClosure(
Map taskSpecs,
Map workflowSpecs,
Map launchPlans) {
this.taskSpecs = taskSpecs;
this.workflowSpecs = workflowSpecs;
this.launchPlans = launchPlans;
}
@Override
public Map taskSpecs() {
return taskSpecs;
}
@Override
public Map workflowSpecs() {
return workflowSpecs;
}
@Override
public Map launchPlans() {
return launchPlans;
}
@Override
public String toString() {
return "ProjectClosure{"
+ "taskSpecs=" + taskSpecs + ", "
+ "workflowSpecs=" + workflowSpecs + ", "
+ "launchPlans=" + launchPlans
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ProjectClosure) {
ProjectClosure that = (ProjectClosure) o;
return this.taskSpecs.equals(that.taskSpecs())
&& this.workflowSpecs.equals(that.workflowSpecs())
&& this.launchPlans.equals(that.launchPlans());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= taskSpecs.hashCode();
h$ *= 1000003;
h$ ^= workflowSpecs.hashCode();
h$ *= 1000003;
h$ ^= launchPlans.hashCode();
return h$;
}
static final class Builder extends ProjectClosure.Builder {
private Map taskSpecs;
private Map workflowSpecs;
private Map launchPlans;
Builder() {
}
@Override
ProjectClosure.Builder taskSpecs(Map taskSpecs) {
if (taskSpecs == null) {
throw new NullPointerException("Null taskSpecs");
}
this.taskSpecs = taskSpecs;
return this;
}
@Override
ProjectClosure.Builder workflowSpecs(Map workflowSpecs) {
if (workflowSpecs == null) {
throw new NullPointerException("Null workflowSpecs");
}
this.workflowSpecs = workflowSpecs;
return this;
}
@Override
ProjectClosure.Builder launchPlans(Map launchPlans) {
if (launchPlans == null) {
throw new NullPointerException("Null launchPlans");
}
this.launchPlans = launchPlans;
return this;
}
@Override
ProjectClosure build() {
if (this.taskSpecs == null
|| this.workflowSpecs == null
|| this.launchPlans == null) {
StringBuilder missing = new StringBuilder();
if (this.taskSpecs == null) {
missing.append(" taskSpecs");
}
if (this.workflowSpecs == null) {
missing.append(" workflowSpecs");
}
if (this.launchPlans == null) {
missing.append(" launchPlans");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ProjectClosure(
this.taskSpecs,
this.workflowSpecs,
this.launchPlans);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy