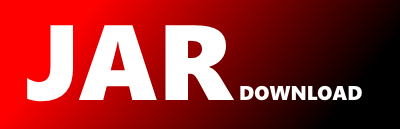
org.fosstrak.llrp.commander.util.LLRP Maven / Gradle / Ivy
/*
*
* Fosstrak LLRP Commander (www.fosstrak.org)
*
* Copyright (C) 2008 ETH Zurich
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see
*
*/
package org.fosstrak.llrp.commander.util;
import java.io.IOException;
import java.io.StringWriter;
import java.net.URL;
import java.util.LinkedList;
import java.util.List;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.eclipse.core.runtime.FileLocator;
import org.fosstrak.llrp.commander.LLRPPlugin;
import org.llrp.ltkGenerator.CodeGenerator;
import org.llrp.ltkGenerator.generated.*;
import org.w3c.dom.Element;
/**
* This class can be used to get information on the LLRP protocol.
* For example, to learn the definition of the "ADD_ROSPEC" message, call
* LLRP.getMessageDefinition("ADD_ROSPEC").
*
* @author Ulrich Etter, ETHZ
*
*/
public class LLRP {
static {
String jaxBPackage = "org.llrp.ltkGenerator.generated";
String pluginPath = null;
URL bundleRootURL = LLRPPlugin.getDefault().getBundle().getEntry("/");
URL pluginURL;
try {
pluginURL = FileLocator.resolve(bundleRootURL);
pluginPath = pluginURL.getPath();
} catch (IOException e) {
e.printStackTrace();
}
pluginPath = "file://" + pluginPath;
String namespacePrefix = "llrp";
String xmlPath = pluginPath + "Definitions/Core/llrp-1x0-def.xml";
String xsdPath = pluginPath + "Definitions/Core/llrp-1x0.xsd";
String[] extensions = {namespacePrefix + ";" + xmlPath + ";" + xsdPath};
CodeGenerator cg = new CodeGenerator();
llrpDefinition = cg.getLLRPDefinition(jaxBPackage, extensions);
}
private static LlrpDefinition llrpDefinition;
/**
* Returns the LLRP definition object.
*
* @return the LLRP definition object
*/
public static LlrpDefinition getLlrpDefintion(){
return llrpDefinition;
}
/**
* Returns the definition of the message with the given name.
* @param messageName the name of a message
* @return the definition object, or null
if no message with the given name exists
*/
public static MessageDefinition getMessageDefinition(String messageName) {
MessageDefinition messageDefinition = null;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy