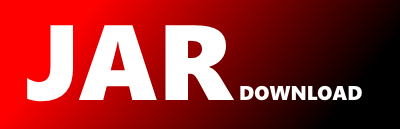
org.freedesktop.wayland.util.Arguments Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stubs Show documentation
Show all versions of stubs Show documentation
Wayland protocol Java-language Binding Stubs
//Copyright 2015 Erik De Rijcke
//
//Licensed under the Apache License,Version2.0(the"License");
//you may not use this file except in compliance with the License.
//You may obtain a copy of the License at
//
//http://www.apache.org/licenses/LICENSE-2.0
//
//Unless required by applicable law or agreed to in writing,software
//distributed under the License is distributed on an"AS IS"BASIS,
//WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,either express or implied.
//See the License for the specific language governing permissions and
//limitations under the License.
package org.freedesktop.wayland.util;
import org.freedesktop.jaccall.Pointer;
import org.freedesktop.wayland.client.Proxy;
import org.freedesktop.wayland.server.Resource;
import org.freedesktop.wayland.util.jaccall.wl_argument;
import org.freedesktop.wayland.util.jaccall.wl_array;
import java.nio.ByteBuffer;
import java.util.LinkedList;
import java.util.List;
import static org.freedesktop.jaccall.Pointer.malloc;
import static org.freedesktop.jaccall.Pointer.nref;
import static org.freedesktop.jaccall.Pointer.ref;
import static org.freedesktop.jaccall.Pointer.wrap;
public class Arguments {
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy