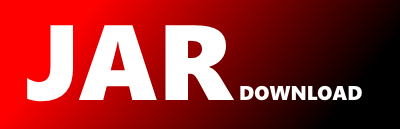
org.freedesktop.wayland.client.WlRegistryProxy Maven / Gradle / Ivy
Show all versions of wayland Show documentation
package org.freedesktop.wayland.client;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.freedesktop.wayland.util.Arguments;
import org.freedesktop.wayland.util.Interface;
import org.freedesktop.wayland.util.Message;
//
//
// Copyright © 2008-2011 Kristian Høgsberg
// Copyright © 2010-2011 Intel Corporation
// Copyright © 2012-2013 Collabora, Ltd.
//
// Permission to use, copy, modify, distribute, and sell this
// software and its documentation for any purpose is hereby granted
// without fee, provided that the above copyright notice appear in
// all copies and that both that copyright notice and this permission
// notice appear in supporting documentation, and that the name of
// the copyright holders not be used in advertising or publicity
// pertaining to distribution of the software without specific,
// written prior permission. The copyright holders make no
// representations about the suitability of this software for any
// purpose. It is provided "as is" without express or implied
// warranty.
//
// THE COPYRIGHT HOLDERS DISCLAIM ALL WARRANTIES WITH REGARD TO THIS
// SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
// FITNESS, IN NO EVENT SHALL THE COPYRIGHT HOLDERS BE LIABLE FOR ANY
// SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN
// AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION,
// ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF
// THIS SOFTWARE.
//
/**
* global registry object
*
*
* The global registry object. The server has a number of global
* objects that are available to all clients. These objects
* typically represent an actual object in the server (for example,
* an input device) or they are singleton objects that provide
* extension functionality.
*
* When a client creates a registry object, the registry object
* will emit a global event for each global currently in the
* registry. Globals come and go as a result of device or
* monitor hotplugs, reconfiguration or other events, and the
* registry will send out global and global_remove events to
* keep the client up to date with the changes. To mark the end
* of the initial burst of events, the client can use the
* wl_display.sync request immediately after calling
* wl_display.get_registry.
*
* A client can bind to a global object by using the bind
* request. This creates a client-side handle that lets the object
* emit events to the client and lets the client invoke requests on
* the object.
*
*/
@Interface(
methods = {
@Message(
types = {
int.class,
org.freedesktop.wayland.client.Proxy.class
},
signature = "usun",
functionName = "bind",
name = "bind"
)
},
name = "wl_registry",
version = 1,
events = {
@Message(
types = {
int.class,
java.lang.String.class,
int.class
},
signature = "usu",
functionName = "global",
name = "global"
)
,
@Message(
types = {
int.class
},
signature = "u",
functionName = "globalRemove",
name = "global_remove"
)
}
)
public class WlRegistryProxy extends Proxy {
public static final String INTERFACE_NAME = "wl_registry";
public WlRegistryProxy(com.sun.jna.Pointer pointer, WlRegistryEvents implementation, int version) {
super(pointer, implementation, version);
}
public WlRegistryProxy(com.sun.jna.Pointer pointer) {
super(pointer);
}
/**
* bind an object to the display
*
*
* Binds a new, client-created object to the server using the
* specified name as the identifier.
*
* @param name unique name for the object
* @param proxyType The type of proxy to create. Must be a subclass of Proxy.
* @param version The protocol version to use. Must not be higher than what the supplied implementation can support.
* @param implementation A protocol event listener for the newly created proxy.
*/
public > T bind(int name, Class proxyType, int version, J implementation) {
return marshalConstructor(0, implementation, version, proxyType, Arguments.create(4).set(0, name).set(1, proxyType.getAnnotation(Interface.class).name()).set(2, version).set(3, 0));
}
}