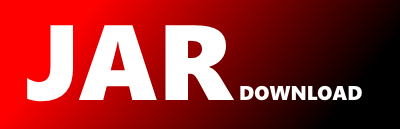
org.freedesktop.wayland.client.WlShellSurfaceProxy Maven / Gradle / Ivy
Show all versions of wayland Show documentation
package org.freedesktop.wayland.client;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.freedesktop.wayland.util.Arguments;
import org.freedesktop.wayland.util.Interface;
import org.freedesktop.wayland.util.Message;
//
//
// Copyright © 2008-2011 Kristian Høgsberg
// Copyright © 2010-2011 Intel Corporation
// Copyright © 2012-2013 Collabora, Ltd.
//
// Permission to use, copy, modify, distribute, and sell this
// software and its documentation for any purpose is hereby granted
// without fee, provided that the above copyright notice appear in
// all copies and that both that copyright notice and this permission
// notice appear in supporting documentation, and that the name of
// the copyright holders not be used in advertising or publicity
// pertaining to distribution of the software without specific,
// written prior permission. The copyright holders make no
// representations about the suitability of this software for any
// purpose. It is provided "as is" without express or implied
// warranty.
//
// THE COPYRIGHT HOLDERS DISCLAIM ALL WARRANTIES WITH REGARD TO THIS
// SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
// FITNESS, IN NO EVENT SHALL THE COPYRIGHT HOLDERS BE LIABLE FOR ANY
// SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN
// AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION,
// ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF
// THIS SOFTWARE.
//
/**
* desktop-style metadata interface
*
*
* An interface that may be implemented by a wl_surface, for
* implementations that provide a desktop-style user interface.
*
* It provides requests to treat surfaces like toplevel, fullscreen
* or popup windows, move, resize or maximize them, associate
* metadata like title and class, etc.
*
* On the server side the object is automatically destroyed when
* the related wl_surface is destroyed. On client side,
* wl_shell_surface_destroy() must be called before destroying
* the wl_surface object.
*
*/
@Interface(
methods = {
@Message(
types = {
int.class
},
signature = "u",
functionName = "pong",
name = "pong"
)
,
@Message(
types = {
org.freedesktop.wayland.client.WlSeatProxy.class,
int.class
},
signature = "ou",
functionName = "move",
name = "move"
)
,
@Message(
types = {
org.freedesktop.wayland.client.WlSeatProxy.class,
int.class,
int.class
},
signature = "ouu",
functionName = "resize",
name = "resize"
)
,
@Message(
types = {
},
signature = "",
functionName = "setToplevel",
name = "set_toplevel"
)
,
@Message(
types = {
org.freedesktop.wayland.client.WlSurfaceProxy.class,
int.class,
int.class,
int.class
},
signature = "oiiu",
functionName = "setTransient",
name = "set_transient"
)
,
@Message(
types = {
int.class,
int.class,
org.freedesktop.wayland.client.WlOutputProxy.class
},
signature = "uu?o",
functionName = "setFullscreen",
name = "set_fullscreen"
)
,
@Message(
types = {
org.freedesktop.wayland.client.WlSeatProxy.class,
int.class,
org.freedesktop.wayland.client.WlSurfaceProxy.class,
int.class,
int.class,
int.class
},
signature = "ouoiiu",
functionName = "setPopup",
name = "set_popup"
)
,
@Message(
types = {
org.freedesktop.wayland.client.WlOutputProxy.class
},
signature = "?o",
functionName = "setMaximized",
name = "set_maximized"
)
,
@Message(
types = {
java.lang.String.class
},
signature = "s",
functionName = "setTitle",
name = "set_title"
)
,
@Message(
types = {
java.lang.String.class
},
signature = "s",
functionName = "setClass",
name = "set_class"
)
},
name = "wl_shell_surface",
version = 1,
events = {
@Message(
types = {
int.class
},
signature = "u",
functionName = "ping",
name = "ping"
)
,
@Message(
types = {
int.class,
int.class,
int.class
},
signature = "uii",
functionName = "configure",
name = "configure"
)
,
@Message(
types = {
},
signature = "",
functionName = "popupDone",
name = "popup_done"
)
}
)
public class WlShellSurfaceProxy extends Proxy {
public static final String INTERFACE_NAME = "wl_shell_surface";
public WlShellSurfaceProxy(com.sun.jna.Pointer pointer, WlShellSurfaceEvents implementation, int version) {
super(pointer, implementation, version);
}
public WlShellSurfaceProxy(com.sun.jna.Pointer pointer) {
super(pointer);
}
/**
* respond to a ping event
*
*
* A client must respond to a ping event with a pong request or
* the client may be deemed unresponsive.
*
* @param serial serial of the ping event
*/
public void pong(int serial) {
marshal(0, Arguments.create(1).set(0, serial));
}
/**
* start an interactive move
*
*
* Start a pointer-driven move of the surface.
*
* This request must be used in response to a button press event.
* The server may ignore move requests depending on the state of
* the surface (e.g. fullscreen or maximized).
*
* @param seat the wl_seat whose pointer is used
* @param serial serial of the implicit grab on the pointer
*/
public void move(@Nonnull WlSeatProxy seat, int serial) {
marshal(1, Arguments.create(2).set(0, seat).set(1, serial));
}
/**
* start an interactive resize
*
*
* Start a pointer-driven resizing of the surface.
*
* This request must be used in response to a button press event.
* The server may ignore resize requests depending on the state of
* the surface (e.g. fullscreen or maximized).
*
* @param seat the wl_seat whose pointer is used
* @param serial serial of the implicit grab on the pointer
* @param edges which edge or corner is being dragged
*/
public void resize(@Nonnull WlSeatProxy seat, int serial, int edges) {
marshal(2, Arguments.create(3).set(0, seat).set(1, serial).set(2, edges));
}
/**
* make the surface a toplevel surface
*
*
* Map the surface as a toplevel surface.
*
* A toplevel surface is not fullscreen, maximized or transient.
*
*/
public void setToplevel() {
marshal(3);
}
/**
* make the surface a transient surface
*
*
* Map the surface relative to an existing surface.
*
* The x and y arguments specify the locations of the upper left
* corner of the surface relative to the upper left corner of the
* parent surface, in surface local coordinates.
*
* The flags argument controls details of the transient behaviour.
*
* @param parent
* @param x
* @param y
* @param flags
*/
public void setTransient(@Nonnull WlSurfaceProxy parent, int x, int y, int flags) {
marshal(4, Arguments.create(4).set(0, parent).set(1, x).set(2, y).set(3, flags));
}
/**
* make the surface a fullscreen surface
*
*
* Map the surface as a fullscreen surface.
*
* If an output parameter is given then the surface will be made
* fullscreen on that output. If the client does not specify the
* output then the compositor will apply its policy - usually
* choosing the output on which the surface has the biggest surface
* area.
*
* The client may specify a method to resolve a size conflict
* between the output size and the surface size - this is provided
* through the method parameter.
*
* The framerate parameter is used only when the method is set
* to "driver", to indicate the preferred framerate. A value of 0
* indicates that the app does not care about framerate. The
* framerate is specified in mHz, that is framerate of 60000 is 60Hz.
*
* A method of "scale" or "driver" implies a scaling operation of
* the surface, either via a direct scaling operation or a change of
* the output mode. This will override any kind of output scaling, so
* that mapping a surface with a buffer size equal to the mode can
* fill the screen independent of buffer_scale.
*
* A method of "fill" means we don't scale up the buffer, however
* any output scale is applied. This means that you may run into
* an edge case where the application maps a buffer with the same
* size of the output mode but buffer_scale 1 (thus making a
* surface larger than the output). In this case it is allowed to
* downscale the results to fit the screen.
*
* The compositor must reply to this request with a configure event
* with the dimensions for the output on which the surface will
* be made fullscreen.
*
* @param method
* @param framerate
* @param output
*/
public void setFullscreen(int method, int framerate, @Nullable WlOutputProxy output) {
marshal(5, Arguments.create(3).set(0, method).set(1, framerate).set(2, output));
}
/**
* make the surface a popup surface
*
*
* Map the surface as a popup.
*
* A popup surface is a transient surface with an added pointer
* grab.
*
* An existing implicit grab will be changed to owner-events mode,
* and the popup grab will continue after the implicit grab ends
* (i.e. releasing the mouse button does not cause the popup to
* be unmapped).
*
* The popup grab continues until the window is destroyed or a
* mouse button is pressed in any other clients window. A click
* in any of the clients surfaces is reported as normal, however,
* clicks in other clients surfaces will be discarded and trigger
* the callback.
*
* The x and y arguments specify the locations of the upper left
* corner of the surface relative to the upper left corner of the
* parent surface, in surface local coordinates.
*
* @param seat the wl_seat whose pointer is used
* @param serial serial of the implicit grab on the pointer
* @param parent
* @param x
* @param y
* @param flags
*/
public void setPopup(@Nonnull WlSeatProxy seat, int serial, @Nonnull WlSurfaceProxy parent, int x, int y, int flags) {
marshal(6, Arguments.create(6).set(0, seat).set(1, serial).set(2, parent).set(3, x).set(4, y).set(5, flags));
}
/**
* make the surface a maximized surface
*
*
* Map the surface as a maximized surface.
*
* If an output parameter is given then the surface will be
* maximized on that output. If the client does not specify the
* output then the compositor will apply its policy - usually
* choosing the output on which the surface has the biggest surface
* area.
*
* The compositor will reply with a configure event telling
* the expected new surface size. The operation is completed
* on the next buffer attach to this surface.
*
* A maximized surface typically fills the entire output it is
* bound to, except for desktop element such as panels. This is
* the main difference between a maximized shell surface and a
* fullscreen shell surface.
*
* The details depend on the compositor implementation.
*
* @param output
*/
public void setMaximized(@Nullable WlOutputProxy output) {
marshal(7, Arguments.create(1).set(0, output));
}
/**
* set surface title
*
*
* Set a short title for the surface.
*
* This string may be used to identify the surface in a task bar,
* window list, or other user interface elements provided by the
* compositor.
*
* The string must be encoded in UTF-8.
*
* @param title
*/
public void setTitle(@Nonnull String title) {
marshal(8, Arguments.create(1).set(0, title));
}
/**
* set surface class
*
*
* Set a class for the surface.
*
* The surface class identifies the general class of applications
* to which the surface belongs. A common convention is to use the
* file name (or the full path if it is a non-standard location) of
* the application's .desktop file as the class.
*
* @param class_
*/
public void setClass(@Nonnull String class_) {
marshal(9, Arguments.create(1).set(0, class_));
}
}