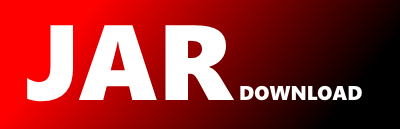
org.freedesktop.wayland.client.WlTouchEventsV2 Maven / Gradle / Ivy
Show all versions of wayland Show documentation
package org.freedesktop.wayland.client;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
//
//
// Copyright © 2008-2011 Kristian Høgsberg
// Copyright © 2010-2011 Intel Corporation
// Copyright © 2012-2013 Collabora, Ltd.
//
// Permission to use, copy, modify, distribute, and sell this
// software and its documentation for any purpose is hereby granted
// without fee, provided that the above copyright notice appear in
// all copies and that both that copyright notice and this permission
// notice appear in supporting documentation, and that the name of
// the copyright holders not be used in advertising or publicity
// pertaining to distribution of the software without specific,
// written prior permission. The copyright holders make no
// representations about the suitability of this software for any
// purpose. It is provided "as is" without express or implied
// warranty.
//
// THE COPYRIGHT HOLDERS DISCLAIM ALL WARRANTIES WITH REGARD TO THIS
// SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
// FITNESS, IN NO EVENT SHALL THE COPYRIGHT HOLDERS BE LIABLE FOR ANY
// SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
// WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN
// AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION,
// ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF
// THIS SOFTWARE.
//
/**
* touchscreen input device
*
*
* The wl_touch interface represents a touchscreen
* associated with a seat.
*
* Touch interactions can consist of one or more contacts.
* For each contact, a series of events is generated, starting
* with a down event, followed by zero or more motion events,
* and ending with an up event. Events relating to the same
* contact point can be identified by the ID of the sequence.
*
*/
public interface WlTouchEventsV2 extends WlTouchEvents {
int VERSION = 2;
/**
* touch down event and beginning of a touch sequence
*
*
* A new touch point has appeared on the surface. This touch point is
* assigned a unique @id. Future events from this touchpoint reference
* this ID. The ID ceases to be valid after a touch up event and may be
* re-used in the future.
*
* @param emitter The protocol object that emitted the event.
* @param serial
* @param time timestamp with millisecond granularity
* @param surface
* @param id the unique ID of this touch point
* @param x x coordinate in surface-relative coordinates
* @param y y coordinate in surface-relative coordinates
*/
public void down(WlTouchProxy emitter, int serial, int time, @Nonnull WlSurfaceProxy surface, int id, @Nonnull org.freedesktop.wayland.util.Fixed x, @Nonnull org.freedesktop.wayland.util.Fixed y);
/**
* end of a touch event sequence
*
*
* The touch point has disappeared. No further events will be sent for
* this touchpoint and the touch point's ID is released and may be
* re-used in a future touch down event.
*
* @param emitter The protocol object that emitted the event.
* @param serial
* @param time timestamp with millisecond granularity
* @param id the unique ID of this touch point
*/
public void up(WlTouchProxy emitter, int serial, int time, int id);
/**
* update of touch point coordinates
*
*
* A touchpoint has changed coordinates.
*
* @param emitter The protocol object that emitted the event.
* @param time timestamp with millisecond granularity
* @param id the unique ID of this touch point
* @param x x coordinate in surface-relative coordinates
* @param y y coordinate in surface-relative coordinates
*/
public void motion(WlTouchProxy emitter, int time, int id, @Nonnull org.freedesktop.wayland.util.Fixed x, @Nonnull org.freedesktop.wayland.util.Fixed y);
/**
* end of touch frame event
*
*
* Indicates the end of a contact point list.
*
* @param emitter The protocol object that emitted the event.
*/
public void frame(WlTouchProxy emitter);
/**
* touch session cancelled
*
*
* Sent if the compositor decides the touch stream is a global
* gesture. No further events are sent to the clients from that
* particular gesture. Touch cancellation applies to all touch points
* currently active on this client's surface. The client is
* responsible for finalizing the touch points, future touch points on
* this surface may re-use the touch point ID.
*
* @param emitter The protocol object that emitted the event.
*/
public void cancel(WlTouchProxy emitter);
}