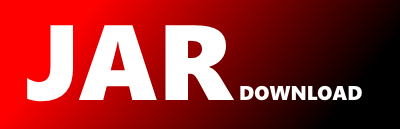
org.freehep.util.io.DecodingInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freehep-io Show documentation
Show all versions of freehep-io Show documentation
FreeHEP extension to the java.io library
// Copyright FreeHEP, 2009
package org.freehep.util.io;
import java.io.IOException;
import java.io.InputStream;
/**
* Handles read by always throwing IOExceptions even in the middle of a read of
* an array. IMPORTANT: inherits from InputStream rather than FilterInputStream
* so that the correct read(byte[], int, int) method is used.
*
* @author Mark Donszelmann ([email protected])
*/
public abstract class DecodingInputStream extends InputStream {
/**
* Overridden to make sure it ALWAYS throws an IOException while a problem
* occurs in read().
*/
@Override
public int read(byte b[], int off, int len) throws IOException {
if (b == null) {
throw new NullPointerException();
} else if (off < 0 || len < 0 || len > b.length - off) {
throw new IndexOutOfBoundsException();
} else if (len == 0) {
return 0;
}
int c = read();
if (c == -1) {
return -1;
}
b[off] = (byte) c;
int i = 1;
for (; i < len; i++) {
c = read();
if (c == -1) {
break;
}
b[off + i] = (byte) c;
}
return i;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy