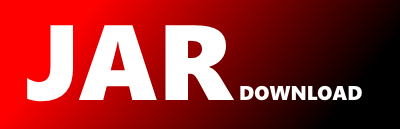
org.friendularity.bundle.bento.gui.MergeGridGlassPane Maven / Gradle / Ivy
/*
* Copyright 2013 by The Friendularity Project (www.friendularity.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.friendularity.bundle.bento.gui;
import java.awt.Color;
import java.awt.Component;
import java.awt.Cursor;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.event.MouseMotionListener;
import java.util.Iterator;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JPopupMenu;
import javax.swing.JSeparator;
import javax.swing.SwingUtilities;
import org.friendularity.bundle.bento.util.Bento_OSGi_ResourceLoader;
import org.friendularity.jvision.broker.ImageStreamBroker;
/**
*
* @author Annie
*/
class MergeGridGlassPane extends JPanel implements MouseListener, MouseMotionListener {
private boolean iHandleEvents = false;
private int newComponentCol;
private int newComponentRow;
public MergeGridGlassPane() {
this.setOpaque(false);
this.setDoubleBuffered(false);
this.addMouseListener(this);
this.addMouseMotionListener(this);
}
@Override
public boolean isOptimizedDrawingEnabled() {
return false; // ensures that z order happens properly
}
@Override
protected void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D)g;
if(isHorSplitDragging)
{
g2.setColor(new Color(128, 128,128, 128));
g2.fillRect(curHorSplitterWidgetX, 0, MergeGrid.SEPARATOR_WIDTH, this.getHeight());
}
if(isVertSplitDragging)
{
g2.setColor(new Color(128, 128,128, 128));
g2.fillRect(0, curVertSplitterWidgetY, this.getWidth(), MergeGrid.SEPARATOR_HEIGHT);
}
/*
g2.setColor(Color.red);
String[] s = debugStr.split(":");
int yy = this.getHeight() / 2;
for(int i = 0; i 1)
{
menuItem = new JMenuItem(DELETE_COLUMN);
menuItem.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e) {
MergeGridGlassPane.this.draggingComponent = null;
((MergeGrid)(MergeGridGlassPane.this.getParent())).attemptDeleteColumn(col);
}
});
popup.add(menuItem);
}
if(((MergeGrid)(MergeGridGlassPane.this.getParent())).getNumRows() > 1)
{
menuItem = new JMenuItem(DELETE_ROW);
menuItem.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e) {
MergeGridGlassPane.this.draggingComponent = null;
((MergeGrid)(MergeGridGlassPane.this.getParent())).attemptDeleteRow(row);
}
});
popup.add(menuItem);
}
}
if (c instanceof BentoPlugin)
{
popup.add(new JSeparator());
menuItem = new JMenuItem(REMOVE_MENU);
menuItem.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e) {
draggingComponent = null;
((BentoPlugin)c).removeFromMergeGrid();
}
});
popup.add(menuItem);
}
if (c == null)
{
popup.add(new JSeparator());
subMenu = new JMenu("Add Item");
for(Iterator i = ImageStreamBroker.getDefaultImageStreamBroker().imageStreamNames() ;
i.hasNext() ; )
{
String name = i.next();
menuItem = new JMenuItem(name);
menuItem.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e) {
MergeGridGlassPane.this.addElementAction(e);
}
});
subMenu.add(menuItem);
}
popup.add(subMenu);
}
if (c instanceof BentoPlugin)
{
((BentoPlugin)c).addAdditionalMenuItems(popup);
}
}
private JPopupMenu popup;
/**
* get the right click menu
*
* If you modify it, do it on swingworker thread
*
* @return popup menu
*/
protected JPopupMenu getPopup()
{
return popup;
}
private String debugStr = "";
/**
* print
* @param handleEventLocally
* @return
*/
private boolean debugEvent(MouseEvent e, boolean handledLocally) {
debugStr = e.toString() + ":";
if(iHandleEvents)debugStr += "iHandleEvents:";
if(isHorSplitDragging)debugStr += "isHorSplitDragging:";
if(isVertSplitDragging)debugStr += "isVertSplitDragging:";
if(draggingComponent != null)debugStr += draggingComponent.getClass().getSimpleName();
this.repaint(50L);
return handledLocally;
}
private void addElementAction(ActionEvent e) {
MergeGrid mg = ((MergeGrid)(MergeGridGlassPane.this.getParent()));
CameraViewer cv = new CameraViewer(e.getActionCommand());
try {
mg.setCell(cv, newComponentCol, newComponentRow, 1, 1);
} catch (ItsBentoBoxesNotBentoTetrisException ex) {
Logger.getLogger(BentoFrame.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy