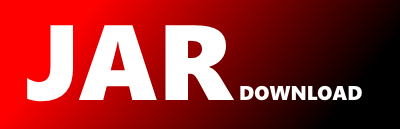
org.opencv.calib3d.StereoBM Maven / Gradle / Ivy
// // This file is auto-generated. Please don't modify it! // package org.opencv.calib3d; import org.opencv.core.Mat; // C++: class StereoBM /** *
* *Class for computing stereo correspondence using the block matching algorithm.
* *// Block matching stereo correspondence algorithm class StereoBM
* *// C++ code:
* * *enum { NORMALIZED_RESPONSE = CV_STEREO_BM_NORMALIZED_RESPONSE,
* *BASIC_PRESET=CV_STEREO_BM_BASIC,
* *FISH_EYE_PRESET=CV_STEREO_BM_FISH_EYE,
* *NARROW_PRESET=CV_STEREO_BM_NARROW };
* *StereoBM();
* *// the preset is one of..._PRESET above.
* *// ndisparities is the size of disparity range,
* *// in which the optimal disparity at each pixel is searched for.
* *// SADWindowSize is the size of averaging window used to match pixel blocks
* *// (larger values mean better robustness to noise, but yield blurry disparity * maps)
* *StereoBM(int preset, int ndisparities=0, int SADWindowSize=21);
* *// separate initialization function
* *void init(int preset, int ndisparities=0, int SADWindowSize=21);
* *// computes the disparity for the two rectified 8-bit single-channel images.
* *// the disparity will be 16-bit signed (fixed-point) or 32-bit floating-point * image of the same size as left.
* *void operator()(InputArray left, InputArray right, OutputArray disparity, int * disptype=CV_16S);
* *Ptr
* *state; };
* *The class is a C++ wrapper for the associated functions. In particular, * :ocv:funcx:"StereoBM.operator()" is the wrapper for
"cvFindStereoCorrespondenceBM".
* * @see org.opencv.calib3d.StereoBM */ public class StereoBM { protected final long nativeObj; protected StereoBM(long addr) { nativeObj = addr; } public static final int PREFILTER_NORMALIZED_RESPONSE = 0, PREFILTER_XSOBEL = 1, BASIC_PRESET = 0, FISH_EYE_PRESET = 1, NARROW_PRESET = 2; // // C++: StereoBM::StereoBM() // /** *The constructors.
* *The constructors initialize
* *StereoBM
state. You can then call *StereoBM.operator()
to compute disparity for a specific stereo * pair.Note: In the C API you need to deallocate
* * @see org.opencv.calib3d.StereoBM.StereoBM */ public StereoBM() { nativeObj = StereoBM_0(); return; } // // C++: StereoBM::StereoBM(int preset, int ndisparities = 0, int SADWindowSize = 21) // /** *CvStereoBM
state when * it is not needed anymore usingcvReleaseStereoBMState(&stereobm)
.The constructors.
* *The constructors initialize
* *StereoBM
state. You can then call *StereoBM.operator()
to compute disparity for a specific stereo * pair.Note: In the C API you need to deallocate
* * @param preset specifies the whole set of algorithm parameters, one of: *CvStereoBM
state when * it is not needed anymore usingcvReleaseStereoBMState(&stereobm)
.
-
*
- BASIC_PRESET - parameters suitable for general cameras *
- FISH_EYE_PRESET - parameters suitable for wide-angle cameras *
- NARROW_PRESET - parameters suitable for narrow-angle cameras *
After constructing the class, you can override any parameters set by the * preset.
* @param ndisparities the disparity search range. For each pixel algorithm will * find the best disparity from 0 (default minimum disparity) to *ndisparities
. The search range can then be shifted by changing
* the minimum disparity.
* @param SADWindowSize the linear size of the blocks compared by the algorithm.
* The size should be odd (as the block is centered at the current pixel).
* Larger block size implies smoother, though less accurate disparity map.
* Smaller block size gives more detailed disparity map, but there is higher
* chance for algorithm to find a wrong correspondence.
*
* @see org.opencv.calib3d.StereoBM.StereoBM
*/
public StereoBM(int preset, int ndisparities, int SADWindowSize)
{
nativeObj = StereoBM_1(preset, ndisparities, SADWindowSize);
return;
}
/**
* The constructors.
* *The constructors initialize StereoBM
state. You can then call
* StereoBM.operator()
to compute disparity for a specific stereo
* pair.
Note: In the C API you need to deallocate CvStereoBM
state when
* it is not needed anymore using cvReleaseStereoBMState(&stereobm)
.
-
*
- BASIC_PRESET - parameters suitable for general cameras *
- FISH_EYE_PRESET - parameters suitable for wide-angle cameras *
- NARROW_PRESET - parameters suitable for narrow-angle cameras *
After constructing the class, you can override any parameters set by the * preset.
* * @see org.opencv.calib3d.StereoBM.StereoBM */ public StereoBM(int preset) { nativeObj = StereoBM_2(preset); return; } // // C++: void StereoBM::operator ()(Mat left, Mat right, Mat& disparity, int disptype = CV_16S) // /** *Computes disparity using the BM algorithm for a rectified stereo pair.
* *The method executes the BM algorithm on a rectified stereo pair. See the
* stereo_match.cpp
OpenCV sample on how to prepare images and call
* the method. Note that the method is not constant, thus you should not use the
* same StereoBM
instance from within different threads
* simultaneously. The function is parallelized with the TBB library.
disptype==CV_16S
, the map is a 16-bit signed
* single-channel image, containing disparity values scaled by 16. To get the
* true disparity values from such fixed-point representation, you will need to
* divide each disp
element by 16. If disptype==CV_32F
,
* the disparity map will already contain the real disparity values on output.
* @param disptype Type of the output disparity map, CV_16S
* (default) or CV_32F
.
*
* @see org.opencv.calib3d.StereoBM.operator()
*/
public void compute(Mat left, Mat right, Mat disparity, int disptype)
{
compute_0(nativeObj, left.nativeObj, right.nativeObj, disparity.nativeObj, disptype);
return;
}
/**
* Computes disparity using the BM algorithm for a rectified stereo pair.
* *The method executes the BM algorithm on a rectified stereo pair. See the
* stereo_match.cpp
OpenCV sample on how to prepare images and call
* the method. Note that the method is not constant, thus you should not use the
* same StereoBM
instance from within different threads
* simultaneously. The function is parallelized with the TBB library.
disptype==CV_16S
, the map is a 16-bit signed
* single-channel image, containing disparity values scaled by 16. To get the
* true disparity values from such fixed-point representation, you will need to
* divide each disp
element by 16. If disptype==CV_32F
,
* the disparity map will already contain the real disparity values on output.
*
* @see org.opencv.calib3d.StereoBM.operator()
*/
public void compute(Mat left, Mat right, Mat disparity)
{
compute_1(nativeObj, left.nativeObj, right.nativeObj, disparity.nativeObj);
return;
}
@Override
protected void finalize() throws Throwable {
delete(nativeObj);
}
// C++: StereoBM::StereoBM()
private static native long StereoBM_0();
// C++: StereoBM::StereoBM(int preset, int ndisparities = 0, int SADWindowSize = 21)
private static native long StereoBM_1(int preset, int ndisparities, int SADWindowSize);
private static native long StereoBM_2(int preset);
// C++: void StereoBM::operator ()(Mat left, Mat right, Mat& disparity, int disptype = CV_16S)
private static native void compute_0(long nativeObj, long left_nativeObj, long right_nativeObj, long disparity_nativeObj, int disptype);
private static native void compute_1(long nativeObj, long left_nativeObj, long right_nativeObj, long disparity_nativeObj);
// native support for java finalize()
private static native void delete(long nativeObj);
}