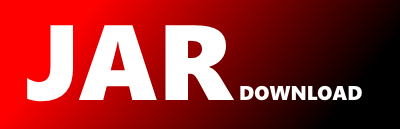
org.friendularity.gen.reacted.percept.MusicToken Maven / Gradle / Ivy
/*
* generated by http://RDFReactor.semweb4j.org ($Id: CodeGenerator.java 1954 2014-10-29 14:09:16Z [email protected] $) on 5/5/15 7:51 PM
*/
package org.friendularity.gen.reacted.percept;
import org.ontoware.aifbcommons.collection.ClosableIterator;
import org.ontoware.rdf2go.exception.ModelRuntimeException;
import org.ontoware.rdf2go.model.Model;
import org.ontoware.rdf2go.model.node.BlankNode;
import org.ontoware.rdf2go.model.node.Node;
import org.ontoware.rdf2go.model.node.Resource;
import org.ontoware.rdf2go.model.node.URI;
import org.ontoware.rdf2go.model.node.impl.URIImpl;
import org.ontoware.rdfreactor.runtime.Base;
import org.ontoware.rdfreactor.runtime.ReactorResult;
/**
*
* This class was generated by RDFReactor on 5/5/15 7:51 PM
*/
public class MusicToken extends Token {
private static final long serialVersionUID = 5289094405873273623L;
/** http://onto.coghcar.org/onto/201406/PerceptObsEstim_OWL2#MusicToken */
public static final URI RDFS_CLASS = new URIImpl("http://onto.coghcar.org/onto/201406/PerceptObsEstim_OWL2#MusicToken", false);
/**
* All property-URIs with this class as domain.
* All properties of all super-classes are also available.
*/
public static final URI[] MANAGED_URIS = {
};
// protected constructors needed for inheritance
/**
* Returns a Java wrapper over an RDF object, identified by URI.
* Creating two wrappers for the same instanceURI is legal.
* @param model RDF2GO Model implementation, see http://rdf2go.semweb4j.org
* @param classURI URI of RDFS class
* @param instanceIdentifier Resource that identifies this instance
* @param write if true, the statement (this, rdf:type, TYPE) is written to the model
*
* [Generated from RDFReactor template rule #c1]
*/
protected MusicToken (Model model, URI classURI, Resource instanceIdentifier, boolean write) {
super(model, classURI, instanceIdentifier, write);
}
// public constructors
/**
* Returns a Java wrapper over an RDF object, identified by URI.
* Creating two wrappers for the same instanceURI is legal.
* @param model RDF2GO Model implementation, see http://rdf2go.ontoware.org
* @param instanceIdentifier an RDF2Go Resource identifying this instance
* @param write if true, the statement (this, rdf:type, TYPE) is written to the model
*
* [Generated from RDFReactor template rule #c2]
*/
public MusicToken (Model model, Resource instanceIdentifier, boolean write) {
super(model, RDFS_CLASS, instanceIdentifier, write);
}
/**
* Returns a Java wrapper over an RDF object, identified by a URI, given as a String.
* Creating two wrappers for the same URI is legal.
* @param model RDF2GO Model implementation, see http://rdf2go.ontoware.org
* @param uriString a URI given as a String
* @param write if true, the statement (this, rdf:type, TYPE) is written to the model
* @throws ModelRuntimeException if URI syntax is wrong
*
* [Generated from RDFReactor template rule #c7]
*/
public MusicToken (Model model, String uriString, boolean write) throws ModelRuntimeException {
super(model, RDFS_CLASS, new URIImpl(uriString,false), write);
}
/**
* Returns a Java wrapper over an RDF object, identified by a blank node.
* Creating two wrappers for the same blank node is legal.
* @param model RDF2GO Model implementation, see http://rdf2go.ontoware.org
* @param bnode BlankNode of this instance
* @param write if true, the statement (this, rdf:type, TYPE) is written to the model
*
* [Generated from RDFReactor template rule #c8]
*/
public MusicToken (Model model, BlankNode bnode, boolean write) {
super(model, RDFS_CLASS, bnode, write);
}
/**
* Returns a Java wrapper over an RDF object, identified by
* a randomly generated URI.
* Creating two wrappers results in different URIs.
* @param model RDF2GO Model implementation, see http://rdf2go.ontoware.org
* @param write if true, the statement (this, rdf:type, TYPE) is written to the model
*
* [Generated from RDFReactor template rule #c9]
*/
public MusicToken (Model model, boolean write) {
super(model, RDFS_CLASS, model.newRandomUniqueURI(), write);
}
///////////////////////////////////////////////////////////////////
// typing
/**
* Return an existing instance of this class in the model. No statements are written.
* @param model an RDF2Go model
* @param instanceResource an RDF2Go resource
* @return an instance of MusicToken or null if none existst
*
* [Generated from RDFReactor template rule #class0]
*/
public static MusicToken getInstance(Model model, Resource instanceResource) {
return Base.getInstance(model, instanceResource, MusicToken.class);
}
/**
* Create a new instance of this class in the model.
* That is, create the statement (instanceResource, RDF.type, http://onto.coghcar.org/onto/201406/PerceptObsEstim_OWL2#MusicToken).
* @param model an RDF2Go model
* @param instanceResource an RDF2Go resource
*
* [Generated from RDFReactor template rule #class1]
*/
public static void createInstance(Model model, Resource instanceResource) {
Base.createInstance(model, RDFS_CLASS, instanceResource);
}
/**
* @param model an RDF2Go model
* @param instanceResource an RDF2Go resource
* @return true if instanceResource is an instance of this class in the model
*
* [Generated from RDFReactor template rule #class2]
*/
public static boolean hasInstance(Model model, Resource instanceResource) {
return Base.hasInstance(model, RDFS_CLASS, instanceResource);
}
/**
* @param model an RDF2Go model
* @return all instances of this class in Model 'model' as RDF resources
*
* [Generated from RDFReactor template rule #class3]
*/
public static ClosableIterator getAllInstances(Model model) {
return Base.getAllInstances(model, RDFS_CLASS, Resource.class);
}
/**
* @param model an RDF2Go model
* @return all instances of this class in Model 'model' as a ReactorResult,
* which can conveniently be converted to iterator, list or array.
*
* [Generated from RDFReactor template rule #class3-as]
*/
public static ReactorResult extends MusicToken> getAllInstances_as(Model model) {
return Base.getAllInstances_as(model, RDFS_CLASS, MusicToken.class );
}
/**
* Remove triple {@code (this, rdf:type, MusicToken)} from this instance. Other triples are not affected.
* To delete more, use deleteAllProperties
* @param model an RDF2Go model
* @param instanceResource an RDF2Go resource
*
* [Generated from RDFReactor template rule #class4]
*/
public static void deleteInstance(Model model, Resource instanceResource) {
Base.deleteInstance(model, RDFS_CLASS, instanceResource);
}
/**
* Delete all triples {@code (this, *, *)}, i.e. including {@code rdf:type}.
* @param model an RDF2Go model
* @param instanceResource an RDF2Go resource
*
* [Generated from RDFReactor template rule #class5]
*/
public static void deleteAllProperties(Model model, Resource instanceResource) {
Base.deleteAllProperties(model, instanceResource);
}
///////////////////////////////////////////////////////////////////
// property access methods
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy