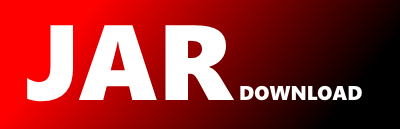
org.fryske_akademy.jsf.NewSupportingLazyController Maven / Gradle / Ivy
/*
* Copyright 2018 Fryske Akademy.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fryske_akademy.jsf;
import java.io.Serializable;
import org.fryske_akademy.jpa.EntityInterface;
import org.fryske_akademy.jsf.lazy.AbstractLazyModel;
import org.fryske_akademy.jsf.lazy.NewSupportingLazyModel;
import org.fryske_akademy.jsf.util.JsfUtil;
import org.primefaces.PrimeFaces;
/**
* Controller that supports new entities as a row in a datatable.
* @author eduard
*/
public abstract class NewSupportingLazyController> extends AbstractLazyController{
public NewSupportingLazyController(Class clazz, M lazyModel) {
super(clazz, lazyModel);
}
/**
* javascript to execute when new row is on last page in datatable, called from {@link #editNew(java.lang.String) }
* @param table
* @return
*/
protected abstract String newRowOnLastPage(String table);
/**
* javascript to execute when new row is on current page in datatable, called from {@link #editNew(java.lang.String) }
* @param table
* @return
*/
protected abstract String newRowOnCurrentPage(String table);
/**
* Calls {@link #prepareCreate() } and {@link #executOnClient(java.lang.String) }.
* @param table
* @throws InstantiationException
* @throws IllegalAccessException
*/
public void editNew(String table) throws InstantiationException, IllegalAccessException {
prepareCreate();
executOnClient(table);
}
/**
* Executes {@link #newRowOnCurrentPage(java.lang.String) } or
* {@link #newRowOnLastPage(java.lang.String) } on the client.
* @param table
*/
protected void executOnClient(String table) {
if (newOnLastPage()) {
PrimeFaces.current().executeScript(newRowOnLastPage(table));
} else {
PrimeFaces.current().executeScript(newRowOnCurrentPage(table));
}
}
/**
* Calls {@link #copy() } and {@link #executOnClient(java.lang.String) }.
* @param table
* @throws InstantiationException
* @throws IllegalAccessException
*/
public void copy(String table) throws InstantiationException, IllegalAccessException {
copy();
executOnClient(table);
}
/**
* returns true when {@link AbstractLazyModel#getPageSize() } <= {@link AbstractLazyModel#getWrappedData() wrapped datasize}.
* In this case the new entity, added in {@link NewSupportingLazyModel#setWrappedData(java.lang.Object) }, will land on the last page.
* @return
*/
protected boolean newOnLastPage() {
if (getLazyModel().getWrappedData() != null) {
if (getLazyModel().getPageSize() <= getLazyModel().getWrappedData().size()) {
// new entity lands on last page
return true;
}
}
return false;
}
/**
* calls the super if no newEntity is present
* @throws InstantiationException
* @throws IllegalAccessException
*/
@Override
public void prepareCreate() throws InstantiationException, IllegalAccessException {
if (getNewEntity() == null) {
super.prepareCreate();
}
}
/**
* return id or -1 for new entity, for the new entity the rowKey will be -1 which makes it visible in a datatable
*
* @param id
* @return
*/
public Serializable getRowKey(Serializable id) {
return id != null ? id : -1;
}
/**
* calls the super and {@link #setNewEntity(org.fryske_akademy.jpa.EntityInterface) } with null.
* @param e
* @return
* @throws Exception
*/
@Override
public E create(E e) throws Exception {
E create = super.create(e);
setNewEntity(null);
return create;
}
/**
* When the entity argument is transient call {@link #setNewEntity(org.fryske_akademy.jpa.EntityInterface) } with null
* otherwise call {@link #destroy(org.fryske_akademy.jpa.EntityInterface) } and {@link #setSelected(org.fryske_akademy.jpa.EntityInterface) }
* with null. Decrements rowCount in the lazymodel and removes the entity from the wrapped data
* @param entity
* @throws Exception
*/
public void remove(E entity) throws Exception {
if (entity.isTransient()) {
setNewEntity(null);
} else if (entity.equals(getSelected())) {
destroy(entity);
setSelected(null);
}
getLazyModel().setRowCount(getLazyModel().getRowCount()-1);
getLazyModel().getWrappedData().remove(entity);
}
/**
* return {@link #getNewEntity() } when it isn't null and {@link #getSelected() } is null, otherwise
* return {@link #getSelected() }.
* @return
*/
public E toDelete() {
return (getNewEntity() != null && getSelected() == null) ? getNewEntity()
: getSelected();
}
/**
* delete should be disabled when {@link #toDelete() } is null;
* @return
*/
public boolean disableDelete() {
return toDelete() == null;
}
/**
* show some usefull info to a user what is about to be deleted
* @return
*/
public String deleteTitle() {
return toDelete() == null ? "" : JsfUtil.getFromBundle(JsfUtil.getLocaleBundle(getBundleName()), "Delete") + " "
+ JsfUtil.getConverter(toDelete().getClass()).getAsString(null, null, toDelete()) + "?";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy